In Vue.js, the lifecycle of a component or app refers to the different stages it goes through during its existence. These stages are define by various lifecycle hooks or functions, which are special methods that you can define within your Vue component to perform certain actions at specific times.
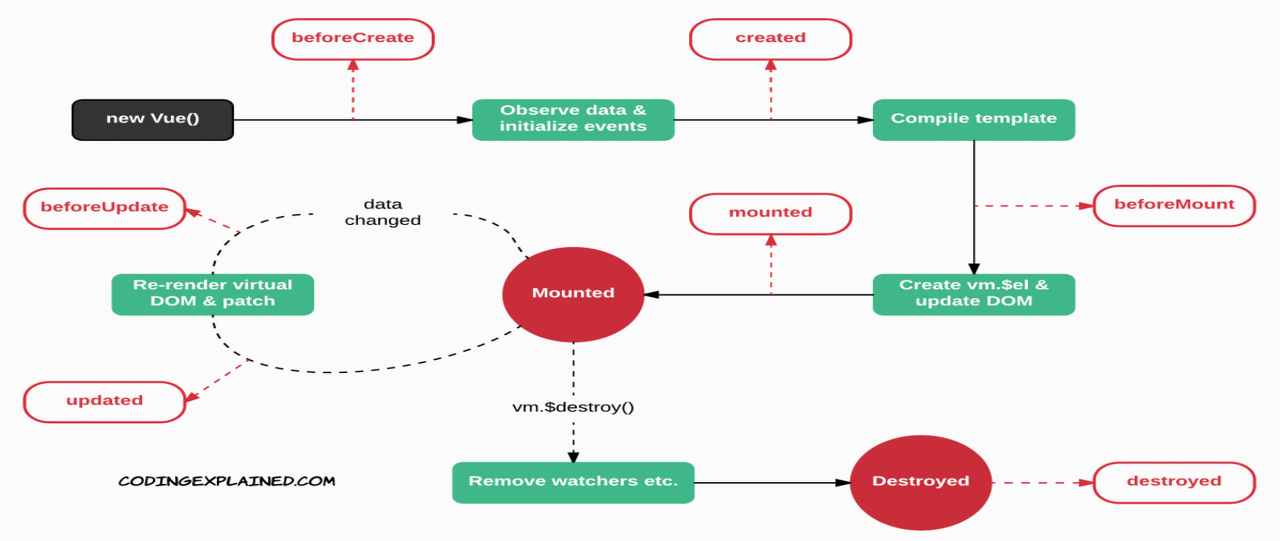
Lifecycle hooks or functions of Vue:
- beforeCreate: This hook is called before the component is created. At this point, data and events have not been initialized.
- created: This hook is called when the component has been created. Data observation, computed properties, and methods have been set up, but the component has not been inserted into the DOM yet.
- beforeMount: This hook is called before the component is inserted into the DOM. At this point, the template has been compiled, and the component is about to be rendered.
- mounted: This hook is called when the component has been inserted into the DOM. You can access the DOM elements and manipulate them in this hook. It is often used for initial setup that requires DOM interaction.
- beforeUpdate: This hook is called when the component is about to be updated, typically as a result of a data change. You can perform some actions before the component re-renders.
- updated: This hook is called after the component has been updated and re-rendered. You can access the updated DOM in this hook. Be cautious of infinite update loops when modifying data inside this hook.
- beforeUnmount: This hook is called before the component is unmounted and destroyed. You can perform cleanup tasks or cancel pending requests in this hook.
- unmounted: This hook is called after the component has been unmounted and destroyed. It is a good place to release any resources or event listeners associated with the component.
- errorCaptured: This hook is called when an error occurs within the component's descendant components. It allows you to capture and handle the error.
mounted of Vue:
mounted hook is used widely. Calling api, initial configurations, checking initial conditions etc are define here.
{% extends "base.html" %}
{% load static %}
{% block content %}
<div id="vue_app">
<h1>[[ message ]]</h1>
<p>[[ email ]]</p>
</div>
<script src="https://unpkg.com/vue@3"></script>
<script>
let app = Vue.createApp({
delimiters: ["[[", "]]"],
data() {
return {
"message" : "Vue is connected.",
"email" : "youremail@gmail.com"
}
},
mounted() {
console.log("calling mounted function")
}
})
app.mount('#vue_app')
</script>
{% endblock content %}
Output
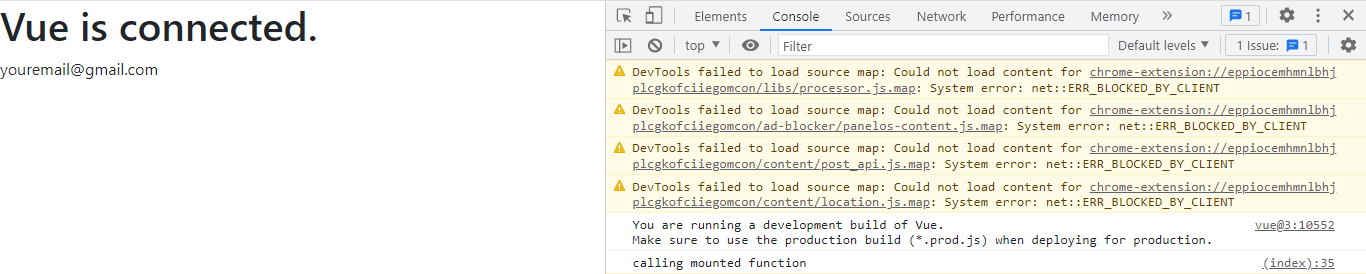