Django is python base web development framework use for large scale, multi page and highly secure application. This course base on django and vue. We use vue framework inside django template without npm. So first step is to create djagno project. Step-by-step guides are below:
Pre Requirements:
Python should be install in your device.
Creating a folder for this project. In that folder open your command prompt or terminal.
Creating virtual environment:
A Python virtual environment is a self-contained directory that contains a specific version of Python and its installed packages. It allows you to create an isolated environment for your Python projects, where you can install and manage dependencies separately from your system-wide Python installation.
virtualenv venv
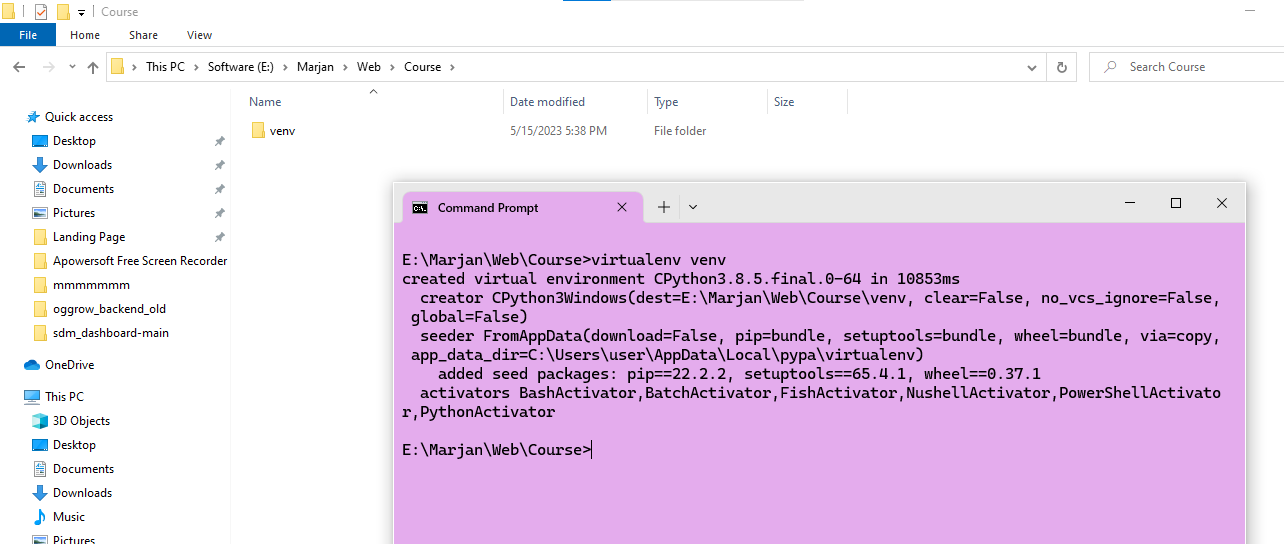
Install Django using pip:
pip install django
Creating django project:
django-admin startproject vue_in_django
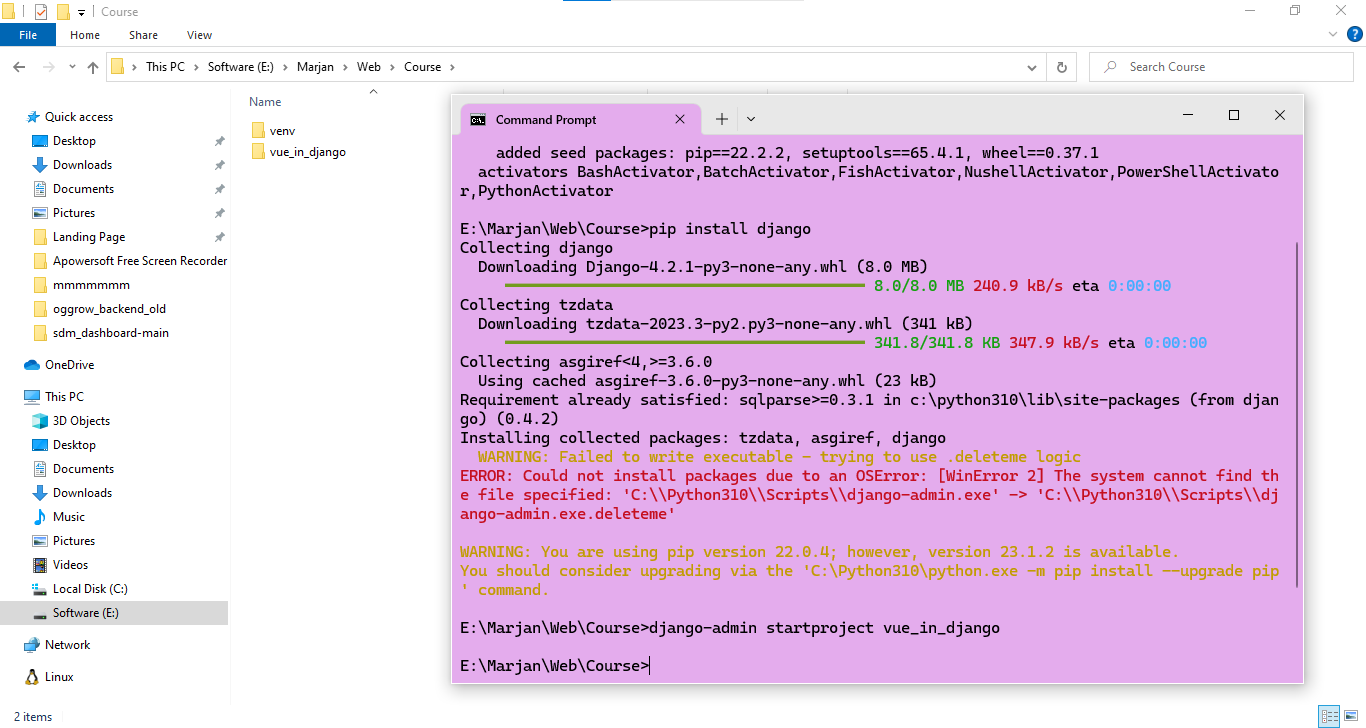
For this course, I'm using VS code text editor. So open the project in VS code editor.
cd vue_in_django
code .
Creating a new app:
django-admin startapp blog
Register app in settings.py:
# Application definition
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
# register your apps
"blog",
]
Creating a folder in root project directory:
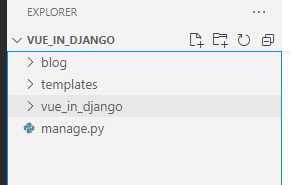
Adding template folder with django:
import os
TEMPLATES = [
{
"BACKEND": "django.template.backends.django.DjangoTemplates",
"DIRS": [os.path.join(BASE_DIR, "templates")],
"APP_DIRS": True,
"OPTIONS": {
"context_processors": [
"django.template.context_processors.debug",
"django.template.context_processors.request",
"django.contrib.auth.context_processors.auth",
"django.contrib.messages.context_processors.messages",
],
},
},
]
Config static and media folder:
STATIC_URL = "/static/"
MEDIA_URL = "/media/"
STATIC_ROOT = os.path.join(BASE_DIR, "static")
MEDIA_ROOT = os.path.join(BASE_DIR, "media")
Setup urls.py with blog/urls.py:
Creating urls.py file in blog folder and add basic code of url handling:
from django.urls import path, re_path
app_name = "blog"
urlpatterns = []
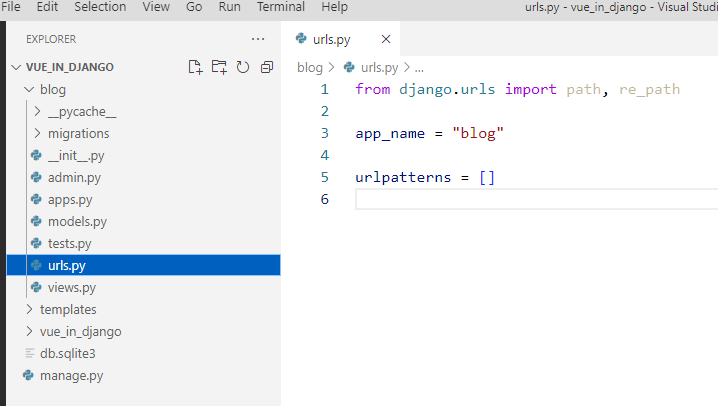
Register blog/urls.py with vue_in_django/urls.py:
from django.contrib import admin
from django.urls import path, include
from django.conf.urls.static import static
from django.conf import settings
urlpatterns = [
path("admin/", admin.site.urls),
path("blog/", include("blog.urls", "blog")),
]
if settings.DEBUG:
urlpatterns += static(settings.STATIC_URL, document_root=settings.STATIC_ROOT)
urlpatterns += static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
Creating a base.html file in templates/base.html:
Here, I'm using bootstrap starter template. And this base will use everywhere.
<!doctype html>
<html lang="en">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<!-- Bootstrap CSS -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.4.1/dist/css/bootstrap.min.css"
integrity="sha384-Vkoo8x4CGsO3+Hhxv8T/Q5PaXtkKtu6ug5TOeNV6gBiFeWPGFN9MuhOf23Q9Ifjh" crossorigin="anonymous">
<title>Vue in django template</title>
</head>
<body>
{% block content %}
{% endblock content %}
<!-- Optional JavaScript -->
<!-- jQuery first, then Popper.js, then Bootstrap JS -->
<script src="https://code.jquery.com/jquery-3.4.1.slim.min.js"
integrity="sha384-J6qa4849blE2+poT4WnyKhv5vZF5SrPo0iEjwBvKU7imGFAV0wwj1yYfoRSJoZ+n" crossorigin="anonymous">
</script>
<script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.0/dist/umd/popper.min.js"
integrity="sha384-Q6E9RHvbIyZFJoft+2mJbHaEWldlvI9IOYy5n3zV9zzTtmI3UksdQRVvoxMfooAo" crossorigin="anonymous">
</script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@4.4.1/dist/js/bootstrap.min.js"
integrity="sha384-wfSDF2E50Y2D1uUdj0O3uMBJnjuUD4Ih7YwaYd1iqfktj0Uod8GCExl3Og8ifwB6" crossorigin="anonymous">
</script>
</body>
</html>
Creating home.html file in blog/template/home.html:
{% extends "base.html" %}
{% load static %}
{% block content %}
<div>
<p>We are learning Vue in django template from Educodiv</p>
</div>
{% endblock content %}
Creating a django view in views.py:
from django.shortcuts import render
def home_view(request):
context = {}
return render(request, "home.html", context)
This time migration database before run the server:
python manage.py makemigrations
python manage.py migrate
Django runserver:
python manage.py runserver
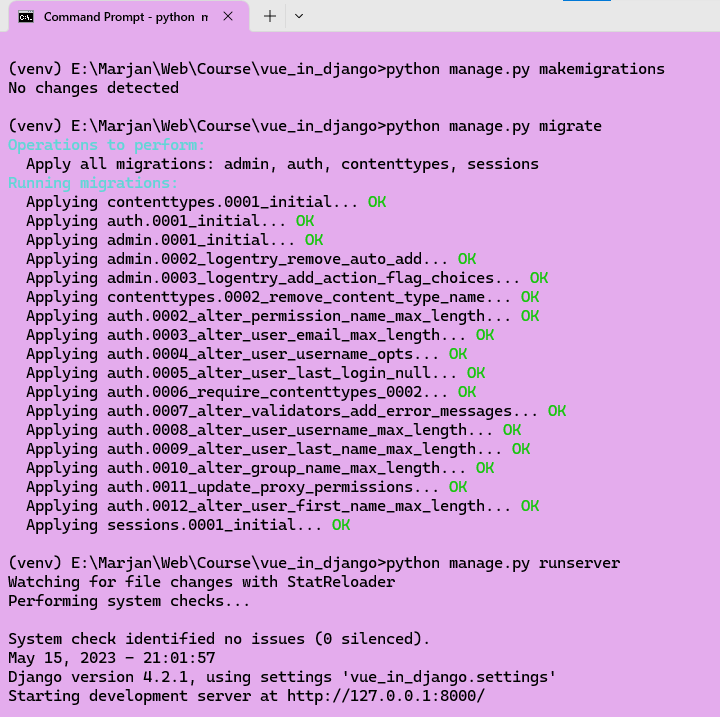
Output:
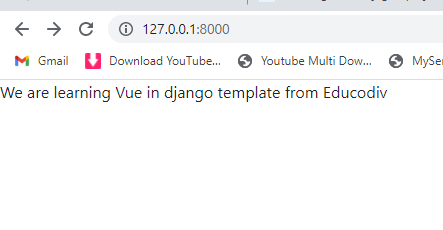