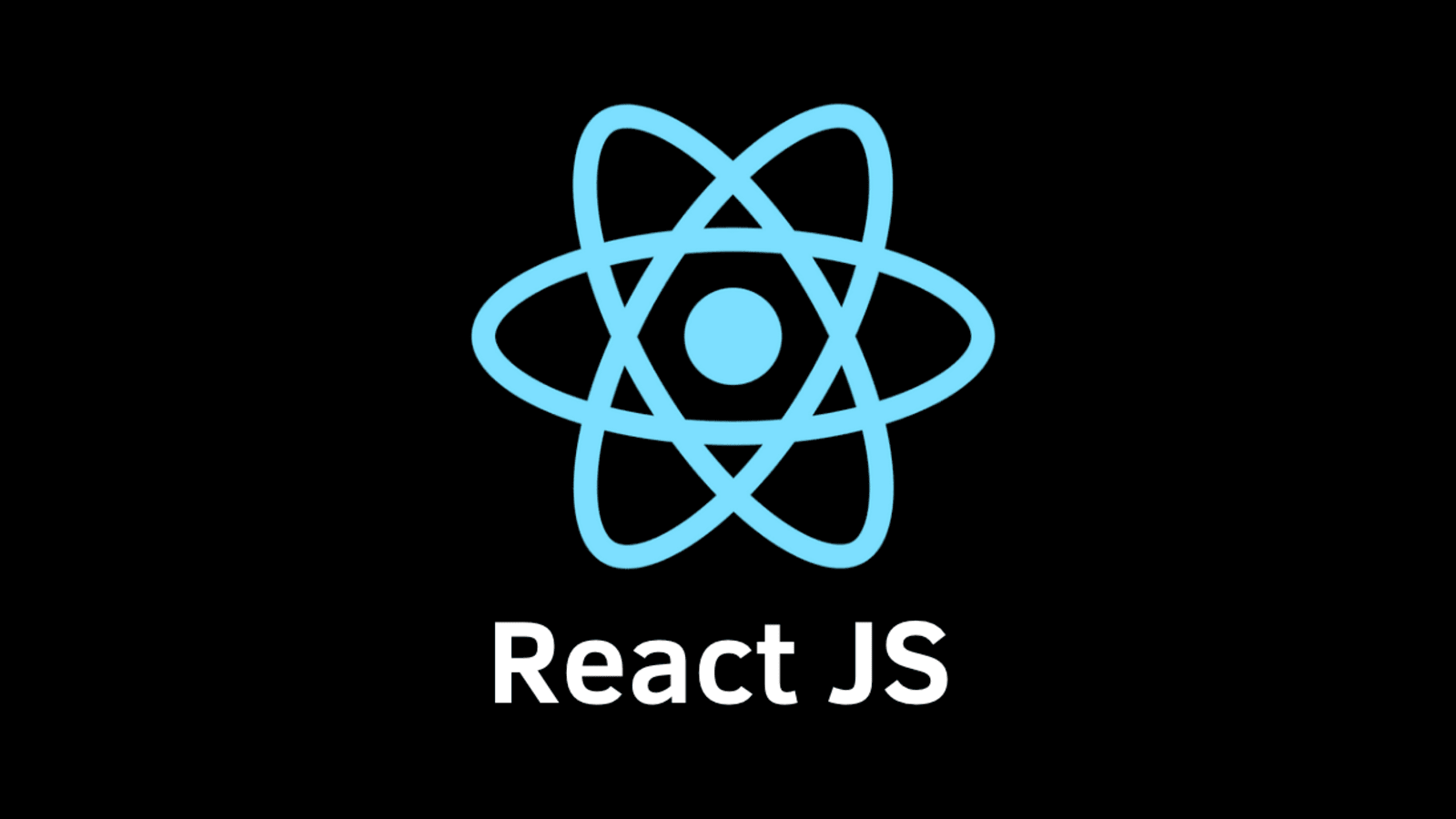
API calls using the POST method are a common requirement in modern web development, enabling data submission and modification. In this tutorial, we will explore how to make POST requests in React.js using two popular methods: Fetch API and Axios. We will provide step-by-step examples and explanations to help you effectively integrate POST functionality into your React.js applications.
Understanding the POST Method
The POST method is one of the HTTP methods used for sending data to a server to create or update a resource. It is commonly used to submit form data, upload files, and perform operations that require data to be sent to the server for processing.
Characteristics of the POST method:
- Data Submission: POST requests are used to send data from the client to the server. The data is included in the body of the request, allowing for more complex and larger payloads compared to other methods like GET.
- Non-Idempotent: Unlike the GET method, POST requests are considered non-idempotent. Performing the same POST request multiple times may result in different outcomes or create duplicate resources on the server. Therefore, caution must be exercised when making POST requests.
- Security Considerations: POST requests are more secure than GET requests for sending sensitive data. Since the data is sent in the request body, it is not visible in the URL or browser history. This makes POST more suitable for operations that involve sensitive information like passwords, credit card details, or personal data.
- Response Expectation: Upon receiving a POST request, the server typically processes the data and sends a response back to the client. The response may include a status code to indicate the success or failure of the request, along with any relevant data or information returned by the server.
Use Cases for the POST method:
- Form Submission: POST requests are commonly used when submitting form data on websites. The form data, such as user input, is sent to the server for processing, storing, or performing an action.
- Data Creation: POST requests are used to create new resources on the server. For example, when adding a new user to a database, a POST request can be made with the user's data, and the server creates a new user entry.
- File Upload: POST requests can be used to upload files from the client to the server. The file data is included in the request body, allowing the server to handle the file and store it accordingly.
- API Operations: POST requests are widely used in APIs to perform various operations, such as creating new records, updating existing records, or triggering specific actions on the server.
- Transactional Operations: POST requests are suitable for performing actions that involve multiple steps or transactions, such as placing an order, initiating a payment, or performing complex business operations.
Making POST Requests with Fetch API
The Fetch API is a modern web API built into most modern browsers that provides a straightforward way to make HTTP requests. Let's explore how to make POST requests using the Fetch API in React.js.
import React, { useState } from 'react'
const ApiPostFetch = () => {
const [postData, setPostData] = useState({ title: '', body: '' });
const [response, setResponse] = useState(null);
const handleSubmit = (event) => {
event.preventDefault();
fetch('https://jsonplaceholder.typicode.com/posts', {
method: 'POST',
body: JSON.stringify(postData),
headers: {
'Content-Type': 'application/json',
},
})
.then((response) => response.json())
.then((responseData) => setResponse(responseData))
.catch((error) => console.log(error));
};
return (
<div>
<h2>POST method with fetch</h2>
<form onSubmit={handleSubmit}>
<input
type="text"
value={postData.title}
onChange={(event) =>
setPostData({ ...postData, title: event.target.value })
}
placeholder="Title"
/>
<br />
<textarea
value={postData.body}
onChange={(event) =>
setPostData({ ...postData, body: event.target.value })
}
placeholder="Body"
/>
<button type="submit">Submit</button>
</form>
{response && <p>Response: {JSON.stringify(response)}</p>}
</div>
);
}
export default ApiPostFetch
In this example, we create a simple form to submit a new post to the JSONPlaceholder API. The postData state holds the title and body values for the post. On form submission, we use the Fetch API to make a POST request to the JSONPlaceholder /posts endpoint. We set the request method to 'POST', include the Content-Type header as 'application/json', and pass the serialized postData object as the request body.
The response is handled using the .then() method, where we convert the response to JSON and set it to the response state using setResponse. Any errors are caught in the .catch() block and logged to the console.
In the example, we are already sending JSON data by setting the Content-Type header to 'application/json' and serializing the postData object using JSON.stringify. JSONPlaceholder expects JSON data for the POST request, so this setup works seamlessly.
Making POST Requests with axios
import axios from 'axios';
import React, { useState } from 'react'
const ApiPostAxios = () => {
const [postData, setPostData] = useState({ title: '', body: '' });
const [response, setResponse] = useState(null);
const handleSubmit = (event) => {
event.preventDefault();
axios
.post('https://jsonplaceholder.typicode.com/posts', postData)
.then((response) => setResponse(response.data))
.catch((error) => console.log(error));
};
return (
<div>
<h2>POST method with axios</h2>
<form onSubmit={handleSubmit}>
<input
type="text"
value={postData.title}
onChange={(event) =>
setPostData({ ...postData, title: event.target.value })
}
placeholder="Title"
/>
<textarea
value={postData.body}
onChange={(event) =>
setPostData({ ...postData, body: event.target.value })
}
placeholder="Body"
/>
<button type="submit">Submit</button>
</form>
{response && <p>Response: {JSON.stringify(response)}</p>}
</div>
);
}
export default ApiPostAxios;
In this example, we use Axios to make a POST request to the JSONPlaceholder /posts endpoint. We use the axios.post method and pass the API endpoint and the postData object as the request data. Axios automatically handles serializing the data as JSON and setting the appropriate headers.
The response is handled in the .then() method, where we set the response data to the response state using setResponse. Any errors are caught in the .catch() block and logged to the console.
Similar to the Fetch API example, we handle the response and errors using the `.then()` and `.catch()` methods, respectively. The response data is stored in the state, and errors are logged to the console. You can customize the error handling based on your application's requirements.
Both Fetch API and Axios support `async/await` syntax, which provides a more concise and synchronous-looking way to handle asynchronous operations. You can rewrite the previous examples using `async/await` to make the code more readable and easier to understand.
Best Practices for POST Requests in React.js
When working with POST requests in React.js, it's essential to follow certain best practices to ensure secure and efficient data submission. Here are some key practices to consider:
- Validate and sanitize user input before sending it to the server to prevent security vulnerabilities like SQL injection or cross-site scripting (XSS) attacks.
- Use SSL/TLS encryption (HTTPS) to secure the transmission of sensitive data.
- Implement proper error handling and provide meaningful error messages to users when a POST request fails.
- Consider implementing CSRF protection to prevent cross-site request forgery attacks.
- Implement server-side input validation and ensure that your server validates the incoming data to maintain data integrity.
- Utilize server-side throttling and rate limiting mechanisms to prevent abuse and protect server resources.
- Follow RESTful API conventions for structuring your POST endpoints and handling different HTTP status codes.
Consider using libraries or frameworks that provide form validation and data serialization utilities to simplify data handling and submission.
By adhering to these best practices, you can ensure the security, reliability, and performance of your POST requests in React.js applications.