The methods
function is used to define methods for a Vue app or component. These functions can be used to handle events or listeners. Functions have access to the app's or component's data and can modify it. Functions can also access functions or computed properties.
Create vue function:
methods: {
update_email() {
console.log("calling update email function")
this.email = "updateyouremail@gmail.com"
}
}
Vue function use as click listener:
creating a button and click listener will use there:
<div id="vue_app">
<p>[[ email ]]</p>
<button>Click me</button>
</div>
Adding click listener on button:
<button @click="update_email">Click me</button>
Passing argument @click event:
methods: {
update_passing_email(email) {
console.log(`calling update_passing_email ${email}`)
this.email = email
}
}
<button @click="update_passing_email('newemail@gmail.com')">Update email</button>
Output:
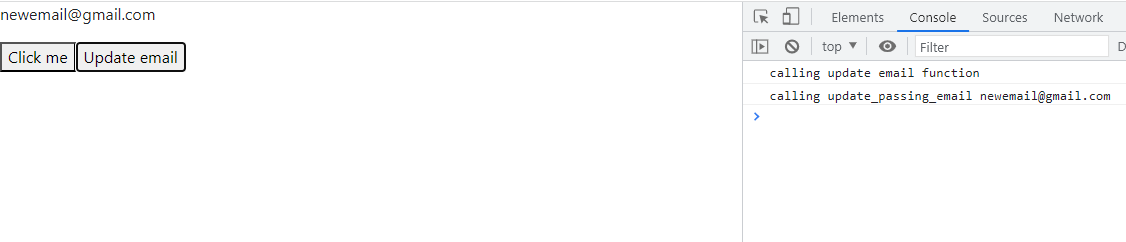
Full code:
{% extends "base.html" %}
{% load static %}
{% block content %}
<div id="vue_app">
<p>[[ email ]]</p>
<button @click="update_email">Click me</button>
<button @click="update_passing_email('newemail@gmail.com')">Update email</button>
</div>
<script src="https://unpkg.com/vue@3"></script>
<script>
let app = Vue.createApp({
delimiters: ["[[", "]]"],
data() {
return {
"email" : "youremail@gmail.com"
}
},
methods: {
update_email() {
console.log("calling update email function")
this.email = "updateyouremail@gmail.com"
},
update_passing_email(email) {
console.log(`calling update_passing_email ${email}`)
this.email = email
}
}
})
app.mount('#vue_app')
</script>
{% endblock content %}