In Vue.js, the v-model
directive is a convenient way to create two-way data binding between form input elements and component data. It simplifies the process of synchronizing the data between the input and the component's data property.
Creating a vue variable:
data() {
return {
"username": ""
}
}
username
is a variable that will be bound to an input field. This binding will be a two-way binding. That means if you change the username value, it will automatically change the input field's value. Also, if you change the input field, it will update the username's current value.
Input Binding with v-model
:
<input type="text" v-model="username">
Display the value of the binding variable:
<p>Value of username: [[ username ]]</p>
Output:
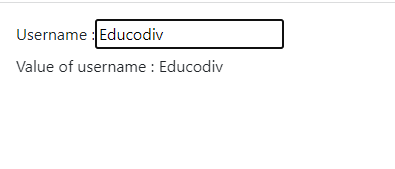
Full Code:
{% extends "base.html" %}
{% load static %}
{% block content %}
<style>
#vue_app {
margin: 1rem;
}
</style>
<div id="vue_app">
<label>Username : </label>
<input type="text" v-model="username">
<p>Value of username : [[ username ]]</p>
</div>
<script src="https://unpkg.com/vue@3"></script>
<script>
let app = Vue.createApp({
delimiters: ["[[", "]]"],
data() {
return {
"username": ""
}
}
})
app.mount('#vue_app')
</script>
{% endblock content %}