The watch
function in Vue.js enables you to monitor changes to a particular property or expression and execute some logic in response to those changes. It is frequently used to respond to data changes and update other Vue app's or component parts appropriately.
To understand the watch function properly, first create a variable username
and bind that to an input field. So that to change the value of the input field's text, username
will also update automatically.
create a variable:
data() {
return {
"username": ""
}
}
username bind with an input field:
<label>Username : </label>
<input type="text" v-model="username">
<p>Value of username : [[ username ]]</p>
Adding watch function:
Syntax:
watch: { variable_name(new_value, old_value) { // You can log data in the console } }
watch: {
username(new_value, old_value) {
console.log(`Old username ${old_value} and new username ${new_value}`)
}
}
Output:
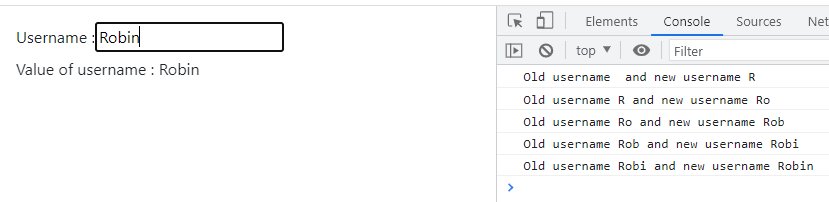
Full Code:
{% extends "base.html" %}
{% load static %}
{% block content %}
<style>
#vue_app {
margin: 1rem;
}
</style>
<div id="vue_app">
<label>Username : </label>
<input type="text" v-model="username">
<p>Value of username : [[ username ]]</p>
</div>
<script src="https://unpkg.com/vue@3"></script>
<script>
let app = Vue.createApp({
delimiters: ["[[", "]]"],
data() {
return {
"username": ""
}
},
watch: {
username(new_value, old_value) {
console.log(`Old username ${old_value} and new username ${new_value}`)
}
}
})
app.mount('#vue_app')
</script>
{% endblock content %}