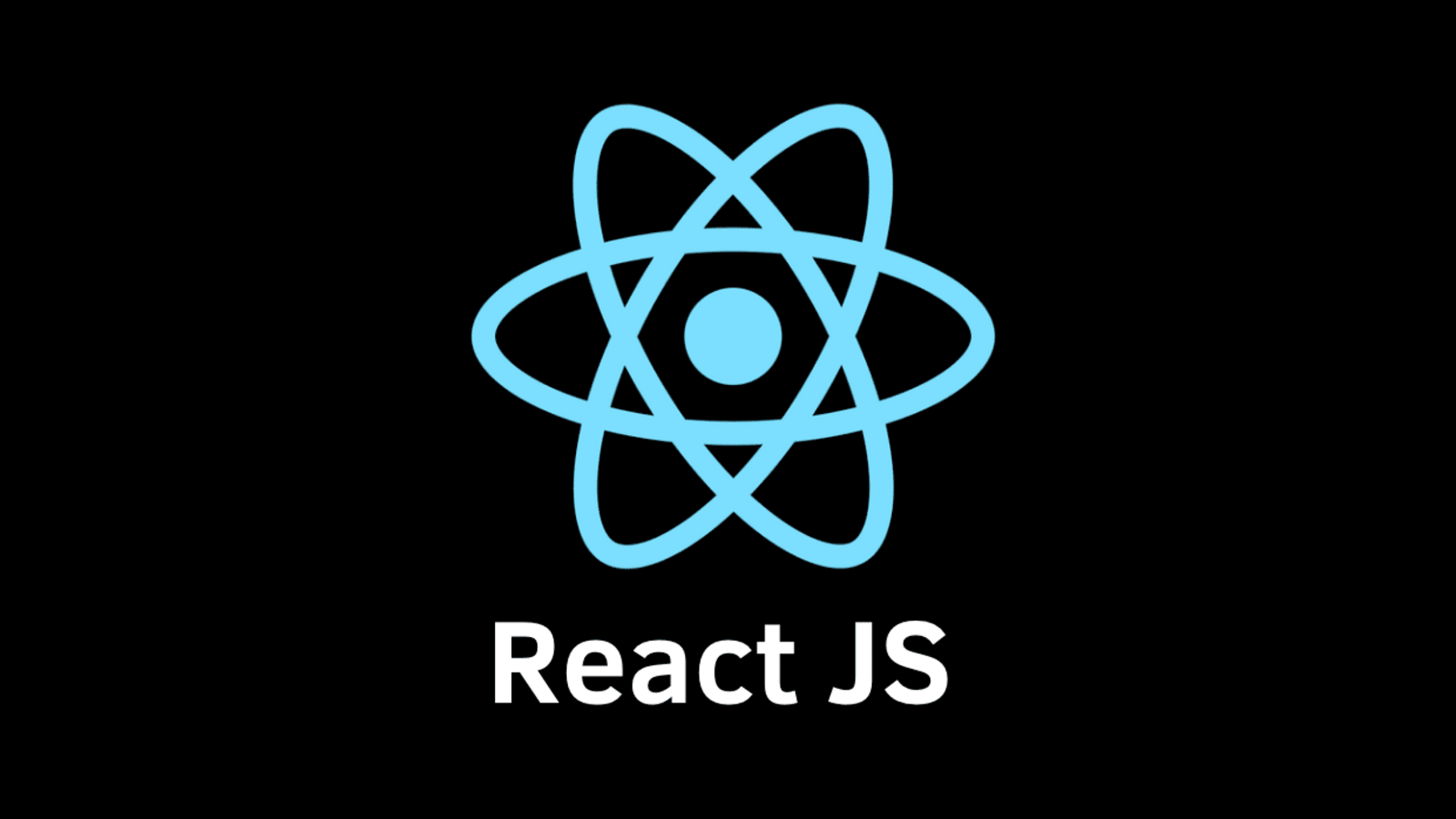
In modern web development, updating existing resources on the server is a crucial requirement. The PUT method allows us to modify data on the server by sending an HTTP request with the updated data. In this tutorial, we will explore how to perform PUT requests in React.js using two popular methods: Fetch API and Axios. We will provide detailed examples and explanations to help you effectively integrate PUT functionality into your React.js applications.
Understanding the PUT Method and its Purpose
The PUT method is an HTTP request method used to update existing resources on the server. It allows us to send the complete updated representation of a resource to the server, replacing the existing resource with the new data. In React.js applications, the PUT method is essential for interacting with APIs that support resource updates.
Characteristics of HTTP PUT Request:
- Purpose of PUT: The HTTP PUT method is used to update or replace an existing resource on the server with the data provided in the request.
- Idempotent: Like the HTTP DELETE request, the PUT request is also considered idempotent. This means that making the same PUT request multiple times will have the same effect as making it just once. Repeated PUT requests with the same data will not create additional or different changes on the server.
- Target URL: The PUT request requires a specific URL (Uniform Resource Locator) that represents the resource to be updated. The URL should uniquely identify the resource to be modified.
- Request Body: The data to be updated is usually sent in the request body. The body can contain any format, but JSON is commonly used for complex data.
- Idempotent Action: PUT is an idempotent action, meaning it is safe to repeat the same PUT request without causing unintended side effects or changes.
Use Cases of HTTP PUT Request:
- Updating User Profile: When a user wants to update their profile information, such as name, email, or profile picture, a PUT request can be used to update the user's data on the server.
- Editing Posts or Articles: In a content management system, authors might want to modify the content of their articles or blog posts. The PUT request can be employed to update the content on the server.
- Managing Inventories: In e-commerce applications, the PUT request can be used to update the quantity or availability of products in the inventory.
- Updating Settings: Users often have the option to change application settings, such as notification preferences or account settings. A PUT request can be used to update these settings on the server.
- Editing Documents: In collaborative platforms, users might need to edit shared documents. The PUT request can be utilized to update the document content on the server.
Making PUT Requests with Fetch API
The Fetch API is a modern web API built into most modern browsers that provides a straightforward way to make HTTP requests. Let's explore how to make PUT requests using the Fetch API in React.js.
import React, { useState } from 'react'
const ApiPutFetch = () => {
const [postData, setPostData] = useState({ id: 1, title: '', body: '' });
const [response, setResponse] = useState(null);
const handleSubmit = (event) => {
event.preventDefault();
fetch(`https://jsonplaceholder.typicode.com/posts/${postData.id}`, {
method: 'PUT',
body: JSON.stringify(postData),
headers: {
'Content-Type': 'application/json',
},
})
.then((response) => response.json())
.then((responseData) => setResponse(responseData))
.catch((error) => console.log(error));
};
return (
<div>
<h2>API PUT with fetch</h2>
<form onSubmit={handleSubmit}>
<input
type="number"
value={postData.id}
onChange={(event) =>
setPostData({ ...postData, id: parseInt(event.target.value) })
}
placeholder="ID"
/>
<input
type="text"
value={postData.title}
onChange={(event) =>
setPostData({ ...postData, title: event.target.value })
}
placeholder="Title"
/>
<textarea
value={postData.body}
onChange={(event) =>
setPostData({ ...postData, body: event.target.value })
}
placeholder="Body"
/>
<button type="submit">Update</button>
</form>
{response && <p>Response: {JSON.stringify(response)}</p>}
</div>
);
}
export default ApiPutFetch;
In this example, we create a form to update an existing post on the JSONPlaceholder API. The `postData` state holds the `id`, `title`, and `body` values for the post to be updated. On form submission, we use the Fetch API to make a PUT request to the JSONPlaceholder `/posts/{id}` endpoint, where `{id}` represents the post ID.
The request method is set to `'PUT'`, and we include the `Content-Type` header as `'application/json'`. The `postData` object is serialized to JSON using `JSON.stringify()` and sent as the request body.
The response is handled using the `.then()` method, where we convert the response to JSON and set it to the `response` state using `setResponse`. Any errors are caught in the `.catch()` block and logged to the console.
As in the example, we send JSON data by setting the `Content-Type` header to `'application/json'` and serializing the `postData` object using `JSON.stringify`. JSONPlaceholder expects JSON data for the PUT request, so this setup works seamlessly.
Making PUT Requests with Axios and JSONPlaceholder
import axios from 'axios';
import React, { useState } from 'react'
const ApiPutAxios = () => {
const [postData, setPostData] = useState({ id: 1, title: '', body: '' });
const [response, setResponse] = useState(null);
const handleSubmit = (event) => {
event.preventDefault();
axios
.put(`https://jsonplaceholder.typicode.com/posts/${postData.id}`, postData)
.then((response) => setResponse(response.data))
.catch((error) => console.log(error));
};
return (
<div>
<h2>API PUT with axios</h2>
<form onSubmit={handleSubmit}>
<input
type="number"
value={postData.id}
onChange={(event) =>
setPostData({ ...postData, id: parseInt(event.target.value) })
}
placeholder="ID"
/>
<input
type="text"
value={postData.title}
onChange={(event) =>
setPostData({ ...postData, title: event.target.value })
}
placeholder="Title"
/>
<textarea
value={postData.body}
onChange={(event) =>
setPostData({ ...postData, body: event.target.value })
}
placeholder="Body"
/>
<button type="submit">Update</button>
</form>
{response && <p>Response: {JSON.stringify(response)}</p>}
</div>
);
}
export default ApiPutAxios
In this example, we use Axios to make a PUT request to the JSONPlaceholder `/posts/{id}` endpoint. We pass the API endpoint and the `postData` object as the request data. Axios automatically handles serializing the data as JSON and setting the appropriate headers.
The response is handled in the `.then()` method, where we set the response data to the `response` state using `setResponse`. Any errors are caught in the `.catch()` block and logged to the console.
Managing Asynchronous Operations with Async/Await
Both Fetch API and Axios support `async/await` syntax, which provides a more concise and synchronous-looking way to handle asynchronous operations. You can rewrite the previous examples using `async/await` to make the code more readable and easier to understand.
Best Practices for PUT Requests in React.js
When working with PUT requests in React.js, it's important to follow certain best practices to ensure secure and efficient resource updates. Here are some key practices to consider:
- Ensure proper authentication and authorization before allowing PUT requests that modify sensitive data.
- Implement client-side form validation to ensure that the data sent in the PUT request meets the server's requirements.
- Use optimistic updates to immediately update the UI with the modified data and provide a smooth user experience while waiting for the server's response.
- Handle errors gracefully and provide meaningful feedback to users if the PUT request fails.
- Test the PUT request thoroughly and handle edge cases, such as handling conflicts when updating resources.
In this tutorial, we explored how to make PUT requests in React.js using both the Fetch API and Axios. We covered the basic setup, provided step-by-step examples, and explained the important concepts and best practices for making PUT API calls. By mastering the PUT method, you can seamlessly integrate resource updates into your React.js applications and interact with APIs that support PUT operations.