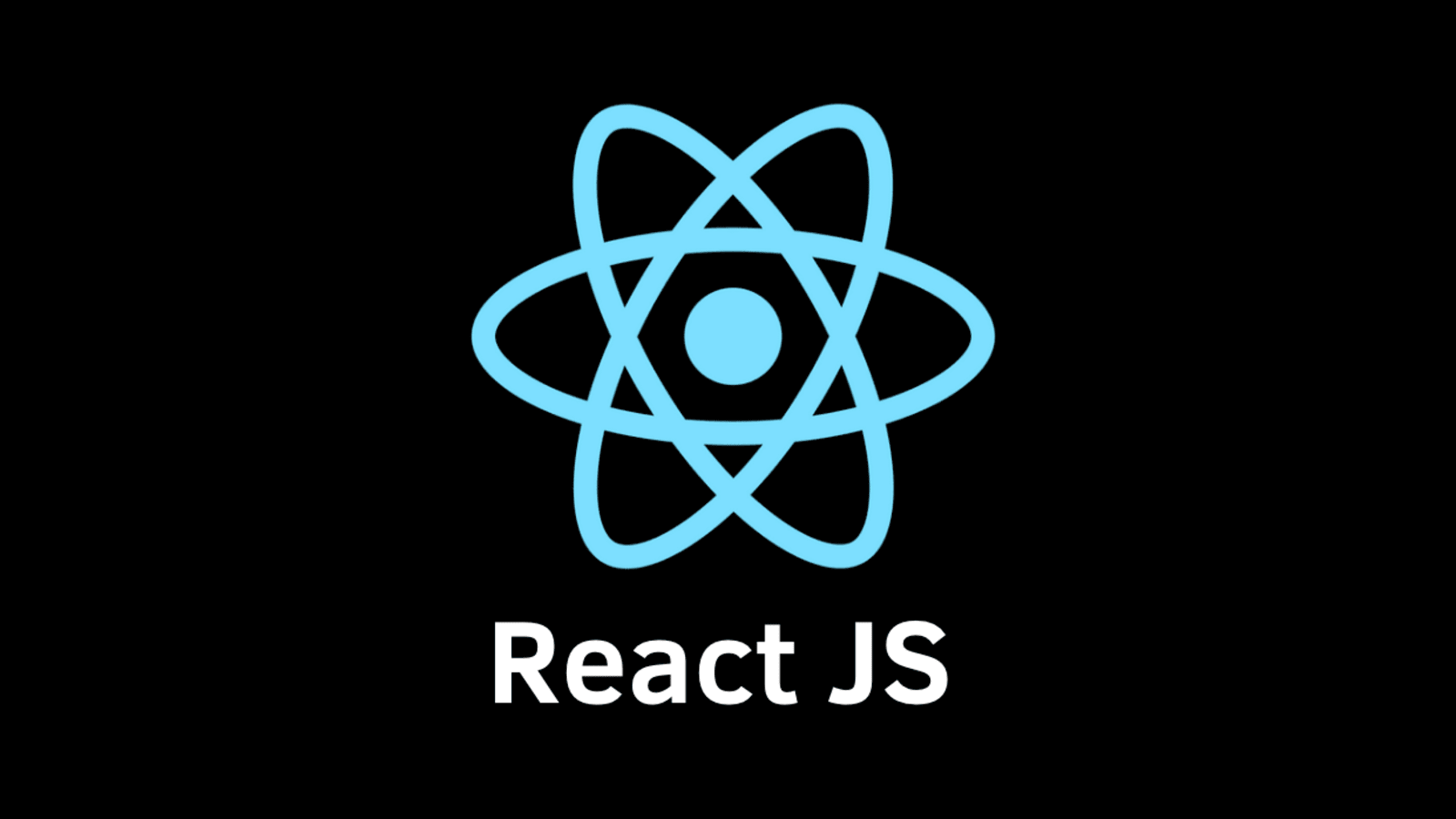
When it comes to building dynamic and interactive user interfaces, React.js has emerged as a popular choice among developers. React's component-based architecture allows for efficient and reusable code, and one of its fundamental concepts is props (short for properties). In this tutorial post, we'll explore the ins and outs of React props, understanding their role, how they work, and their importance in building robust and flexible React applications.
Understanding Props
React props, short for properties, are a fundamental concept in React.js. They allow you to pass data and configuration from a parent component to its child components. Props serve as a way to customize the behavior and appearance of components, making them reusable and versatile.
Here are some key points to understand about React props:
1. Passing Props:
- Props are passed from a parent component to its child component when the child component is rendered.
- To pass props, you include them as attributes when rendering the child component, similar to HTML attributes.
- Props can be any JavaScript value, including strings, numbers, booleans, objects, arrays, or even functions.
2. Receiving Props:
- In a functional component, props are received as a single object argument in the component's function definition.
- In a class component, props are accessed via the `this.props` object.
3. Immutable and Read-Only:
- Props in React are read-only, meaning that child components should not modify the received props directly.
- Modifying props directly can lead to unpredictable behavior and make debugging more challenging.
- Props are intended to be treated as immutable data, allowing components to remain predictable and easier to reason about.
4. Default Props:
- Sometimes, you may want to provide default values for props in case they are not explicitly passed from the parent component.
- React allows you to define default props using the `defaultProps` property.
- Default props ensure that the component functions correctly even if certain props are not provided.
5. Prop Types:
- React provides a way to define the expected types for props using the `propTypes` property.
- Prop types help catch potential bugs by validating the types of props at runtime.
- They also serve as documentation, making it clear what kind of props a component expects.
- Prop types can be defined using the `prop-types` package, which needs to be installed separately.
By leveraging React props effectively, you can create reusable and flexible components. Props enable you to build modular UIs by passing data and configuration down the component tree, promoting code reusability and maintainability.
It's important to note that with the introduction of React Hooks, functional components have become the preferred approach for building components in React, and the usage of class components is gradually diminishing.
Using Props:
To pass props from a parent component to its child component, you simply include them as attributes when rendering the child component. Let's take a look at a basic example:
ParentComponent.jsx:
// ParentComponent.jsx
import React from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = () => {
const name = 'John';
return (
<div>
<ChildComponent name={name} />
</div>
);
};
export default ParentComponent;
ChildComponent.jsx:
// ChildComponent.jsx
import React from 'react';
const ChildComponent = (props) => {
return (
<div>
<h1>Hello, {props.name}!</h1>
</div>
);
};
export default ChildComponent;
In the example above, we passed the `name` prop from the `ParentComponent` to the `ChildComponent`. The `ChildComponent` then receives the prop as an argument in its function component definition and renders it within an `<h1>` element.
Output:
Default Props:
Sometimes, you may want to provide default values for props in case they are not explicitly passed from the parent component. React provides a way to set default props using the `defaultProps` property. Here's an example:
// ChildComponent.jsx
import React from 'react';
const ChildComponent = (props) => {
return (
<div>
<h1>Hello, {props.name}!</h1>
<p>{props.message}</p>
</div>
);
};
ChildComponent.defaultProps = {
message: 'Welcome to my blog!',
};
export default ChildComponent;
In this case, if the `message` prop is not provided by the parent component, the child component will display the default message instead.
Output:
Prop Types:
React allows you to define the expected types for props using the `propTypes` property. This can help catch potential bugs and provide clear documentation for your components. To use prop types, you'll need to install the `prop-types` package. Here's an example:
// ChildComponent.jsx
import React from 'react';
import PropTypes from 'prop-types';
const ChildComponent = (props) => {
return (
<div>
<h1>Hello, {props.name}!</h1>
</div>
);
};
ChildComponent.propTypes = {
name: PropTypes.string.isRequired,
};
export default ChildComponent;
In the example above, we define that the `name` prop is expected to be a string and is required. If the parent component fails to provide a `name` prop or passes a prop of the wrong type, React will log a warning in the browser's console.
Best Practices:
1. Provide Clear and Descriptive Prop Names:
- Use meaningful names for props that accurately describe their purpose and usage.
- Clear and descriptive prop names make it easier for other developers (including yourself) to understand the component's functionality.
2. Document Props with PropTypes:
- Use PropTypes to define the expected types of props and document them.
- PropTypes serve as documentation, making it easier for other developers to understand how to use your component and what types of props are required.
3. Define Default Props:
- Set default values for props using the defaultProps property.
- Default props ensure that the component functions correctly even if certain props are not explicitly provided by the parent component.
4. Avoid Directly Modifying Props:
- Treat props as immutable data and avoid modifying them directly within child components.
- Modifying props directly can lead to unpredictable behavior and make debugging more challenging.
- If you need to modify props, consider creating a local variable within the component and perform modifications on that instead.
5. Destructure Props:
- Destructure props within the component's function parameters or within the component body to improve readability.
- Destructuring allows you to access specific props directly, making the code more concise and easier to understand.
6. Keep Components Small and Focused:
- Aim to keep your components small and focused on a specific task or responsibility.
- Components with fewer props are typically easier to understand and maintain.
- If a component requires too many props, consider breaking it down into smaller, more manageable components.
7. Minimize Prop Dependencies:
- Limit the number of props a component depends on.
- Minimizing prop dependencies improves the component's reusability and reduces the risk of breaking changes when modifying parent components.
8. Consider Using Prop Spreading:
- Prop spreading (using the spread operator) can be helpful when passing multiple props to a child component.
- It simplifies the code by avoiding the need to pass each prop individually and improves readability.
By adhering to these best practices, you can enhance the clarity, maintainability, and reusability of your React components. Consistently following these guidelines can also make your code more accessible to other developers and promote a more efficient and collaborative development process.
Props play a vital role in React development, allowing for the transfer of data and configuration between components. By utilizing props effectively, you can create reusable and flexible components, enabling you to build complex UIs with ease. Understanding how to use props, set default values, and define prop types will empower you to write cleaner, more maintainable code.
Keep exploring the world of React, and don't hesitate to experiment with props to unlock the full potential of this powerful JavaScript library. Happy coding!