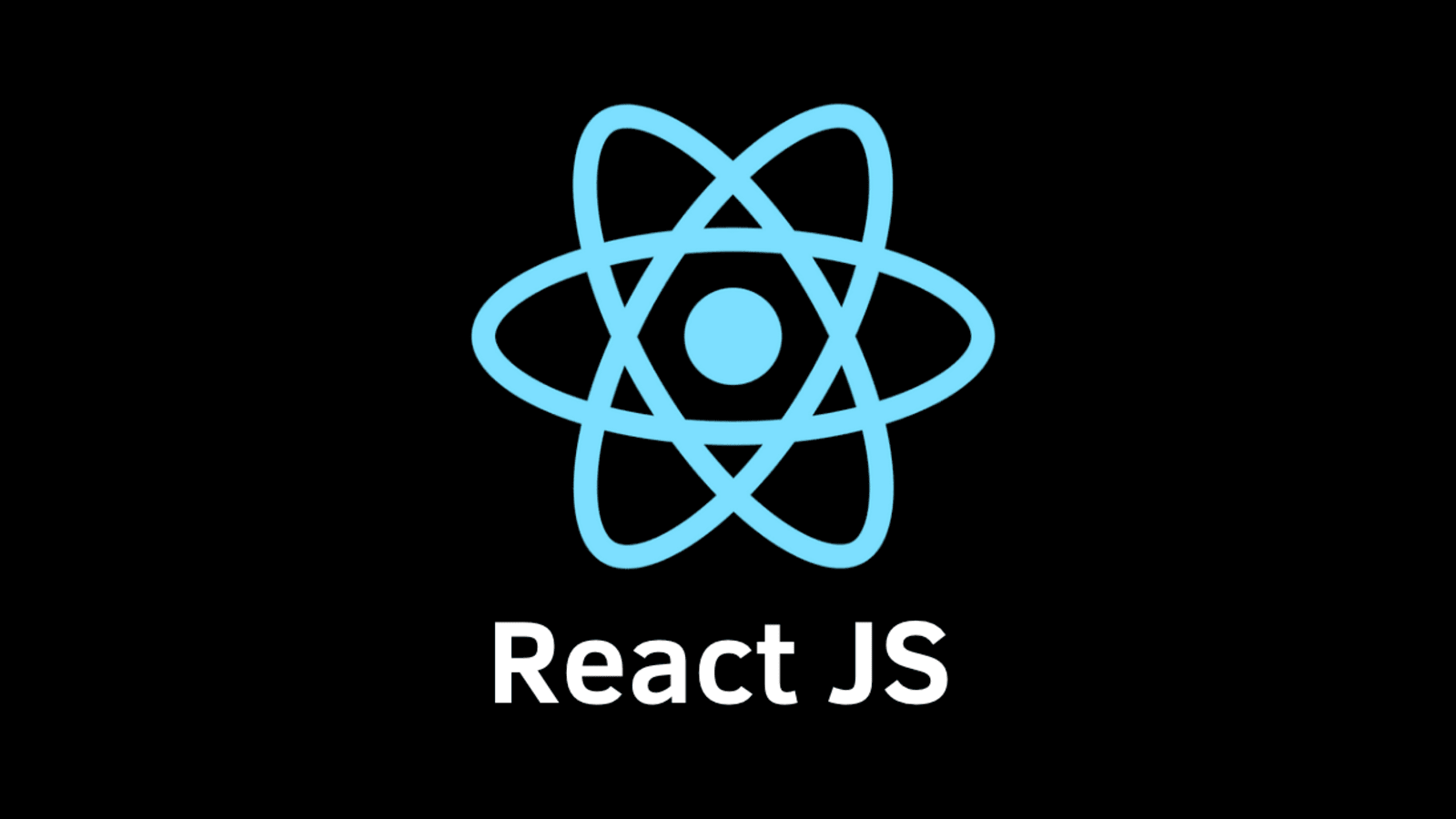
Route parameters in React Router allow you to capture dynamic values from the URL and use them within your components. This powerful feature enables you to create dynamic and data-driven web applications with clean and maintainable code. In this tutorial, we'll dive deep into route parameters in React Router and explore how to use them effectively to enhance your application's functionality.
Introduction to Route parameters
A route parameter, also known as a URL parameter, is a dynamic value that can be extracted from the URL of a web application. In the context of React Router, route parameters are used to capture specific segments of a URL and pass them as variables to components for further processing.
Route parameters are enclosed within colons in the route's path definition. For example, in the route path /user/:username, :username is a route parameter that can capture different usernames from the URL.
Here's a breakdown of how route parameters work:
- Defining Route Parameters: When defining a route using React Router, you can include route parameters in the route's path definition. These parameters are indicated by a colon followed by a parameter name. For example: /user/:username.
- Capturing Values: When a user navigates to a URL that matches a route with parameters, React Router captures the value of the parameter from the URL segment. For instance, if the user visits /user/john, the value john is captured from the username parameter.
- Passing to Components: The captured parameter value is then passed to the associated component as a prop. This allows the component to access and use the dynamic value in its rendering and functionality.
Route parameters are extremely useful for creating dynamic and personalized user experiences. They allow you to create reusable components that can display content or perform actions based on the dynamic values extracted from the URL. For example, in a blogging application, you could use route parameters to display individual blog posts by capturing the post's unique identifier from the URL.
Here's a quick example of how route parameters can be used:
<Route path="/user/:username" component={UserProfile} />
In this example, the UserProfile component can access the captured username parameter as a prop and use it to fetch user-specific data from a backend server or database.
Route parameters are a fundamental feature in building dynamic and data-driven web applications, allowing you to create flexible and interactive user interfaces.
Advantages:
- Dynamic Content: Route parameters allow you to dynamically change the content of a page based on the value in the URL. This enables you to display personalized data or content to users.
- Cleaner URLs: Route parameters help in creating cleaner and more user-friendly URLs by embedding relevant information directly in the URL structure.
- Bookmarked URLs: Users can bookmark or share URLs with route parameters, ensuring that when they revisit the link, they see the same content or data.
- SEO Optimization: Search engines can crawl and index individual pages with different route parameters, enhancing the discoverability of specific content on your website.
- User Engagement: By showing personalized content or data using route parameters, you can enhance user engagement and keep users interested in your application.
- Modularity: Route parameters allow you to create modular components that can be reused across different URLs with varying parameter values.
Use Cases:
- User Profiles: Capture a user's username from the URL and display their profile information, posts, or activities on a dedicated profile page.
- Product Pages: Use route parameters to display detailed information about a specific product based on its unique identifier.
- Blog Posts: Create dynamic routes for individual blog posts, allowing users to access and read specific posts by their unique identifiers.
- Category Pages: Build category-specific pages that display products, articles, or content related to a particular category captured from the URL.
- Search Results: Implement route parameters to display search results based on user queries, allowing users to bookmark or share specific search results.
- User Dashboards: Use route parameters to create dynamic user dashboards that display different information based on the user's role or preferences.
- Pagination: Implement pagination with route parameters to display different pages of content, such as paginated lists of blog posts or products.
- Language Selection: Capture a language code from the URL to dynamically change the language of your application's content.
- Location-Based Content: Show location-specific content by capturing location data from the URL, allowing users to see relevant information based on their region.
- Survey or Form Responses: Use route parameters to capture response IDs or tokens and display survey or form responses for users to review.
Example:
Step 1: Create Components
In this example, we'll create two components: Home and UserProfile. The UserProfile component will display user profiles using data from route parameters.
Create a new file named Home.js inside the src folder:
// Home.js
import React from 'react';
const Home = () => {
return <h1>Welcome to the Home Page</h1>;
};
export default Home;
Create a new file named UserProfile.js inside the src folder:
// UserProfile.js
import React from 'react';
import { useParams } from 'react-router-dom';
const UserProfile = () => {
const { username } = useParams();
return <h1>User Profile: {username}</h1>;
};
export default UserProfile;
Step 2: Set Up App Routing
Now, let's configure the routing in the App.js file using React Router DOM and implement route parameters.
Open the src/App.js file and replace its contents with the following code:
// App.js
import React from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
import Home from './Home';
import UserProfile from './UserProfile';
const App = () => {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/user/:username" component={UserProfile} />
</Switch>
</Router>
);
};
export default App;
Using Route Parameters
In this example, we're using the Route component with the path prop containing the route parameter (:username). This parameter will capture the dynamic value from the URL and make it available to the UserProfile component.
When a user visits /user/john, the UserProfile component will display "User Profile: john".
When a user visits /user/susan, the component will display "User Profile: susan".
Best Practices:
Using route parameters effectively is crucial for maintaining a well-structured and user-friendly web application. Here are some best practices to follow when working with route parameters in React Router:
- Choose Descriptive Parameter Names: Select meaningful and descriptive names for your route parameters. This makes your code more understandable and helps other developers quickly grasp the purpose of each parameter.
- Validate and Sanitize Parameters: Ensure that the values captured by route parameters are valid and properly sanitized before using them. This prevents unexpected behavior and security vulnerabilities.
- Document Your Route Parameters: Document the purpose and format of each route parameter in your codebase's documentation. This documentation will be invaluable for both you and your team members.
- Use Route Configuration: Instead of defining routes inline, consider using a route configuration object that centralizes all your route definitions. This makes it easier to manage and update routes as your application grows.
- Avoid Overusing Route Parameters: While route parameters are powerful, avoid using them excessively within a single route. Overusing route parameters can make your URLs complex and less user-friendly.
- Consider Optional Parameters: Use optional route parameters when appropriate. This can help maintain a cleaner URL structure and allow users to omit certain parameters without causing errors.
- Avoid Redundant Parameters: Avoid capturing parameters that can be derived from other captured parameters. Redundant parameters can complicate your code and URLs.
- Handle Nonexistent Routes: Implement a "404 Not Found" route to handle cases where users navigate to a route that doesn't exist. This improves the user experience by displaying a meaningful error page.
- Test Edge Cases: Test your route parameters with various inputs, including edge cases, to ensure your application behaves as expected. Use unit tests and end-to-end tests to cover different scenarios.
- Use Prop Validation: If you're passing route parameters as props to components, use prop validation (using tools like PropTypes or TypeScript) to ensure that the received data is of the expected type.
- Code Splitting: Consider implementing code splitting for components that are only used on specific routes. This improves performance by loading only the necessary code for each route.
- Use Route Guards: Implement route guards or authentication checks to ensure that users have the appropriate permissions to access routes with sensitive data.
- Optimize SEO: If SEO is a concern, ensure that search engines can crawl and index the content displayed using route parameters. Consider using server-side rendering (SSR) for improved SEO.
- Keep URLs Intuitive: Strive to create URLs that are intuitive and readable. Users should be able to understand the purpose of a route by looking at its URL.
Route parameters in React Router allow you to create dynamic and data-driven components by capturing values from the URL. This feature is invaluable when building applications that require personalized content or user-specific data. By following the steps outlined in this tutorial, you've learned how to implement route parameters in your React application. Feel free to experiment with different parameter names and integrate route parameters into your own projects to create more dynamic and engaging user experiences.