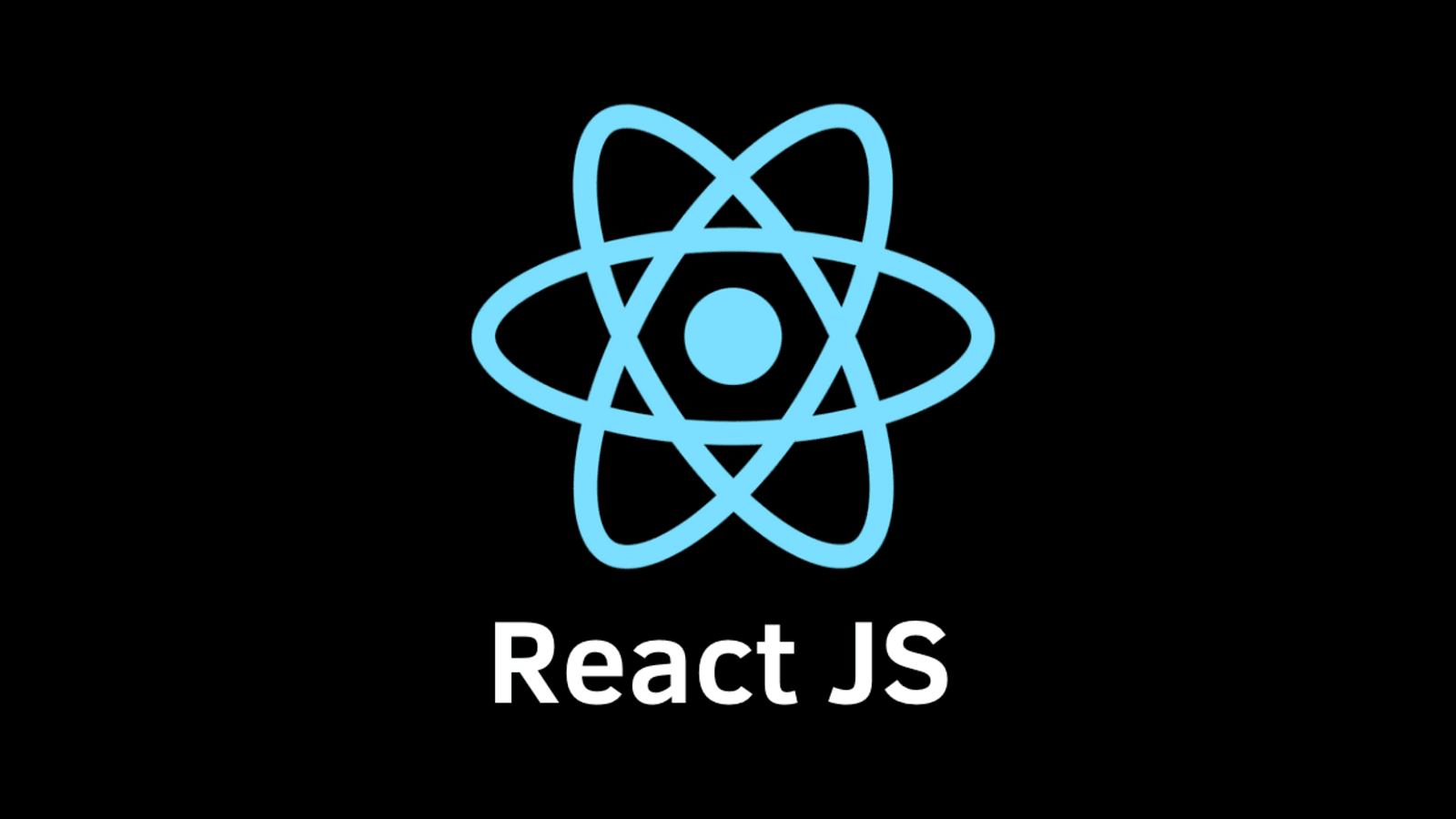
In React.js, handling user interactions and events is a core aspect of building interactive and dynamic user interfaces. One of the most common events is the click event, which occurs when a user clicks on a particular element. In this blog post, we will explore how to handle click events in React.js and demonstrate various techniques to respond to user interactions effectively. The importance of click event handling in web development cannot be overstated. Click events are at the core of user interactions, allowing users to interact with buttons, links, menus, and various other elements on a webpage. Effectively handling click events is crucial for creating intuitive and interactive user interfaces. Here are some reasons why click event handling is important:
- User Interaction: Click events enable users to perform actions and navigate through a website or application. By handling click events properly, developers can provide a seamless and intuitive user experience, allowing users to interact with elements in a meaningful way.
- Triggering Actions: Click events are commonly used to trigger actions such as submitting forms, toggling menus, opening modals, or executing specific functions. By handling click events, developers can initiate the desired actions and respond to user input effectively.
- Form Submissions: When it comes to forms, click events are often associated with form submissions. Validating user input, processing data, and triggering form submissions rely heavily on click events. Proper click event handling ensures that form data is captured accurately and processed correctly.
- Event Delegation: Click event handling allows for efficient event delegation, especially when dealing with dynamic or large sets of elements. Instead of attaching individual click event handlers to each element, event delegation allows a single event handler to handle events for multiple elements. This technique reduces code complexity and improves performance.
- User Feedback and Validation: Click events are instrumental in providing visual feedback to users. For instance, highlighting a clicked button, displaying success or error messages, or updating the UI based on user interactions. Handling click events enables developers to implement interactive feedback mechanisms that enhance the user experience.
- Mobile and Touch Devices: Click events are equally important for touch-based devices. With the proliferation of smartphones and tablets, click event handling becomes essential for delivering a consistent and responsive user experience across different devices. Properly handling touch-based click events ensures that users on mobile devices can interact with elements effortlessly.
- Accessibility: Click event handling plays a significant role in ensuring accessibility for users with disabilities. Providing appropriate keyboard support and handling click events through keyboard actions, such as pressing the Enter key, allows users who rely on assistive technologies to navigate and interact with web content effectively.
In summary, click event handling is vital for creating engaging and interactive web experiences. It empowers users to interact with elements, trigger actions, and navigate through applications. By understanding the importance of click event handling, developers can build user-friendly interfaces, improve usability, and enhance overall user satisfaction.
Understanding the Click Event in React.js:
The click event is triggered when a user clicks on an element in the UI. In React.js, click events are commonly used to handle user interactions such as button clicks, link clicks, or element toggling. Understanding how the click event works in React.js is crucial for effectively handling user interactions.
Handling Click Events in Functional Components:
- Using Inline Event Handlers
Functional components allow us to define event handlers directly inline within the JSX code. Here's an example:
import React from 'react';
const Button = () => {
return (
<button onClick={()=>console.log("Button clicked!")}>Click Me</button>
);
};
export default Button;
In this example, we define a functional component called `Button` that renders a button element. When clicked, it logs the message `'Button clicked!'` to the console.
- Creating Separate Event Handler Functions
Alternatively, we can define the event handler function separately and then reference it within the JSX code. Here's an example:
import React from 'react';
const Button = () => {
const handleClick = () => {
console.log('Button clicked!');
};
return (
<button onClick={handleClick}>Click Me</button>
);
};
export default Button;
In this example, the `handleClick` function is defined separately and then assigned to the `onClick` attribute of the button element. This approach can be useful when you want to reuse the same event handler function in multiple components.
- Event Object and Synthetic Event
When handling click events, React provides access to the event object. Here's an example of accessing the event object in a click event handler:
import React from 'react';
const Button = () => {
const handleClick = (event) => {
console.log('Button clicked!', event);
};
return (
<button onClick={handleClick}>Click Me</button>
);
};
export default Button;
In this example, the `handleClick` function accepts the `event` object as an argument. We can access properties and methods of the event object to gather information about the click event, such as the target element or the mouse position.
- Passing Parameters to Event Handlers
Sometimes, we need to pass additional parameters to the event handler function. We can achieve this by creating a wrapper function. Here's an example:
import React from 'react';
const Button = () => {
const handleClick = (param) => {
console.log('Button clicked with:', param);
};
return (
<button onClick={() => handleClick('Custom parameter')}>Click Me</button>
);
};
export default Button;
In this example, we create an anonymous arrow function in the `onClick` attribute and invoke the `handleClick` function with a custom parameter. This allows us to pass specific values or data to the event handler.
Best Practices for Click Event Handling
- Avoiding Direct DOM Manipulation
When handling click events in React, it's important to follow the React way and avoid direct DOM manipulation. Instead, use React's declarative approach to update the UI by modifying component state or props.
- Debouncing or Throttling Click Events
In scenarios where click events can trigger rapid updates or actions, consider implementing techniques like debouncing or throttling to control the frequency of event execution. Libraries like lodash provide helpful functions for this purpose.
- Proper Cleanup of Event Handlers
When using event handlers, ensure that they are properly cleaned up to avoid memory leaks. In class components, you can use lifecycle methods like `componentWillUnmount` to remove event listeners. In functional components, you can use the `useEffect` hook with a cleanup function.
Handling click events in React.js is fundamental to building interactive and user-friendly applications. In this blog post, we explored various techniques to handle click events in both functional and class components. We discussed passing data, best practices, and advanced techniques for click event handling. Armed with this knowledge, you can effectively respond to user interactions and create engaging React applications.
Remember to choose the technique that best suits your application's needs and follow React's principles for event handling. With practice and experimentation, you'll become adept at handling click events and building dynamic and responsive user interfaces using React.js.