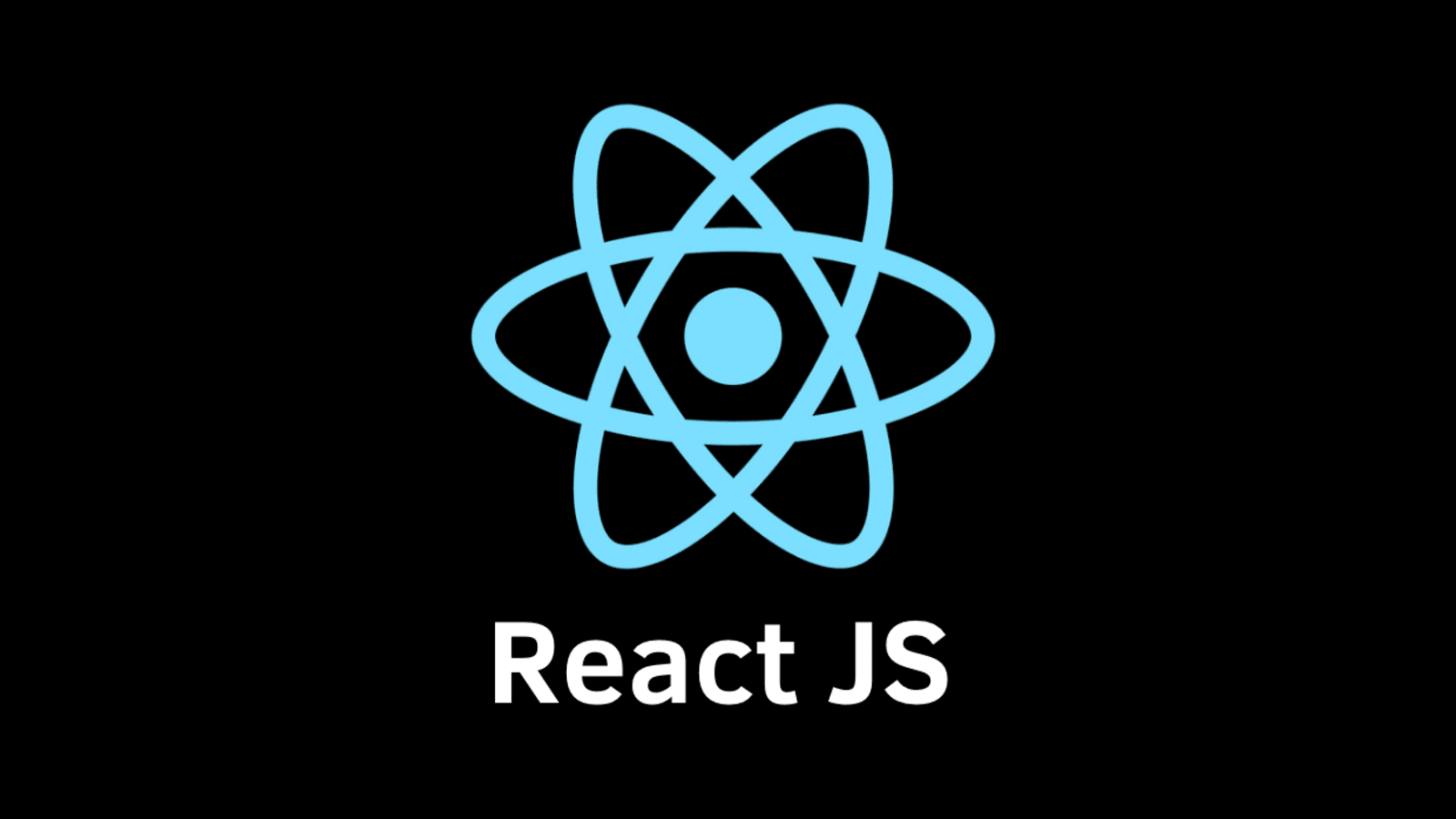
State management is a fundamental aspect of building modern React applications. As applications grow in complexity, managing state using simple `useState` hooks may become cumbersome and lead to code duplication. This is where `useReducer` comes to the rescue. The `useReducer` hook is a powerful alternative to `useState` that offers a more structured approach to state management in React.
In this tutorial, we will explore the `useReducer` hook, understand its purpose, and learn how to use it effectively to manage state in complex React applications.
What is `useReducer`?
`useReducer` is one of the built-in hooks provided by React. It is primarily used for managing state in situations where state transitions are complex and closely related or when the state logic is better expressed in a reducer-style approach, similar to how Redux manages state. The hook is inspired by the `reducer` function in Redux and helps to simplify the management of complex state updates.
The `useReducer` hook takes in two arguments: a `reducer` function and an `initialState` value. The `reducer` function receives the current state and an `action` object as arguments, and it returns the updated state based on the action type. The `initialState` value represents the initial state of the component.
How `useReducer` Works?
Let's dive into the inner workings of `useReducer`:
Reducer Function
The reducer function is the core of `useReducer`. It takes the current state and an action object as input and returns the updated state. The reducer function is defined as follows:
const reducer = (state, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
};
In this example, the reducer handles two types of actions: `'INCREMENT'` and `'DECREMENT'`. Depending on the action type, it returns an updated state with the `count` value incremented or decremented.
Initializing the `useReducer` Hook
To use the `useReducer` hook, you need to call it within your functional component, passing the `reducer` function and the `initialState` as arguments:
import React, { useReducer } from 'react';
const initialState = { count: 0 };
const Counter = () => {
const [state, dispatch] = useReducer(reducer, initialState);
// Rest of the component code
};
In this example, we initialize the `useReducer` hook with the `reducer` function we defined earlier and set the initial state of the component to `{ count: 0 }`.
Dispatching Actions
To update the state, you use the `dispatch` function returned by the `useReducer` hook. Dispatching an action means sending an object with a `type` property that matches one of the cases in the `reducer` function:
const handleIncrement = () => {
dispatch({ type: 'INCREMENT' });
};
const handleDecrement = () => {
dispatch({ type: 'DECREMENT' });
};
When you call `dispatch({ type: 'INCREMENT' })`, the `reducer` function will be executed with the current state and the action object `{ type: 'INCREMENT' }`, which increments the `count` value.
Benefits of `useReducer`
The `useReducer` hook provides several benefits over the traditional `useState` approach:
- Centralized Logic: With `useReducer`, you can centralize complex state transitions and actions within the reducer function. This results in a more organized and maintainable codebase, especially as your application grows.
- Scalability: As your application's state management requirements become more intricate, `useReducer` scales better than `useState`. It helps you avoid prop drilling and keeps your codebase cleaner.
- Readability: By following a reducer-style pattern, the code becomes more predictable and easier to read. Each action type is handled explicitly within the reducer, which aids in understanding how state changes occur.
When to Use `useReducer`
While `useReducer` offers various advantages, it may not always be the best choice for every situation. Consider using `useReducer` when:
- Complex State Transitions: Your state changes are interdependent or require complex logic to update.
- Multiple Actions: You have multiple actions that affect the same state, and you want to handle them centrally.
- Predictable State Updates: You want your state updates to be more predictable and maintainable.
- Integration with Libraries: You are integrating your application with libraries like Redux or other state management tools that use a similar reducer pattern.
For simpler state management requirements, the `useState` hook might be sufficient, as it requires less boilerplate code.
Using `useReducer` with Complex State
One of the most significant benefits of `useReducer` is its ability to handle complex state objects. Instead of managing individual state variables with `useState`, you can manage the entire state as an object within the reducer function.
Let's consider an example of managing a more complex state with `useReducer`:
const initialState = {
username: '',
email: '',
age: 0,
isSubscribed: false,
};
const reducer = (state, action) => {
switch (action.type) {
case 'SET_USERNAME':
return { ...state, username: action.payload };
case 'SET_EMAIL':
return { ...state, email: action.payload };
case 'SET_AGE':
return { ...state, age: action.payload };
case 'TOGGLE_SUBSCRIPTION':
return { ...state, isSubscribed: !state.isSubscribed };
default:
return state;
}
};
In this example, we have a state object with properties `username`, `email`, `age`, and `isSubscribed`. The reducer function handles different actions to update these properties.
Combining `useReducer` with `useContext`
Combining `useReducer` with `useContext` can be a powerful way to manage state across different components in your application. `useContext` provides a way to pass the state and dispatch function to components without prop drilling.
To achieve this, you can define a context and use `useReducer` within the context provider:
const StateContext = React.createContext();
const StateProvider = ({ children }) => {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<StateContext.Provider value={{ state, dispatch }}>
{children}
</StateContext.Provider>
);
};
With this setup, you can access the state and dispatch function from any child component within the `StateProvider`.
Performance Considerations
While `useReducer` is an excellent choice for state management, it's important to consider performance implications, especially for components with frequent re-renders. For some applications, the overhead of using `useReducer` for simple state may not be worth the benefits.
For highly dynamic and frequently updating state, consider using more specialized state management libraries like Redux, Zustand, or Recoil. These libraries are optimized for performance and provide additional features like memoization, time-travel debugging, and more.
Best Practices and Tips
Here are some best practices and tips to keep in mind when using `useReducer`:
- Follow the Single Source of Truth: Keep your state logic within the reducer function and avoid duplicating state management across multiple components.
- Compose Reducers: If your state becomes too complex, consider splitting the reducer into smaller reducers and compose them using the `useReducer` hook.
- Immutability Matters: Always return a new state object from the reducer to ensure immutability.
- Keep Actions Descriptive: Use descriptive action types to help you understand the state changes at a glance.
- Avoid Side Effects: Try to avoid side effects within the reducer function. For side effects, use the `useEffect` hook or other appropriate mechanisms.
- Write Unit Tests: Write unit tests for your reducer functions to ensure they behave as expected.
In this tutorial, we explored the `useReducer` hook in React, its purpose, and how it works. We learned how `useReducer` can help manage complex state in a structured and organized manner. By centralizing state transitions and actions within the reducer function, `useReducer` offers a cleaner and more scalable approach to state management.
While `useReducer` is powerful, it's essential to consider your application's specific state management needs. For simple state, the `useState` hook may be more suitable, while for complex scenarios, `useReducer` can provide significant benefits.
In conclusion, by understanding and effectively using `useReducer`, you can build maintainable and performant React applications with advanced state management capabilities. Happy coding!