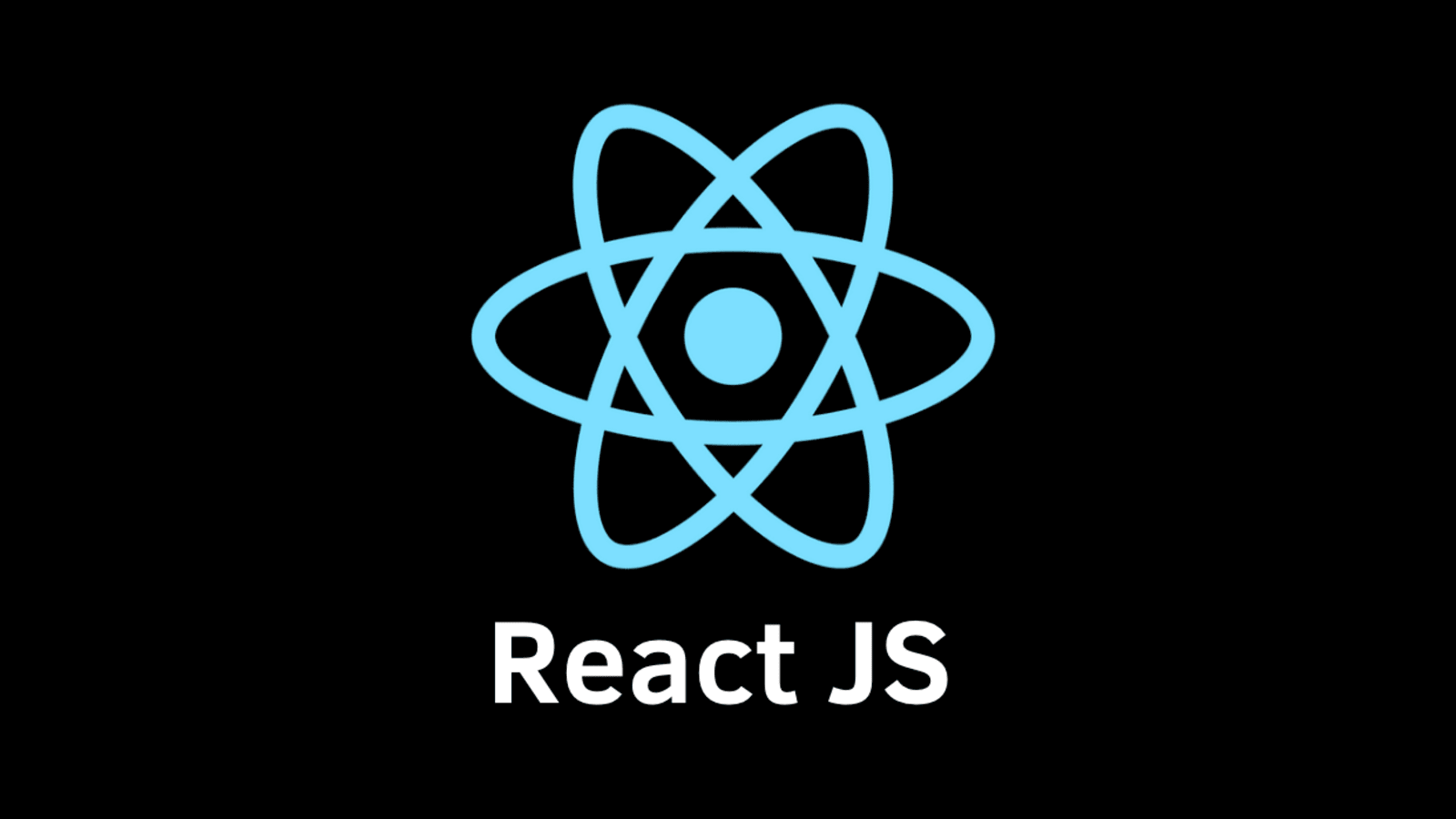
Styling plays a crucial role in crafting visually appealing and user-friendly React applications. As a React developer, you have multiple options for styling your components, each with its own set of advantages and considerations. In this comprehensive tutorial, we will explore three popular styling options in React: CSS, CSS-in-JS, and component libraries. We will delve into each approach, provide code examples, and explain the pros and cons of each method.
Styling options in React have evolved significantly over the years, and developers now have various techniques to choose from. The traditional approach is to use CSS, either through inline styles or external CSS files. More recently, CSS-in-JS libraries have gained popularity, offering a dynamic and powerful way to style components directly within the JavaScript code. Additionally, component libraries provide pre-designed UI components and styles, simplifying the styling process. Let's dive into each of these options and understand their use cases, advantages, and drawbacks.
CSS:
a. Inline CSS:
Inline CSS involves adding style attributes directly to JSX elements. While it offers quick styling, it can lead to a cluttered codebase and reduced maintainability. Let's look at an example:
const MyComponent = () => {
return <h1 style={{ color: 'blue', fontSize: '24px' }}>Hello, Inline CSS!</h1>;
};
In React, inline styles can be applied directly to JSX elements using the style attribute. The style attribute accepts a JavaScript object where the keys represent CSS property names, and the values represent their corresponding values.
Advantages:
- Quick and Simple: Inline styles allow you to apply styles directly to JSX elements using the style attribute, making it a fast and easy way to style individual elements.
- Dynamic Styling: Since inline styles are written in JavaScript, you can easily make them dynamic by using variables, props, or state values, allowing for reactive UI updates.
- Scoped Styles: Inline styles are scoped to the specific component they are applied to, preventing style conflicts across the application.
b. External CSS Files:
Using external CSS files is a traditional approach, where styles are defined in separate CSS files and imported into components. This method helps maintain a separation of concerns but can result in global style conflicts. Example:
/* styles.css */
.heading {
color: blue;
font-size: 24px;
}
/* MyComponent.jsx */
import React from 'react';
import './styles.css';
const MyComponent = () => {
return <h1 className="heading">Hello, External CSS!</h1>;
};
The styles.css file contains CSS rules that define styles for specific class selectors.
In the MyComponent.jsx file, we import the styles.css file using a relative path ./styles.css. This allows us to access the styles defined in styles.css within the scope of MyComponent.
Advantages:
- Separation of Concerns: External stylesheets provide a clear separation between styling and component logic, making the codebase more organized and easier to maintain.
- Global Styles: External stylesheets allow you to define global styles that can be applied consistently across the entire application, ensuring a cohesive look and feel.
- Caching and Performance: External stylesheets can be cached by the browser, leading to improved performance for subsequent page loads.
c. CSS Modules:
CSS Modules provide a solution to the global style conflicts of external CSS files. Each CSS file is treated as a local module, and the classes are scoped to the component. Example:
/* styles.module.css */
.heading { color: blue; font-size: 24px; }
/* MyComponent.jsx */
import React from 'react';
import styles from './styles.module.css';
const MyComponent = () => {
return <h1 className={styles.heading}>Hello, CSS Modules!</h1>;
};
The styles.module.css file is a CSS Module, and it contains CSS rules that define styles for specific class selectors.
Inside the MyComponent.jsx file, we import the styles.module.css file using a relative path ./styles.module.css. By doing this, we can access the styles defined in styles.module.css within the scope of MyComponent.
Advantages:
- Local Scoping: CSS Modules offer local scoping, which means styles defined in one component do not affect other components. This reduces the likelihood of unintended style conflicts.
- Reusable Classes: CSS Modules generate unique class names for each component, allowing you to reuse the same class names without worrying about naming collisions.
- Maintainable and Modular: With CSS Modules, you can encapsulate styles within the component, leading to a more maintainable and modular codebase.
CSS-in-JS:
a. Styled Components:
Styled Components is a popular CSS-in-JS library that allows you to write CSS directly within your JavaScript code. It provides component-level styling with automatic scoping.
import React from 'react';
import styled from 'styled-components';
const Heading = styled.h1`
color: blue;
font-size: 24px;
`;
const MyComponent = () => {
return <Heading>Hello, Styled Components!</Heading>;
};
b. Emotion:
Emotion is another CSS-in-JS library with similar functionality to Styled Components. It emphasizes performance and provides a powerful set of features for styling React components.
import React from 'react';
import { css } from '@emotion/react';
const MyComponent = () => {
return (
<h1
css={css`
color: blue;
font-size: 24px;
`}
>
Hello, Emotion!
</h1>
);
};
Advantages:
- Scoped Styles: CSS-in-JS libraries like Styled Components and Emotion provide scoped styles, ensuring that styles are encapsulated within the component, reducing the risk of global style conflicts.
- Dynamic Styling: With CSS-in-JS, you can easily use JavaScript variables, props, or theme providers to create dynamic and responsive styles, enabling a more interactive user experience.
- Component Reusability: Styles are closely tied to components, making it easier to reuse and share components with their associated styles across the application.
- Themability: CSS-in-JS allows you to set up theming and easily switch between different themes without changing the underlying component logic.
- Better Developer Experience: CSS-in-JS brings CSS and JavaScript together, allowing developers to write styles directly within their component codebase, leading to a more streamlined development process.
Component Libraries:
a. Material-UI:
Material-UI is a widely used React component library that follows the Material Design guidelines. It offers a rich set of pre-designed components and styles, making it easy to create beautiful and consistent UIs.
npm install @mui/material @emotion/react @emotion/styled
import React from 'react';
import Button from '@mui/material/Button';
const MyComponent = () => {
return <Button variant="contained">Hello, Material-UI!</Button>;
};
b. React Bootstrap:
React Bootstrap is an adaptation of the popular Bootstrap framework for React. It provides a comprehensive set of components and styles for building responsive web applications.
npm install react-bootstrap bootstrap
import React from 'react';
import Button from 'react-bootstrap/Button';
const MyComponent = () => {
return <Button variant="primary">Hello, React Bootstrap!</Button>;
};
Advantages:
- Ready-to-Use Components: Component libraries like Material-UI and React Bootstrap provide a wide range of pre-designed and tested UI components, saving development time and effort.
- Consistent Design: Component libraries adhere to design guidelines and offer a cohesive set of components, ensuring a consistent and professional look and feel for the entire application.
- Responsive and Mobile-Friendly: Component libraries are designed to be responsive out of the box, ensuring that the UI adapts well to various screen sizes and devices.
- Reduced Learning Curve: By using component libraries, developers can take advantage of existing components and styles, reducing the need to write custom CSS or styles from scratch.
- Community and Support: Popular component libraries have active communities, frequent updates, and extensive documentation, providing support and resolving issues more efficiently.
Comparison and Use Cases:
a. Performance:
- CSS: Lower performance impact but can lead to global style conflicts.
- CSS-in-JS: Slightly higher performance impact due to style injection, but offers better scoping and dynamic styling.
- Component Libraries: Higher performance impact due to importing external styles but provides consistent and well-optimized components.
b. Code Maintainability:
- CSS: Limited scope isolation and potential naming conflicts.
- CSS-in-JS: Better code organization with component-level styling and dynamic theming.
- Component Libraries: Consistent and standardized components lead to easier maintenance.
c. Theming and Customization:
- CSS: Limited theming support, requires manual customization for each component.
- CSS-in-JS: Supports dynamic theming and easy customization using props or theme providers.
- Component Libraries: Themed by default and allow customization through theming providers.
d. Developer Experience:
- CSS: Familiar and easy to use, especially for small projects.
- CSS-in-JS: Requires learning a new syntax, but offers a powerful and dynamic styling approach.
- Component Libraries: Easy to use, reduces development time, and promotes consistent design.
Best Practices and Recommendations:
- Mixing Styling Approaches: You can use a combination of styling approaches based on the specific needs of your project. For instance, CSS-in-JS or component libraries for complex components, and traditional CSS for basic styling.
- Consistent Styling: Maintain consistency in your styling approach throughout the project to avoid confusion and improve code readability.
- Managing Global Styles: When using global styles, consider using CSS Modules or CSS-in-JS libraries like styled-components to scope the styles locally.
- Project-Specific Considerations: Evaluate your project's requirements, team expertise, and performance considerations before choosing a styling approach.
In this tutorial, we explored various styling options available in React, including CSS, CSS-in-JS, and component libraries like Material-UI and React Bootstrap. Each approach has its own set of advantages and considerations, and the choice depends on the specific needs of your project. Whether you prefer the familiarity of CSS, the flexibility of CSS-in-JS, or the convenience of component libraries, React offers numerous options to style your components effectively. Consider the performance, code maintainability, theming requirements, and developer experience when making your decision. By understanding the strengths and weaknesses of each approach, you can make informed choices and create stunning and responsive user interfaces for your React applications.