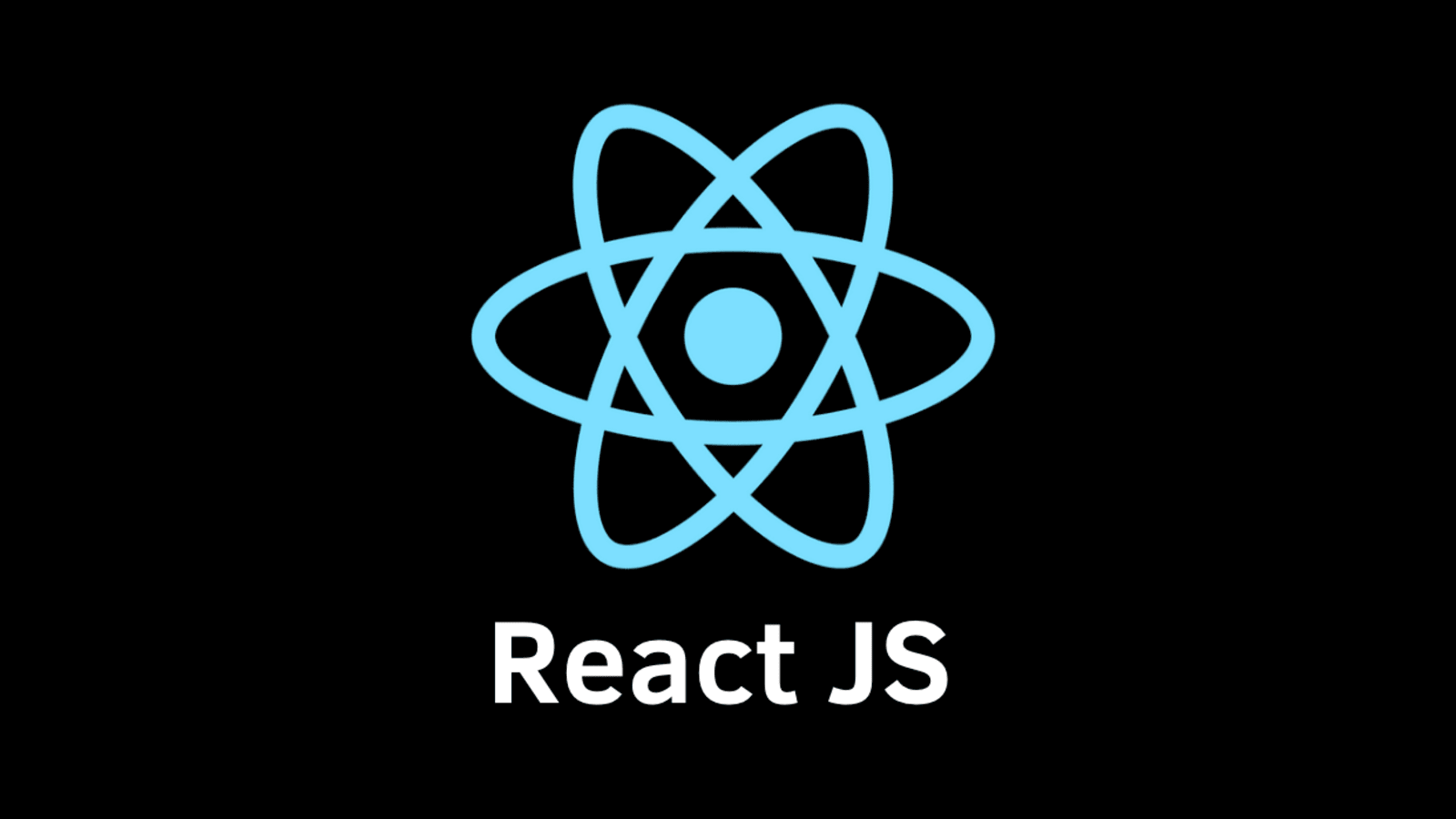
When using Vite, the file structure for a React project may differ slightly compared to other build tools. Here's the typical file structure for a React project created with Vite:
getting-started/
├── node_modules/
├── public/
│ ├── vite.svg
├── src/
│ ├── assets
│ ├── react.svg
│ ├── App.css
│ ├── App.jsx
│ ├── index.css
│ └── main.jsx
├── .eslintrc.cjs
├── .gitignore
├── index.html
├── package-lock.json
├── package.json
└── vite.config.js
Let's explore the purpose of each file and folder in this structure:
- node_modules/: This folder contains the dependencies installed by npm or yarn. It is automatically generated when dependencies are installed.
- public/: This folder contains static assets that are directly copied to the build output folder without being processed by the bundler. It typically includes the index.html file and other static files like images or fonts.
- vite.svg: The favicon for the vite icon.
- src/: This folder contains the source code for the React application.
- App.css: It defines some common styles for the components within the App.jsx component or other components in the project
- App.jsx: The entry point of the React application.
- index.css: A CSS file that contains global styles for the application.
- main.jsx: The entry point for the JavaScript code. It typically renders the React application using ReactDOM.
- .eslintrc.cjs: This is the configuration file for ESLint, a popular JavaScript linting tool. It contains rules and settings for code quality and consistency enforcement.
- .gitignore: A file that specifies which files and folders should be ignored by Git version control.
- index.html: The HTML template used as the entry point for the React application.
- package-lock.json: This file is automatically generated by npm to lock the versions of installed packages. It ensures that the same versions of dependencies are installed across different machines.
- package.json: The package configuration file that contains metadata about the project and its dependencies.
- README.md: A markdown file that provides information and instructions about the project.
- vite.config.js: The configuration file for Vite. It allows you to customize Vite's behavior and apply additional configuration options.
This file structure provides a basic foundation for organizing a React project created with Vite. You can further expand and customize it based on your project requirements and preferences.
When it comes to structuring a React.js project, there are several best practices that can help improve maintainability, scalability, and organization. While the specific structure may vary based on project requirements, here are some general best practices for organizing a React.js project:
1. Group by Feature:
- Organize your project based on features or functionalities. Group related components, styles, and utilities together to improve code discoverability and maintainability.
- Create feature-specific folders or directories to encapsulate related files. For example, a "User" feature might include components, styles, and utilities specific to user-related functionality.
2. Separate Components:
- Create a separate directory for React components. This can be a "components" directory at the top level or a nested directory structure based on your project's needs.
- Group components based on their functionality or purpose. For instance, create subdirectories like "ui" for reusable UI components, "pages" for top-level page components, and "shared" for components used across multiple features.
3. File Naming Conventions:
- Use descriptive and meaningful names for your files to indicate their purpose and content. Follow a consistent naming convention, such as PascalCase for component files (e.g., "MyComponent.jsx") and camelCase for utility or helper files (e.g., "apiUtils.js").
- Consider using index files within directories to provide a clean import syntax. For example, placing an index.js file within a directory allows importing components from that directory without explicitly specifying the component file name.
4. Styles and CSS:
- Place component-specific styles in the same directory as the component itself. This promotes encapsulation and makes it easier to locate and manage styles.
- Consider using CSS-in-JS solutions like styled-components or CSS modules for better component encapsulation and styling organization.
5. Data Management:
- Consider adopting a state management library like Redux or React Context API for managing global or shared state, depending on the complexity and scale of your application.
- Group related actions, reducers, and selectors together in separate directories to keep them organized and maintainable.
6. Tests and Documentation:
- Include separate directories for tests and documentation. Organize tests based on the component or feature they are testing to maintain clarity and ease of navigation.
- Use tools like Jest and React Testing Library for testing React components, and consider generating documentation using tools like Storybook or Styleguidist.
7. Configuration Files and Build Scripts:
- Keep configuration files (e.g., .babelrc, .eslintrc) at the project's root level to maintain consistency across the codebase.
- Store build scripts, such as webpack configurations or Vite's vite.config.js, in their respective files within the project's root directory.
Remember, these best practices provide a starting point, and you can adapt them to fit your project's specific needs and team preferences. Consistency and maintainability are key, so choose a structure that allows for easy navigation, promotes modularity, and aligns with the project's size and complexity.