The methods
function is used to define methods for a Vue app or component. These functions can be used to handle events or listeners. Functions have access to the app's or component's data and can modify it. Functions can also access functions or computed properties.
Create vue function:
Syntax:
methods: { name_of_the_function() { //function body } }
methods: {
setup_message() {
console.log("calling setup_message")
this.message = "Vue connected and update message"
}
}
Calling Vue function:
From mounted
hook, we can call function.
Syntax:
this.name_of_the_function()
this.setup_message()
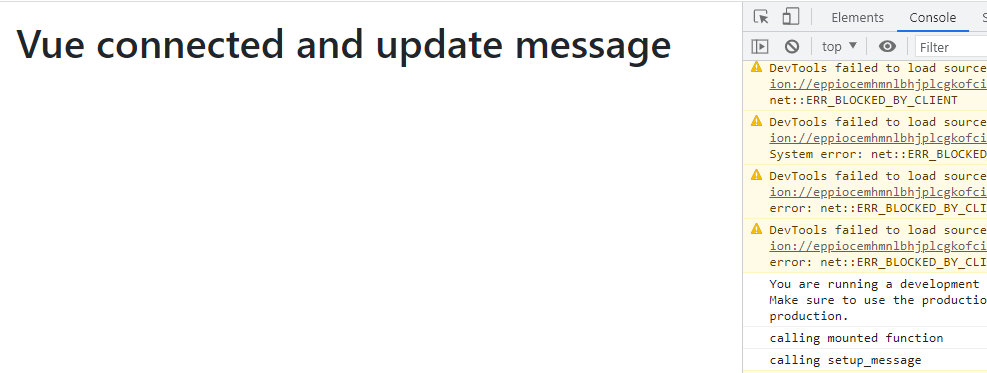
Full code:
{% extends "base.html" %}
{% load static %}
{% block content %}
<style>
#vue_app {
margin: 1rem;
}
</style>
<div id="vue_app">
<h1>[[ message ]]</h1>
</div>
<script src="https://unpkg.com/vue@3"></script>
<script>
let app = Vue.createApp({
delimiters: ["[[", "]]"],
data() {
return {
"message" : "Vue is connected.",
}
},
methods: {
setup_message() {
console.log("calling setup_message")
this.message = "Vue connected and update message"
}
},
mounted() {
console.log("calling mounted function")
this.setup_message()
}
})
app.mount('#vue_app')
</script>
{% endblock content %}