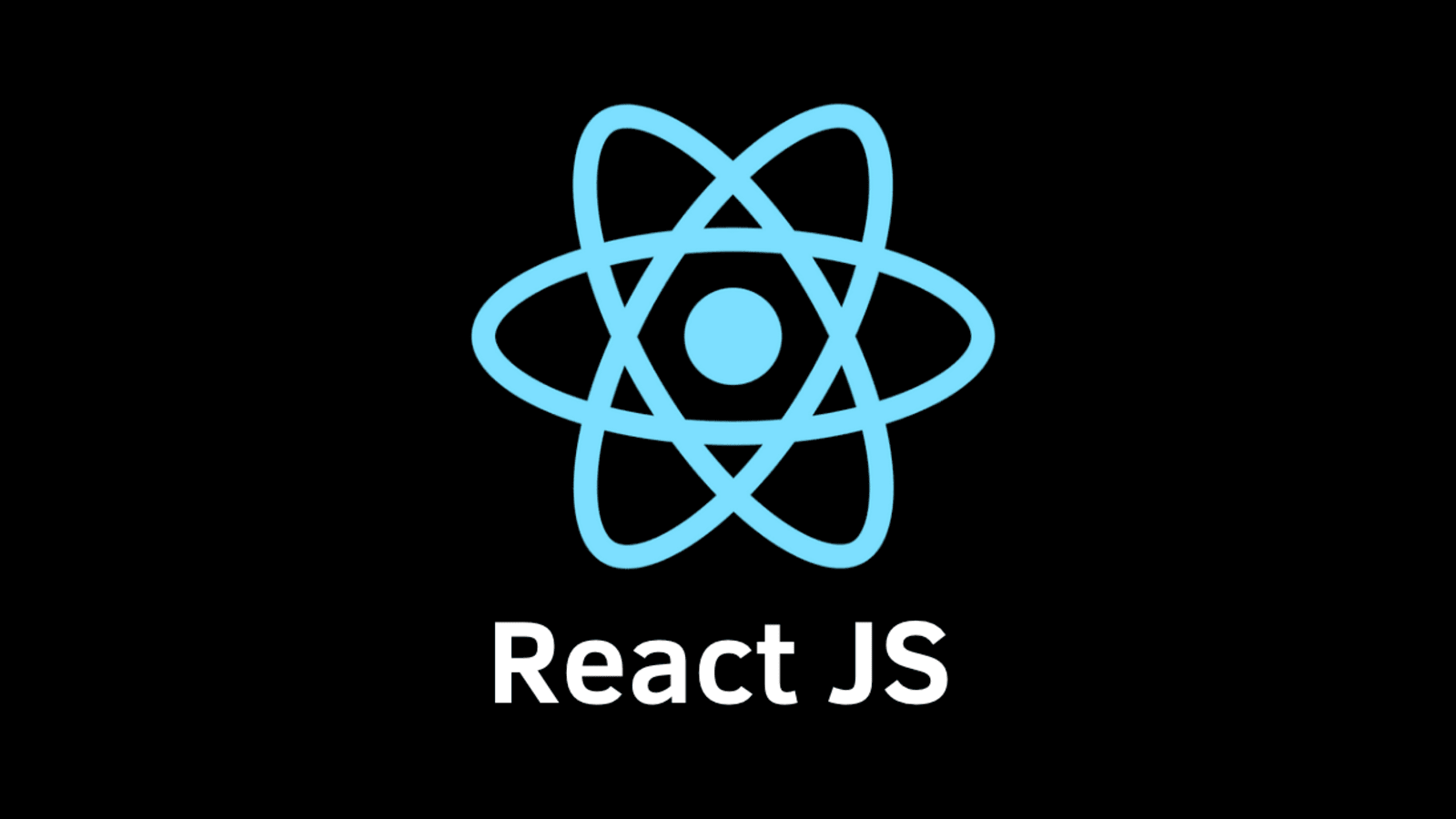
Conditional rendering is a powerful technique in React.js that allows you to display different components or elements based on specific conditions. It enables you to create dynamic user interfaces that adapt and respond to changing data or user interactions. In this blog post, we will delve into the concept of conditional rendering in React.js and provide an in-depth guide to help you leverage this technique effectively.
Understanding Conditional Rendering in React.js
Conditional rendering is the process of selectively rendering components or elements based on certain conditions. It is a fundamental aspect of building interactive and responsive user interfaces. In React.js, conditional rendering allows you to dynamically control what is rendered based on the state, props, or other variables in your components. Conditional rendering is of utmost importance in React.js as it enables developers to create dynamic, responsive, and interactive user interfaces. Here are some key reasons why conditional rendering is crucial:
1. User Experience: Conditional rendering allows developers to tailor the UI based on specific conditions or user interactions. By selectively showing or hiding components, elements, or content, you can provide a personalized and intuitive user experience. For example, you can display error messages when form validation fails or show loading spinners while waiting for data to load.
2. Data-driven Rendering: React.js is commonly used for data-driven applications. Conditional rendering allows you to display components based on the available data. For instance, you can render a list of items only if the data array is not empty, or display different components based on the state of an API request. This flexibility empowers you to build UIs that adapt to varying data conditions.
3. Simplified Component Structure: Conditional rendering helps maintain a clean component structure by avoiding unnecessary rendering of components when certain conditions are not met. It allows you to reduce the complexity of your codebase by showing only the necessary components or elements at any given time. This improves code readability, maintainability, and reusability.
4. Performance Optimization: By conditionally rendering components, you can optimize the performance of your React application. Unnecessary rendering can cause performance bottlenecks, especially when dealing with large or frequently updated data. With conditional rendering, you can minimize unnecessary re-renders and improve overall application performance.
5. Responsiveness Across Devices: In today's multi-device landscape, responsive design is paramount. Conditional rendering enables you to adjust the UI based on the device's screen size or capabilities. You can hide or rearrange components for mobile devices or show different layouts for desktop and tablet users. This ensures a seamless and optimized experience across various devices.
6. Enhanced Code Maintainability: Conditional rendering allows you to organize your code more efficiently by separating concerns based on specific conditions. By encapsulating condition-based rendering logic within components or functions, you can easily manage and update the UI based on changing requirements. This improves code maintainability and makes it easier to add new features or make modifications in the future.
7. Accessibility Considerations: Conditional rendering plays a crucial role in creating accessible web applications. By conditionally showing or hiding elements, you can optimize the experience for users who rely on assistive technologies. For example, you can present different versions of content for screen readers or adapt the UI for users with specific accessibility requirements.
Conditional rendering is a fundamental concept in React.js that allows developers to create dynamic and adaptive user interfaces. It enhances user experience, improves performance, simplifies component structure, and enables responsive design. By leveraging conditional rendering effectively, you can build engaging and user-friendly applications that cater to various data conditions and user interactions.
Using Conditional Statements for Rendering
React.js provides several approaches for conditional rendering. Let's explore the commonly used techniques:
- if Statements:
export const ConditionalRenderingIf = () => {
const condition=false;
if (condition) {
return <h1>Show Component A</h1>;
} else {
return <h1>Show Component B</h1>;
}
}
In this example, the Component function has a variable condition. If the `condition` evaluates to true, it renders "Show Component A"; otherwise, it renders "Show Component B". By using if statements, you can have more complex conditions and perform different rendering logic.
- Ternary Operator:
export const ConditionalRenderingTernary = () => {
const condition=true;
return (
<div>
{condition ? <h1>Show Component A</h1> : <h1>Show Component B</h1>}
</div>
);
}
The ternary operator provides a concise way to conditionally render components. In this example, the condition variable determines whether to render "Show Component A" or "Show Component B". If the `condition` is true, the first expression is rendered; otherwise, the second expression is rendered.
- Logical && Operator:
export const ConditionalRenderingLogical = () => {
const condition=true;
return (
<div>
{condition && <h1>Showing Component With Logical conditional rendering</h1>}
</div>
);
}
The logical && operator allows you to conditionally render components without using an else block. In this example, if the `condition` variable is true, it renders "Show Component A". If the `condition` is false, nothing is rendered. This technique is useful for rendering components based on a single condition.
- Conditional Rendering with React Hooks:
With React Hooks, such as `useState` and `useEffect`, you can manage state and control the rendering of components based on specific conditions or user interactions. Hooks provide a powerful way to handle conditional rendering in functional components.
export const ConditionalRenderingInlineFunc = () => {
const [todos, setTodos] = useState([
{ id: 1, text: 'Learn React', completed: true },
{ id: 2, text: 'Build a project', completed: false },
{ id: 3, text: 'Deploy application', completed: false },
]);
const toggleTodo = (id) => {
setTodos((prevTodos) =>
prevTodos.map((todo) =>
todo.id === id ? { ...todo, completed: !todo.completed } : todo
)
);
};
return (
<div>
<h1>Todo List</h1>
{todos.map((todo) => (
<div key={todo.id}>
<input
type="checkbox"
checked={todo.completed}
onChange={() => toggleTodo(todo.id)}
/>
<span
style={{
textDecoration: todo.completed ? 'line-through' : 'none',
}}
>
{todo.text}
</span>
</div>
))}
</div>
);
}
In this example, we have a todo list where each todo item is represented as an object in the todos array. Each object has properties like id, text, and completed, indicating whether the todo item is completed or not.
The toggleTodo function is responsible for toggling the completion status of a todo item. It uses the map method to iterate over the todos array and update the completed property of the corresponding todo item based on its id.
Within the return statement, we use the map method as an inline function to conditionally render todo items. For each todo object, we render a checkbox and the todo text. The checked attribute of the checkbox is set based on the completed property, and the text is styled with a line-through if the item is completed.
By using the map method as an inline function, we can dynamically render todo items based on the data in the todos array and update their completion status when the checkbox is clicked.
Best Practices for Conditional Rendering
When implementing conditional rendering, it's important to follow best practices to ensure clean and maintainable code. Here are some tips:
- Avoiding Excessive Nesting:
Avoid excessive nesting of conditional statements and components. Instead, try to refactor and extract components to keep your code clean and readable.
- Extracting Components for Reusability:
If you have reusable components within conditional rendering, consider extracting them into separate components. This improves code reusability and simplifies the rendering logic.
- Leveraging CSS Classes for Styling:
Conditional rendering can sometimes result in complex component structures. To maintain clean and manageable code, utilize CSS classes to apply different styles based on conditions. This separation of concerns improves code maintainability and enhances styling