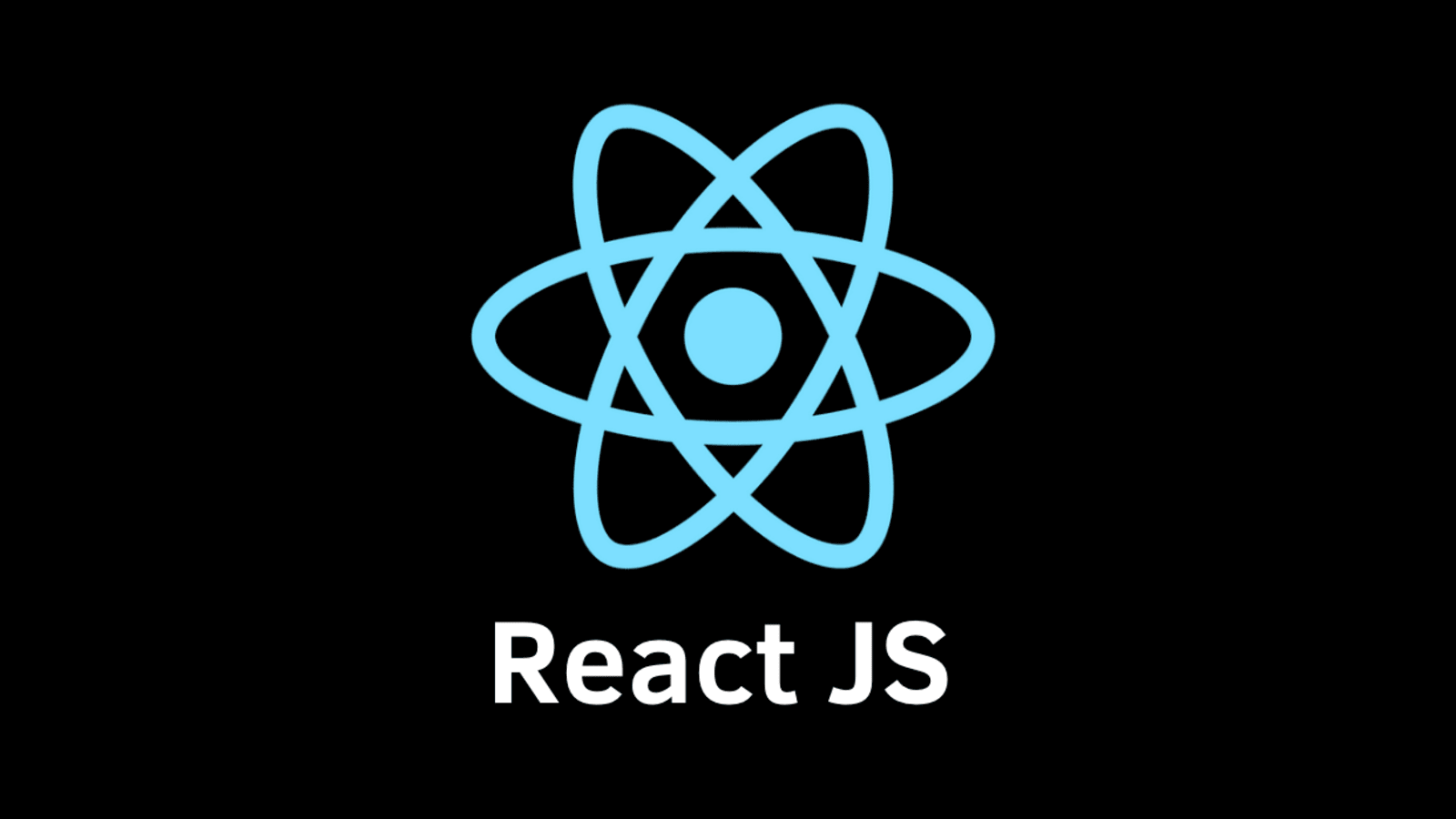
In modern web development, the DELETE method is essential for removing data from the server. It allows us to delete resources stored on the server by sending an HTTP request with the resource's unique identifier. In this tutorial, we will explore how to perform DELETE requests in React.js using two popular methods: Fetch API and Axios. We will provide detailed examples and explanations to help you effectively integrate DELETE functionality into your React.js applications.
Understanding the DELETE Method and its Purpose
The DELETE method is an HTTP request method used to delete resources on the server. It allows us to specify the unique identifier of the resource to be deleted. In React.js applications, the DELETE method is crucial for interacting with APIs that support resource deletion.
Characteristics of DELETE Request:
- Purpose: The DELETE request is an HTTP method used to delete a resource on the server. It requests the server to remove the specified resource permanently.
- Idempotent: Like the GET request, the DELETE request is also considered idempotent, meaning multiple identical DELETE requests will have the same effect as a single request. Subsequent DELETE requests on the same resource won't change the server state further.
- URL: The URL of the DELETE request typically contains the unique identifier (such as an ID or a resource path) of the resource to be deleted.
- No Request Body: Unlike the POST and PUT requests, the DELETE request usually does not contain a request body. The resource to be deleted is identified directly from the URL.
- Response: The server responds with an HTTP status code to indicate the success or failure of the DELETE operation. Common status codes include 200 OK for a successful deletion, 404 Not Found if the resource doesn't exist, and 204 No Content if the resource was successfully deleted but there's no additional data to return.
Use Cases of DELETE Request:
- Deleting Records: The DELETE request is commonly used to delete records from databases or remove items from collections, such as deleting a user account, removing a blog post, or eliminating a comment.
- Data Cleanup: DELETE requests are employed for cleaning up unnecessary or outdated data from the server, ensuring a lean and well-maintained database.
- Resource Management: In RESTful APIs, DELETE requests are used to manage resources, where a resource can be anything from a user profile to a product in an e-commerce store.
- User Actions: DELETE requests can be triggered by user actions in web applications, such as deleting items from a shopping cart or removing items from a to-do list.
- API Maintenance: API administrators use DELETE requests to remove deprecated endpoints or resources that are no longer needed in the API.
- Security and Privacy: DELETE requests are vital for managing users' personal data, allowing them to delete their accounts or personal information.
- Cascading Deletion: In some scenarios, a DELETE request might be designed to trigger cascading deletions, where related resources or child elements are also deleted when the parent resource is removed.
Making DELETE Requests with Fetch API
The Fetch API is a modern web API built into most modern browsers that provides a straightforward way to make HTTP requests. Let's explore how to make DELETE requests using the Fetch API in React.js.
import React, { useState } from 'react'
const ApiDeleteFetch = () => {
const [postId, setPostId] = useState('');
const [response, setResponse] = useState(null);
const handleDelete = () => {
fetch(`https://jsonplaceholder.typicode.com/posts/${postId}`, {
method: 'DELETE',
})
.then((response) => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(() => {
setResponse('Post deleted successfully.');
})
.catch((error) => console.log(error));
};
return (
<div>
<h2>API DELETE with fetch</h2>
<input
type="number"
value={postId}
onChange={(event) => setPostId(event.target.value)}
placeholder="Enter Post ID"
/>
<button onClick={handleDelete}>Delete Post</button>
{response && <p>{response}</p>}
</div>
);
}
export default ApiDeleteFetch;
In this example, we create an input field to enter the ID of the post to be deleted. When the "Delete Post" button is clicked, the `handleDelete` function is triggered. Inside this function, we use the Fetch API to make a DELETE request to the JSONPlaceholder `/posts/{id}` endpoint, where `{id}` represents the post ID.
If the response is successful (status code 200-299), we set the `response` state to "Post deleted successfully." Otherwise, we throw an error and handle it in the `.catch()` block.
Handling Deletion Confirmation
For better user experience, it's a good practice to confirm the deletion before sending the DELETE request. Let's modify the example to include a confirmation dialog.
import React, { useState } from 'react'
const ApiDeleteFetch = () => {
const [postId, setPostId] = useState('');
const [response, setResponse] = useState(null);
const handleDelete = () => {
if (window.confirm('Are you sure you want to delete this post?')) {
fetch(`https://jsonplaceholder.typicode.com/posts/${postId}`, {
method: 'DELETE',
})
.then((response) => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(() => {
setResponse('Post deleted successfully.');
})
.catch((error) => console.log(error));
}
};
return (
<div>
<h2>API DELETE with fetch</h2>
<input
type="number"
value={postId}
onChange={(event) => setPostId(event.target.value)}
placeholder="Enter Post ID"
/>
<button onClick={handleDelete}>Delete Post</button>
{response && <p>{response}</p>}
</div>
);
}
export default ApiDeleteFetch;
In this modified example, we wrap the DELETE request inside an `if (window.confirm())` statement. This will show a confirmation dialog with the message "Are you sure you want to delete this post?" When the user clicks "OK," the DELETE request is sent, and when they click "Cancel," nothing happens.
Error Handling
In the examples, we handle errors by checking the response status in the `.then()` chain and throwing an error if the status is not in the range of 200-299. This ensures that we catch any network or server-related errors during the DELETE request.
Utilizing Axios for DELETE Requests
Axios is a popular JavaScript library for making HTTP requests. Let's explore how to use Axios for making DELETE requests in React.js.
import axios from 'axios';
import React, { useState } from 'react'
const ApiDeleteAxios = () => {
const [postId, setPostId] = useState('');
const [response, setResponse] = useState(null);
const handleDelete = () => {
if (window.confirm('Are you sure you want to delete this post?')) {
axios
.delete(`https://jsonplaceholder.typicode.com/posts/${postId}`)
.then((response) => {
setResponse('Post deleted successfully.');
})
.catch((error) => console.log(error));
}
};
return (
<div>
<h2>API DELETE with axios</h2>
<input
type="number"
value={postId}
onChange={(event) => setPostId(event.target.value)}
placeholder="Enter Post ID"
/>
<button onClick={handleDelete}>Delete Post</button>
{response && <p>{response}</p>}
</div>
);
}
export default ApiDeleteAxios;
In this Axios example, we use the `.delete` method to make a DELETE request to the JSONPlaceholder `/posts/{id}` endpoint, similar to the Fetch API example. The confirmation dialog is also included to ensure that the user confirms the deletion.
The response is handled in the `.then()` method, where we set the `response` state to "Post deleted successfully." Any errors are caught in the `.catch()` block and logged to the console
.
Managing Asynchronous Operations with Async/Await
Both Fetch API and Axios support `async/await` syntax, which provides a more concise and synchronous-looking way to handle asynchronous operations. You can rewrite the previous examples using `async/await` to make the code more readable and easier to understand.
Best Practices for DELETE Requests in React.js
When working with DELETE requests in React.js, it's important to follow certain best practices to ensure secure and efficient resource deletion. Here are some key practices to consider:
- Implement proper authentication and authorization before allowing DELETE requests that modify sensitive data.
- Provide a confirmation dialog to users before executing the DELETE request to prevent accidental deletions.
- Handle errors gracefully and provide meaningful feedback to users if the DELETE request fails.
- Test the DELETE request thoroughly and handle edge cases, such as handling conflicts or ensuring idempotency.
In this tutorial, we explored how to make DELETE requests in React.js using both the Fetch API and Axios. We covered the basic setup, provided step-by-step examples, and explained the important concepts and best practices for making DELETE API calls. By mastering the DELETE method, you can seamlessly integrate resource deletion into your React.js applications and interact with APIs that support DELETE operations.