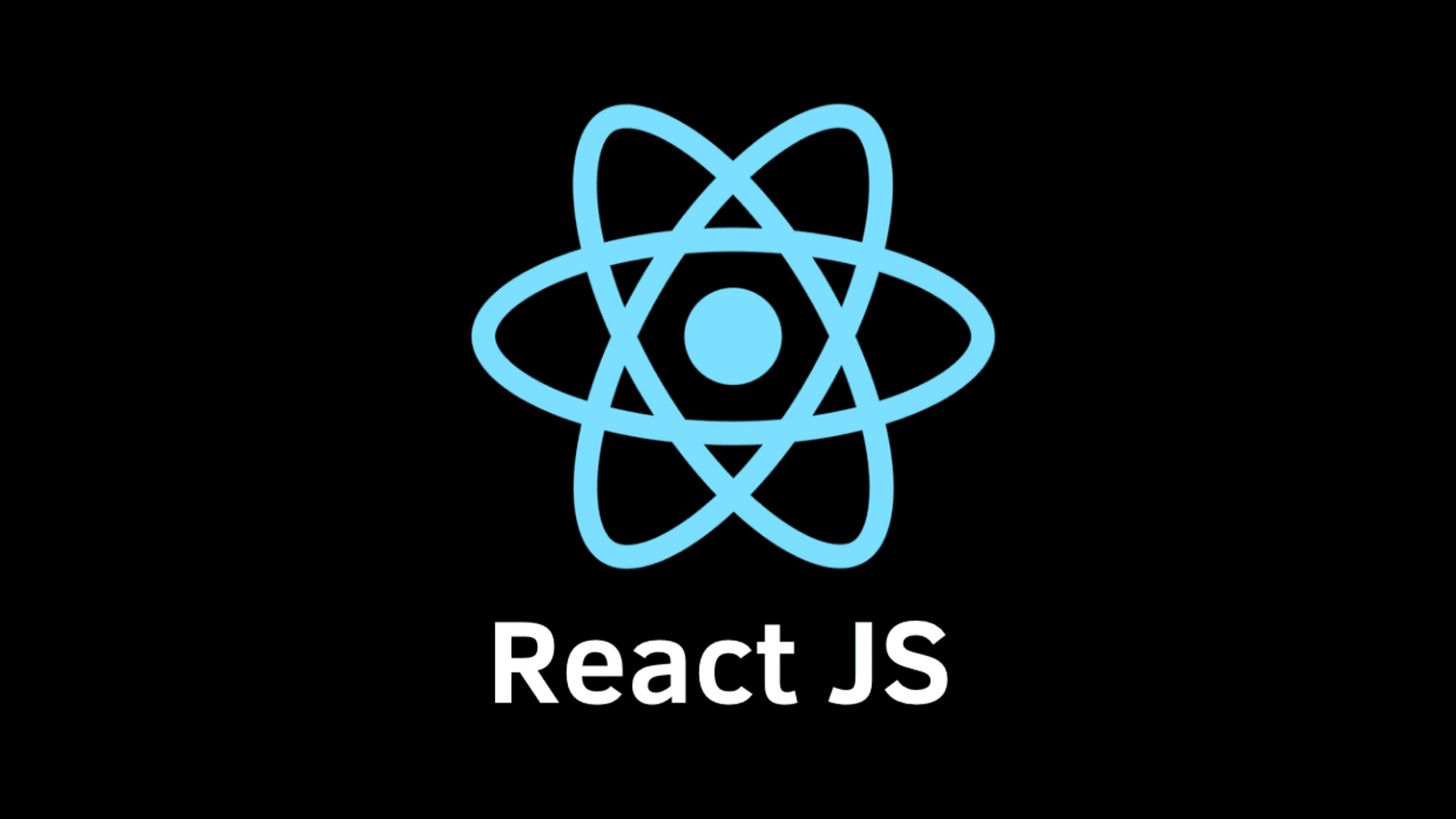
In the world of modern web development, integrating with external APIs (Application Programming Interfaces) has become an essential part of building data-driven applications. React, with its component-based architecture and powerful ecosystem, provides an excellent framework for making API calls. In this blog post, we will explore the process of making API calls in React, covering different approaches, best practices, and common challenges.
Understanding API Calls:
APIs serve as interfaces that allow different software applications to communicate and exchange data. API calls involve sending HTTP requests to an API endpoint, receiving a response, and processing that data within a React application. APIs can provide a wide range of functionalities, including fetching data from a remote server, submitting data, or performing specific operations.
Approaches for Making API Calls:
There are multiple approaches for making API calls in React, depending on your project requirements and preferences. Here are three common approaches:
1. Fetch API:
- The Fetch API is a built-in web API that provides a simple and powerful way to fetch resources asynchronously.
- It uses JavaScript Promises to handle asynchronous operations and works well with modern browsers.
- The Fetch API supports various HTTP methods (GET, POST, PUT, DELETE) and allows you to handle response data using JSON parsing or other techniques.
import React, { useEffect, useState } from 'react';
const ApiFetch = () => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetch('https://jsonplaceholder.typicode.com/users')
.then(response => response.json())
.then(data => {
setData(data);
setLoading(false);
})
.catch(error => {
console.error('Error:', error);
setLoading(false);
});
}, []);
return (
<div>
{loading ? (
<p>Loading...</p>
) : (
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
)}
</div>
);
};
export default ApiFetch;
The ApiFetch functional component demonstrates how to make API calls using the Fetch API in React. Within the useEffect hook, a GET request is made to JSONPlaceholder's /users endpoint. The response is handled using the response.json() method to parse the JSON data. The retrieved data is then stored in the component's state using the setData function. While the API request is in progress, a loading message is displayed. Once the data is fetched, it is mapped over and rendered as a list of post titles.
2. Axios:
- Axios is a popular third-party library that provides an elegant and easy-to-use API for making HTTP requests.
- It supports a wide range of features, including interceptors, request cancellation, and automatic JSON parsing.
- Axios works both in the browser and Node.js environments, making it versatile for server-side rendering or universal React applications.
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const ApiAxios = () => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
axios.get('https://jsonplaceholder.typicode.com/users')
.then(response => {
setData(response.data);
setLoading(false);
})
.catch(error => {
console.error('Error:', error);
setLoading(false);
});
}, []);
return (
<div>
<h3>API calling using axios: </h3>
{loading ? (
<p>Loading...</p>
) : (
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
)}
</div>
);
};
export default ApiAxios;
The ApiAxios functional component illustrates how to make API calls using Axios in React. Similar to the Fetch API example, a GET request is made to JSONPlaceholder's /users endpoint within the useEffect hook. The Axios library simplifies the request with its intuitive syntax. The response data is stored in the component's state using the setData function, and a loading message is displayed while the request is in progress. The retrieved data is then mapped over and rendered as a list of post titles.
Comparison between the Fetch API and Axios
1. Ease of Use:
- Fetch API: The Fetch API provides a straightforward and modern interface for making API calls. It uses the fetch() function to send HTTP requests and returns a Promise that resolves to the response.
- Axios: Axios offers a simpler and more developer-friendly syntax for making API calls. It provides a higher-level API with methods like axios.get(), axios.post(), etc., that handle the request and response objects more intuitively.
2. Browser Support:
- Fetch API: The Fetch API is natively supported in modern browsers, including Chrome, Firefox, Safari, and Edge. However, some older browsers may require a polyfill to use the Fetch API.
- Axios: Axios supports a wider range of browsers, including older versions and Internet Explorer, by using a combination of the XHR and Promise APIs. It provides better compatibility without requiring additional polyfills.
3. Error Handling:
- Fetch API: The Fetch API has built-in error handling capabilities. If a network error occurs or if the response status code is not in the 200-299 range, the Promise is rejected, and you can handle the error using .catch().
- Axios: Axios also has built-in error handling. It automatically rejects the Promise when an HTTP error status code is received (e.g., 404, 500). Additionally, Axios allows you to intercept and handle errors globally using interceptors.
4. Request and Response Interceptors:
- Fetch API: The Fetch API does not have built-in support for request and response interceptors. You would need to handle intercepting and modifying requests or responses manually.
- Axios: Axios provides a powerful feature of interceptors. Interceptors allow you to intercept and modify requests or responses before they are sent or received. This can be useful for adding headers, logging, authentication, or error handling.
5. Request Cancellation:
- Fetch API: The Fetch API does not have native support for cancelling requests. Once a request is initiated, it cannot be easily cancelled.
- Axios: Axios provides built-in cancellation support by using the Cancel Token feature. You can create a cancel token, associate it with a request, and then cancel the request at any time. This can be useful in scenarios like canceling ongoing requests when a user navigates away from a component.
6. Additional Features:
- Fetch API: The Fetch API provides basic functionality for making API calls. It supports custom headers, different HTTP methods, and handling JSON data. However, additional functionality like automatic request/response transformation, timeout handling, and concurrent requests require additional code.
- Axios: Axios offers a rich feature set out of the box. It provides automatic request/response transformation (e.g., JSON parsing), header customization, timeout handling, concurrent requests, and more. It simplifies common API-related tasks, reducing the amount of boilerplate code required.
In conclusion, both the Fetch API and Axios have their strengths and are suitable for making API calls in React. The Fetch API is a built-in, lightweight option with broad browser support. Axios, on the other hand, provides a more developer-friendly interface, better browser compatibility, built-in error handling, request/response interceptors, and cancellation support. The choice between the two ultimately depends on the specific requirements of your project and your preference for simplicity or additional features.
Best Practices :
To ensure efficient and reliable API calling in your React applications, consider the following best practices:
1. Separate Concerns:
- Isolate API calling logic in a separate module or custom hook to keep components clean and focused on rendering UI.
- Separating concerns makes your code more maintainable and allows for better reusability.
2. Use Async/Await or Promises:
- Utilize the power of modern JavaScript syntax by using async/await or Promises for handling asynchronous API requests.
- These approaches make your code more readable and easier to manage.
3. Handle Errors:
- Implement error handling mechanisms to gracefully deal with failed API requests.
- Consider using try/catch blocks or handling errors within Promise chains to capture and handle potential errors.
4. Set Loading States:
- Set loading states to provide feedback to the user while the API request is in progress.
- Use loading indicators or skeleton components to indicate that data is being fetched.
5. Implement Caching and Debouncing:
- Consider caching API responses to minimize unnecessary network requests and improve performance.
- Implement debouncing techniques to optimize API calls for scenarios like search inputs or auto-suggest functionality.
6. Security Considerations:
- When working with sensitive data or APIs requiring authentication, follow best practices for securing API calls.
- Use authentication mechanisms like tokens or API keys, and encrypt sensitive data transmitted over the network.
API calling is a crucial aspect of building data-driven React applications. Whether you choose the Fetch API, Axios, or built-in hooks, the goal is to efficiently retrieve data from APIs and integrate it into your React components. By following best practices such as separating concerns, handling errors, and implementing caching strategies, you can create robust and performant applications that seamlessly interact with external data sources.
Embrace the power of APIs in your React projects, and let the world of data and functionality be at your fingertips. Happy coding!