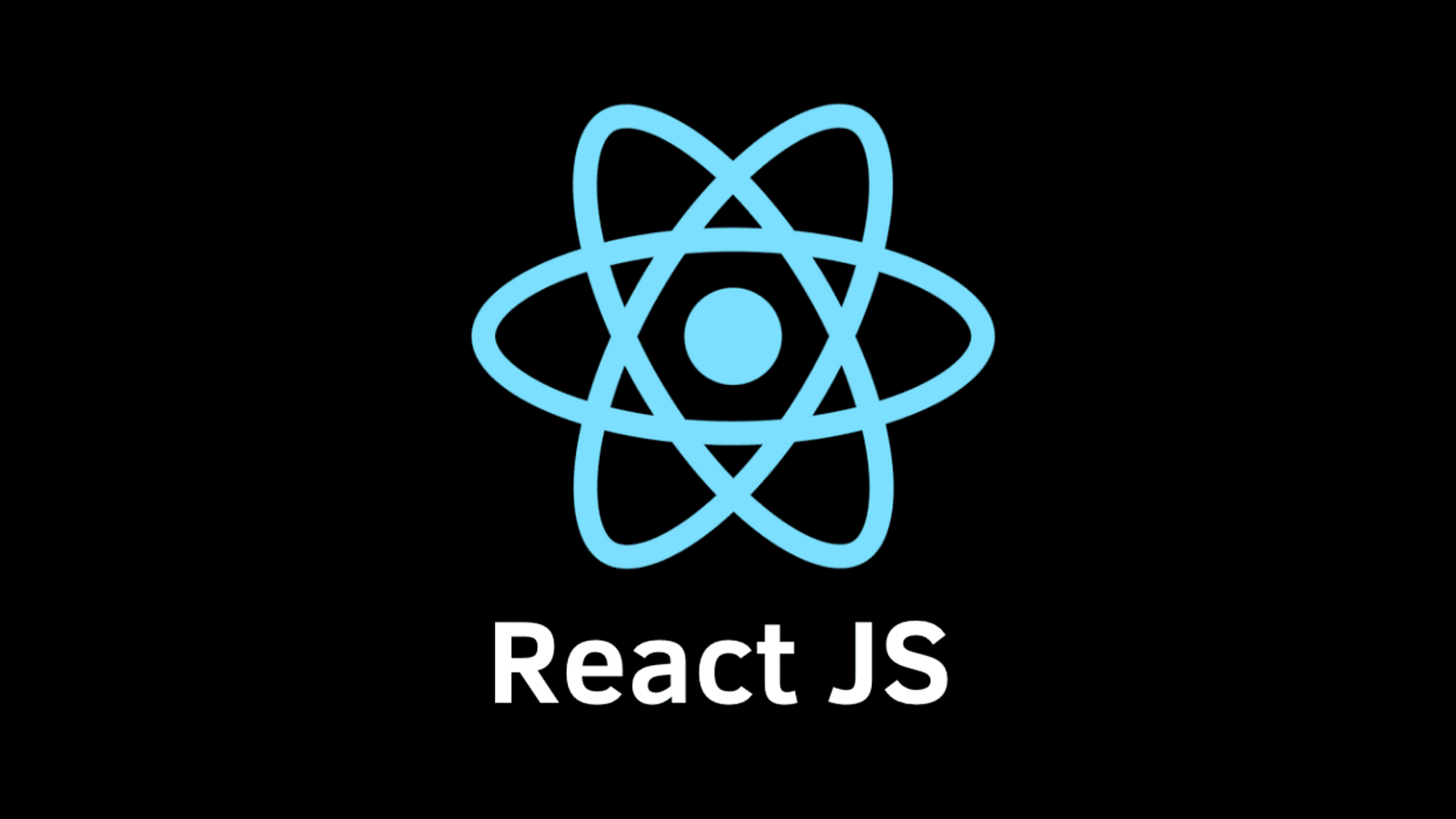
In React.js, iterating over arrays and objects is a common task when working with dynamic data and rendering components dynamically. Array iteration allows you to loop through each element in an array and perform operations, while object iteration enables you to access and manipulate key-value pairs within an object. Understanding how to iterate over arrays and objects in React.js is essential for building interactive and data-driven applications.
Efficient iteration over arrays and objects is a fundamental skill that every developer should master. It allows us to dynamically render data, create reusable components, and build dynamic user interfaces. In this blog post, we'll explore different techniques for iterating over arrays and objects in React.js and demonstrate how to effectively leverage these methods in your projects.
1. Iterating over Arrays:
- Using the map() method: The map() method is a function in JS for iterating over arrays. It allows us to transform each element of an array and return a new array of transformed values. We'll discuss how to use the map() method to dynamically render elements in JSX and handle keys for efficient rendering.
import React from 'react'
export const ArrayIterationMap = () => {
const numbers = [1, 2, 3, 4, 5];
return (
<>
<h3>Array Iteration Map: </h3>
<ul>
{numbers.map((number) => (
<li key={number}>{number}</li>
))}
</ul>
</>
);
}
Output:
- Performing array filtering and sorting: React enables us to filter or sort arrays based on specific conditions. We'll demonstrate how to use array methods like filter() and sort() to manipulate arrays and render a subset of elements or present them in a sorted order.
import React from 'react'
export const ArrayIterationSort = () => {
const numbers = [3, 2, 7, 8, 5];
return (
<>
<h3>Array Iteration Sort: </h3>
<ul>
{numbers.sort((a, b) => a - b).map((number) => (
<li key={number}>{number}</li>
))}
</ul>
</>
);
}
Output:
import React from 'react'
export const ArrayIterationMap = () => {
const numbers = [1, 2, 3, 4, 5];
return (
<>
<h3>Array Iteration Map: </h3>
<ul>
{numbers.map((number) => (
<li key={number}>{number}</li>
))}
</ul>
</>
);
}
Output:
2.Iterating over Objects:
- Utilizing Object.keys() or Object.entries(): When it comes to iterating over objects in React, we can use Object.keys() or Object.entries() to extract keys or key-value pairs from an object. We'll illustrate how to apply these methods to loop through objects and dynamically render components or data based on the object's properties.
import React from 'react';
const ObjectIteration = () => {
const person = {
name: 'John',
age: 30,
occupation: 'Developer'
};
return (
<>
<h3>Object Iteration: </h3>
<ul>
{Object.keys(person).map((property) => (
<li key={property}>
<strong>{property}:</strong> {person[property]}
</li>
))}
</ul>
</>
);
}
export default ObjectIteration;
Output:
- Transforming objects into arrays: In some cases, it may be beneficial to convert objects into arrays before iterating over them. We'll discuss how to convert objects into arrays using Object.keys() or Object.entries(), enabling us to utilize array iteration methods like map() or filter() to work with objects effectively.
import React from 'react'
const ObjectIterationTransform = () => {
const person = {
name: 'John',
age: 30,
occupation: 'Developer'
};
return (
<>
<h3>Object Iteration Transform to array: </h3>
<ul>
{Object.entries(person).map(([key, value]) => (
<li key={key}>
<strong>{key}:</strong> {value}
</li>
))}
</ul>
</>
);
}
export default ObjectIterationTransform;
Output:
- Handling nested objects: Objects often contain nested data structures. We'll explore techniques for handling nested objects, such as using nested loops or recursive functions to iterate through the object hierarchy and render components or access specific values.
import React from 'react'
const ObjectIterationNested = () => {
const person = {
name: 'John',
age: 30,
address: {
street: '123 Main St',
city: 'New York',
country: 'USA'
}
};
return (
<>
<h3>Object Iteration Nested: </h3>
<ul>
{Object.entries(person.address).map(([key, value]) => (
<li key={key}>
<strong>{key}:</strong> {value}
</li>
))}
</ul>
</>
);
}
export default ObjectIterationNested;
Output:
Being proficient in iterating over arrays and objects is crucial for building dynamic and data-driven applications in React.js. In this blog post, we've covered various techniques for iterating over arrays and objects, including utilizing map(), applying conditional rendering, filtering and sorting arrays, and working with objects using Object.keys() or Object.entries(). By mastering these concepts, you'll be equipped to handle complex data structures and create interactive React applications that can dynamically render data based on array or object iteration.