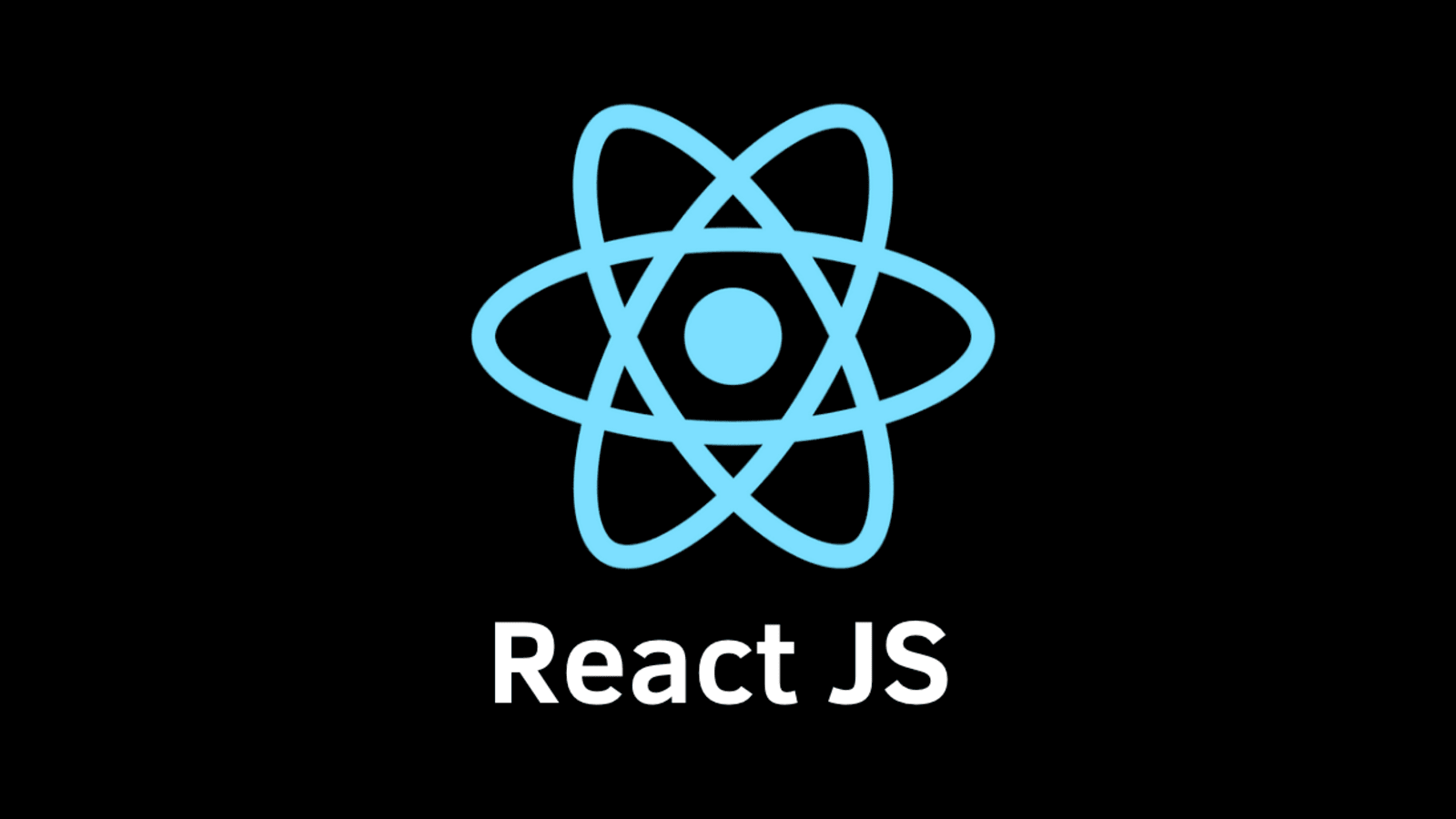
Theming and customization are essential aspects of creating visually appealing and unique user interfaces in React.js applications. While CSS frameworks and component libraries offer a great starting point, customizing these UI components to match your project's specific design and branding requirements is crucial. In this blog post, we'll explore how to theme and customize UI components in React.js, providing detailed explanations and examples to help you achieve a personalized and cohesive user interface.
Understanding Theming and Customization:
Theming involves defining a consistent set of visual styles, such as colors, typography, spacing, and other design elements, that apply across your entire application. Customization, on the other hand, allows you to modify individual components or create new ones with unique styles to suit specific use cases.
Advantages:
- Consistent Design Language: By creating a theme with a predefined set of styles and design elements, theming ensures consistency throughout the application. Consistency in design enhances the user experience and gives the application a polished and professional look. Users can easily navigate the interface, as they become familiar with the design patterns and elements.
- Branding and Identity: Theming allows you to tailor the UI to match the branding and identity of the application or organization. With customized colors, typography, and other visual elements, you can create a distinct and recognizable look that aligns with your brand's personality.
- Ease of Maintenance: Using a theme to manage global styles simplifies the maintenance process. When you need to make design changes, you can update the theme, and the changes will propagate throughout the application. This approach reduces the need to modify styles in individual components, making it more efficient and reducing the risk of introducing inconsistencies.
- Efficient Development Workflow: Having a centralized theme simplifies the development process, especially when multiple developers are working on the project. Developers can refer to the theme file to find the design guidelines and apply the styles consistently, streamlining the workflow and reducing the chances of conflicting styles.
- Accessibility and User Experience: Theming can promote accessibility in applications. By defining accessible color combinations and using appropriate font sizes, contrast ratios, and spacing, you create a user-friendly experience for all users, including those with disabilities. Accessibility features contribute to better user engagement and inclusivity.
- Rapid Prototyping: With a theme in place, developers can quickly prototype new components or screens with consistent styles. This expedites the design iteration process and allows stakeholders to visualize the application's appearance early in the development cycle.
- Flexible Customization: Customizing individual components using CSS-in-JS libraries like styled-components, emotion, or styled-jsx allows developers to override the global theme styles when necessary. This flexibility ensures that specific components can have unique styles while maintaining the overall theme and design consistency.
- Modularity and Reusability: Creating a theme and applying it using a ThemeProvider promotes modularity and reusability in React components. Customizing and reusing components becomes easier, leading to a more maintainable and scalable codebase.
- Optimized Performance: Theming and customizing UI components can lead to optimized performance. By managing styles in a theme, you can eliminate unnecessary CSS duplication and reduce the overall size of the CSS, resulting in faster loading times for your application.
- Better Collaboration with Design Teams: Having a well-defined theme aids in collaboration with design teams. Designers can work with developers using the same design system, ensuring that the implemented UI aligns with the intended visual design.
Designing the Theme:
Create a file called `theme.js` or `variables.scss`, depending on the technology you are using for styling (JavaScript or Sass). In this file, define the global styles and variables that will be used across the application. For example:
// theme.js
const theme = {
primaryColor: '#007bff',
secondaryColor: '#6c757d',
fontFamily: 'Arial, sans-serif',
fontSize: '16px',
};
export default theme;
Creating a Theme Provider:
ThemeProvider is a concept commonly used in React applications, especially when working with CSS-in-JS libraries, to provide a centralized theme throughout the component tree. It allows developers to define a set of global styles, variables, and design properties that can be accessed by any component within the application. This centralization simplifies theming and customization, making it easier to maintain consistent styles and apply a specific visual theme to the entire UI.
How ThemeProvider Works: ThemeProvider is implemented using the Context API in React. The Context API enables data to be passed down the component tree without the need to manually pass props at each level. This means that any component within the ThemeProvider can access the theme data without explicitly receiving it as a prop.
// ThemeContext.js
import { createContext, useContext } from 'react';
const ThemeContext = createContext();
export const useTheme = () => useContext(ThemeContext);
export default ThemeContext;
// ThemeProvider.jsx
import React from 'react';
import ThemeContext from './ThemeContext';
import theme from './theme';
const ThemeProvider = ({ children }) => {
return (
<ThemeContext.Provider value={theme}>
{children}
</ThemeContext.Provider>
);
};
export default ThemeProvider;
Applying the Theme:
In your main application file, wrap the entire application with the `ThemeProvider` to make the theme accessible throughout the component tree.
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import ThemeProvider from './ThemeProvider';
ReactDOM.render(
<React.StrictMode>
<ThemeProvider>
<App />
</ThemeProvider>
</React.StrictMode>,
document.getElementById('root')
);
Using the Theme in Components:
Now that the theme is available, you can access it in any component within the `ThemeProvider` using the `ThemeContext` and apply the styles accordingly.
// ThemeComponent.jsx
import React, { useContext } from 'react'
import ThemeContext from '../theme/ThemeContext';
const ThemeComponent = () => {
const theme = useContext(ThemeContext);
return (
<>
<h1>Theme component</h1>
<div style={{ backgroundColor: theme.primaryColor, fontFamily: theme.fontFamily, fontSize: theme.fontSize }}>
Customized Component using Theme
</div>
</>
);
}
export default ThemeComponent;
Customizing UI Components:
To customize existing UI components, use CSS-in-JS solutions like `styled-components`, `emotion`, or `styled-jsx`. These libraries allow you to style components directly using the theme and custom CSS styles.
// StyledComponent.js
import styled from 'styled-components';
const StyledComponent = styled.div`
background-color: ${props => props.theme.primaryColor};
font-family: ${props => props.theme.fontFamily};
font-size: ${props => props.theme.fontSize};
padding: 10px;
color: white;
`;
Best Practices:
- Separate Theme and Component Logic: Keep the theme-related logic separate from the component logic. Create a dedicated theme.js file or directory to store theme-related variables, colors, and styles. Isolating the theme logic makes it easier to manage and update styles consistently throughout the application.
- Use a Theme Provider: Implement a ThemeProvider component to provide the theme data to all components that need access to it. This centralizes the theme data and allows easy consumption of the theme throughout the component tree.
- Create a Theme Interface: Define a theme interface or type for the theme object to ensure that all theme-related properties are correctly defined and used consistently across the application.
- Use CSS-in-JS Libraries: Consider using CSS-in-JS libraries like styled-components, emotion, or styled-jsx for customizing components. These libraries offer a seamless integration of CSS styles with React components and provide flexibility in creating custom styles using the theme variables.
- Use Custom Props: Use custom props to allow easy customization of components based on the theme. For example, pass color, size, or font options as props to enable variations in components without modifying the global theme.
- Limit Global Overrides: Avoid overriding the global theme styles excessively at the component level. It is preferable to use the theme styles and custom props whenever possible to maintain consistency across the application.
- Define Reusable Components: Create reusable UI components with customizable styles. Encapsulate the customization logic within the component itself, making it easier to reuse the component with different styles and themes.
- Test Thoroughly: Test the customized components across various use cases and screen sizes to ensure that the customization does not lead to layout issues or conflicts with other components in the application.
- Document the Theme: Properly document the theme and its variables, explaining their purpose and usage. This documentation will be helpful for developers who work on the project in the future, ensuring a smoother onboarding process.
- Consider Accessibility: When customizing components, pay attention to accessibility concerns. Ensure that color choices, font sizes, and spacing comply with accessibility standards to create an inclusive and user-friendly application.
- Avoid Excessive Nesting: Avoid excessive nesting of styled components, as it can lead to increased specificity and make the styles harder to maintain. Keep styles as flat as possible and utilize compound components for complex components.
- Performance Considerations: Keep performance in mind when customizing theme components. Avoid using heavy animations or complex styles that could impact the application's performance negatively.
- Keep the Theme Lightweight: While theming is powerful, keep the theme file lightweight and focused on defining essential design elements. Avoid adding unnecessary styles that might not be used in the application.
Theming and customizing UI components in React.js is a powerful way to create visually consistent and unique user interfaces. By setting up a theme and applying it through a `ThemeProvider`, you can customize the global styles of your application easily. Additionally, using CSS-in-JS libraries enables you to customize individual components further, achieving a cohesive and personalized look. With these techniques, you can create stunning and user-friendly React.js applications that match your project's design requirements perfectly.