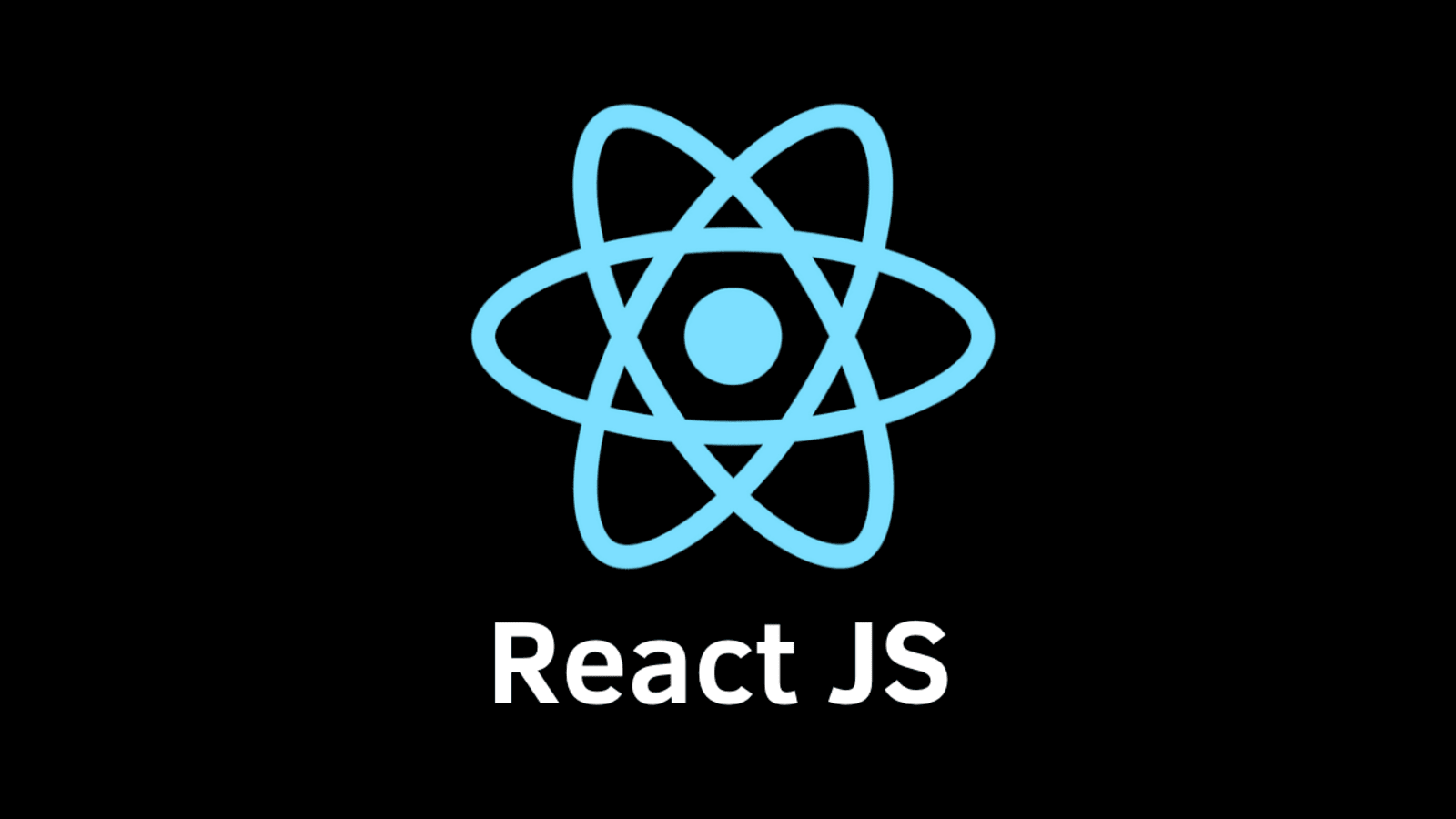
Simplify State Management with Redux Toolkit in React
Managing state in a React application can be complex, especially as the project grows. Redux, a popular state management library, has been a go-to solution for many developers. However, Redux setup and boilerplate code can be overwhelming. Enter Redux Toolkit, a powerful and streamlined library designed to make Redux development more efficient and developer-friendly.
In this tutorial, we'll dive into Redux Toolkit, exploring its features, benefits, and how to use it to simplify state management in your React applications.
Introduction to Redux Toolkit
Redux Toolkit is an official package provided by the Redux team that simplifies and streamlines the process of working with Redux in a React application. It aims to address common challenges and pain points that developers often encounter when setting up and managing Redux state. Redux Toolkit offers a set of utilities, abstractions, and best practices that make Redux development more efficient, less error-prone, and overall more enjoyable.
Redux Toolkit is not a replacement for Redux itself; rather, it's a set of tools built on top of Redux that encourages best practices and helps you write cleaner, more maintainable, and more performant Redux code.
Core Features of Redux Toolkit
- Slice: Slices are a key feature of Redux Toolkit that encapsulate a portion of your Redux state, including its reducer and actions. They significantly reduce the amount of code required to create reducers and action creators.
- Immutability Helpers: Redux Toolkit uses Immer under the hood, allowing you to write reducers that look like they're mutating state directly. Immer ensures that these changes are applied immutably, simplifying your code and improving readability.
- Redux DevTools Integration: Redux Toolkit seamlessly integrates with the Redux DevTools extension, providing powerful debugging and time-traveling capabilities to help you track and understand state changes.
- Thunk Middleware: Thunk middleware comes pre-configured in Redux Toolkit, allowing you to write asynchronous actions with ease. Thunks enable you to handle complex asynchronous operations while keeping your code organized.
Advantages:
- Streamlined Code: Redux Toolkit significantly reduces the amount of boilerplate code required to set up and manage a Redux store. This results in cleaner and more focused code that's easier to read, write, and maintain.
- Slices for Modular Code: Redux Toolkit introduces the concept of slices, which allow you to organize your reducers, actions, and initial state in a more modular way. Slices encapsulate related logic, making it simpler to manage different parts of your state.
- Integrated Immer for Immutability: Redux Toolkit integrates Immer, a library that simplifies working with immutable data. With Immer, you can write reducer logic that appears to directly modify state while ensuring immutability behind the scenes.
- Simplified Async Actions with Thunks: Redux Toolkit comes with the thunk middleware pre-configured. Thunks provide an organized and efficient way to handle asynchronous actions, such as API requests, while keeping your action creators clean.
- Redux DevTools Integration: Redux Toolkit seamlessly integrates with the Redux DevTools browser extension. This integration allows you to monitor and debug state changes, dispatched actions, and even time-travel through state history for debugging purposes.
- Optimized Performance with Memoization: Redux Toolkit employs memoization techniques that prevent unnecessary component re-renders when the state hasn't changed. This leads to improved performance and smoother user experiences.
- Consistent Conventions: Redux Toolkit encourages best practices and provides a consistent structure for managing state. This leads to more standardized code across your application, making it easier for developers to understand and collaborate on.
- Faster Development Process: With Redux Toolkit, you can set up a fully functional Redux store with significantly less code compared to traditional Redux. This speeds up the development process and allows you to focus more on building features.
- Ease of Migration: If you're already using Redux in your application, Redux Toolkit provides clear migration paths to transition your existing codebase to its simplified structure. This makes it easier to adopt Redux Toolkit gradually.
- Officially Maintained and Supported: Redux Toolkit is an official package maintained by the Redux team. This ensures that it's up-to-date, well-documented, and follows best practices for Redux development.
- Familiarity: Redux Toolkit retains the core principles of Redux while simplifying the process. If you're already familiar with Redux, adopting Redux Toolkit won't require learning an entirely new approach.
- Enhanced Collaboration: The standardized structure and conventions of Redux Toolkit make it easier for developers to collaborate on projects. The reduced complexity also lowers the barrier for new team members to understand and contribute.
- Built-in Testing Utilities: Redux Toolkit includes built-in testing utilities that make it easier to write unit tests for your Redux logic. This promotes better code quality and maintainability.
Installing Redux Toolkit
To get started with Redux Toolkit, install the package using npm or yarn:
npm install @reduxjs/toolkit
Getting Started with Slices
Slices are at the core of Redux Toolkit. They define portions of your state, reducers, and action creators in a concise manner. Let's create a slice for a counter feature:
import { createSlice } from '@reduxjs/toolkit';
const counterSlice = createSlice({
name: 'counter',
initialState: { value: 0 },
reducers: {
increment: (state) => {
state.value += 1;
},
decrement: (state) => {
state.value -= 1;
},
},
});
export const { increment, decrement } = counterSlice.actions;
export default counterSlice.reducer;
Creating a Redux Store with Redux Toolkit
With Redux Toolkit, creating a Redux store is a breeze:
import { configureStore } from '@reduxjs/toolkit';
import counterReducer from './counterSlice';
const store = configureStore({
reducer: {
counter: counterReducer,
},
});
export default store;
Dispatching Actions with Redux Toolkit
Dispatching actions in Redux Toolkit is straightforward:
import { useDispatch } from 'react-redux';
import { increment, decrement } from './counterSlice';
const CounterComponent = () => {
const dispatch = useDispatch();
return (
<div>
<button onClick={() => dispatch(increment())}>Increment</button>
<button onClick={() => dispatch(decrement())}>Decrement</button>
</div>
);
};
Asynchronous Data Fetching with Thunks
Asynchronous data fetching is a common requirement in modern web applications, especially when dealing with APIs and external data sources. Thunks are a concept within Redux Toolkit that provide a clean and organized way to handle asynchronous actions, such as making API calls, in your Redux-based application. Thunks allow you to perform complex asynchronous operations while maintaining the predictability and structure of your Redux actions and reducers.
Before diving into thunks, it's important to understand what middleware is in the context of Redux. Middleware are functions that intercept actions before they reach the reducers. They can be used to add custom logic, handle side effects, and modify actions before they update the state.
Thunk middleware is a specific type of middleware that enables you to dispatch functions (thunks) instead of plain action objects. Thunks are functions that can contain asynchronous operations and dispatch multiple actions as needed.
Redux Toolkit's integrated thunk middleware makes handling async actions clean and organized:
import { createAsyncThunk, createSlice } from '@reduxjs/toolkit';
const fetchUserData = createAsyncThunk('user/fetch', async (userId) => {
const response = await fetch(`/api/users/${userId}`);
const data = await response.json();
return data;
});
const userSlice = createSlice({
name: 'user',
initialState: { data: {}, status: 'idle' },
reducers: {},
extraReducers: (builder) => {
builder
.addCase(fetchUserData.pending, (state) => {
state.status = 'loading';
})
.addCase(fetchUserData.fulfilled, (state, action) => {
state.status = 'succeeded';
state.data = action.payload;
})
.addCase(fetchUserData.rejected, (state) => {
state.status = 'failed';
});
},
});
Simplified Immutability with Immer
Immer is a powerful JavaScript library that simplifies the process of working with immutable data structures. It allows you to write code that appears to be directly mutating state, while it ensures that the changes are applied immutably. Immer is widely used in state management libraries like Redux Toolkit to simplify reducer logic and improve code readability.
In the context of Redux Toolkit, Immer is used to streamline the process of updating the state within reducers. Let's explore how Immer simplifies immutability in Redux Toolkit:
Immer takes a different approach by allowing you to write code that looks like you're modifying the state directly, even though you're actually creating a new copy of the state under the hood. This makes the code more concise and easier to understand.
In the context of Redux Toolkit, you can use Immer to simplify reducer logic and eliminate the need for manual copying and spreading of state.
Redux Toolkit's createSlice function integrates Immer automatically when you define reducer functions. This means you can write reducer logic that appears to mutate the state directly, and Immer ensures that the updates are applied immutably.
Here's an example of a reducer using Immer within a Redux Toolkit slice:
const counterSlice = createSlice({
name: 'counter',
initialState: { value: 0 },
reducers: {
increment: (state) => {
state.value += 1;
},
},
});
Redux Toolkit is a game-changer in the Redux ecosystem. With its simplified syntax, integrated tools, and best practices, it offers a robust solution for state management in React applications. By adopting Redux Toolkit, you can build scalable and maintainable applications while enjoying a more efficient and developer-friendly experience. Give it a try and supercharge your Redux development today!