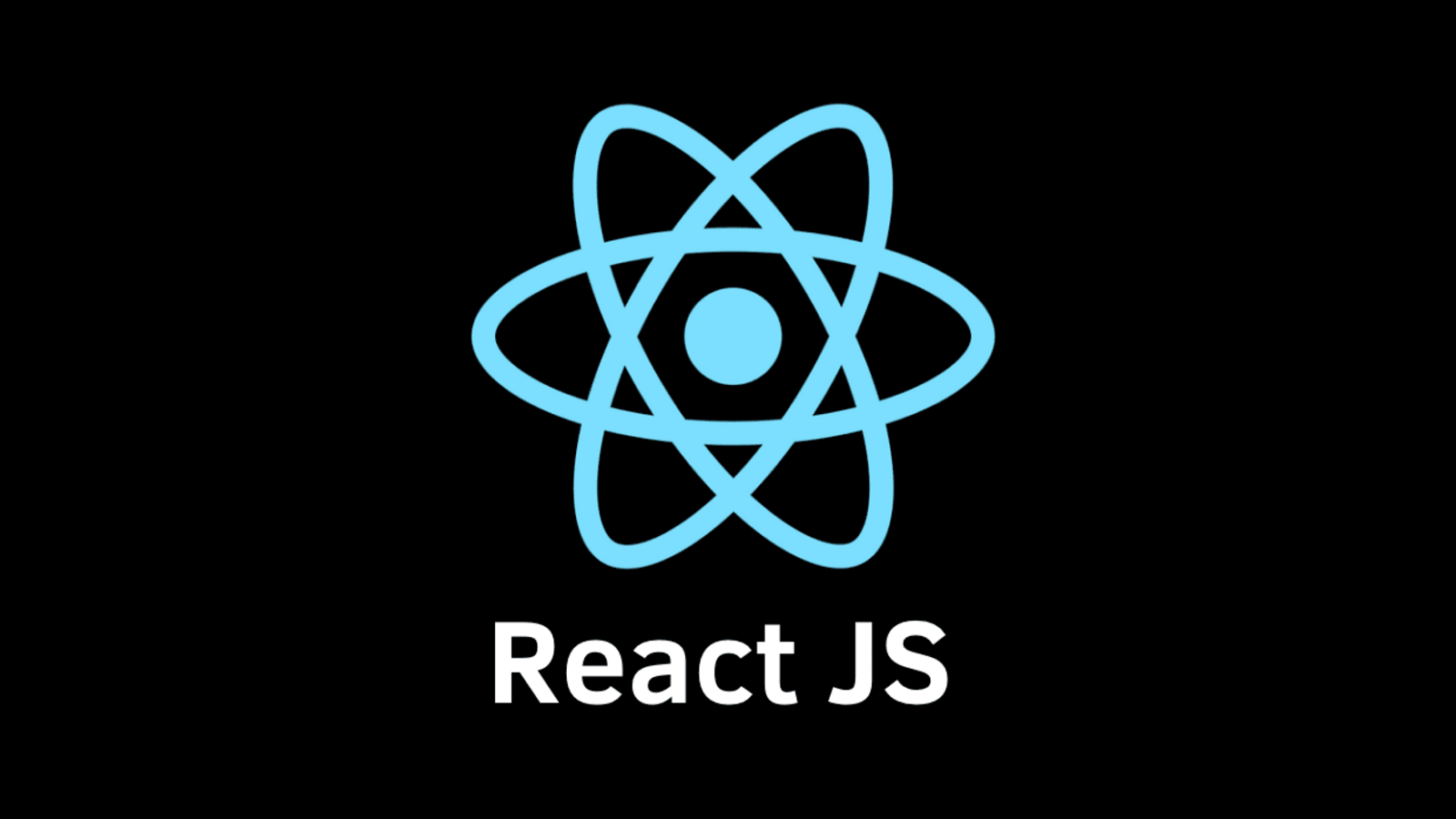
Introduction:
React Hooks revolutionized the way we manage state and side effects in React components. Introduced in React 16.8, Hooks provide a more elegant and functional approach to building React applications. In this blog post, we will explore the concept of React Hooks, understand their purpose, and delve into their various use cases. By harnessing the power of Hooks, developers can write cleaner, more reusable code and enhance the efficiency and scalability of their React applications.
Understanding React Hooks:
React Hooks are functions that enable functional components to manage state, handle side effects, and access React's features without using class components. They allow us to reuse logic and avoid complex component hierarchies by separating concerns into independent functions.
- State Management with useState(): The useState() Hook is used to manage state within functional components. It enables us to define and update state variables without the need for class components. With useState(), we can easily handle local component state, including primitive types, objects, and arrays.
- Managing Side Effects with useEffect(): The useEffect() Hook provides a way to handle side effects within functional components. It replaces lifecycle methods such as componentDidMount, componentDidUpdate, and componentWillUnmount. useEffect() allows us to perform actions like data fetching, subscriptions, or DOM manipulation after the component renders.
- Custom Hooks for Reusability: Custom Hooks allow us to encapsulate and reuse logic across different components. By extracting common functionality into custom Hooks, we can abstract away complex logic and make it more shareable and maintainable. Custom Hooks follow a naming convention of starting with the word "use" and can be used just like built-in Hooks.
- useContext() for Global State Management: The useContext() Hook facilitates global state management by providing access to a context created with React's Context API. It enables components to access shared data without manually passing props through multiple levels of the component tree.
- Additional Hooks and Advanced Use Cases: React provides several other Hooks, such as useRef(), useMemo(), and useCallback(), for specific use cases. useRef() allows us to hold mutable values that persist across renders, while useMemo() and useCallback() optimize expensive computations and prevent unnecessary re-renders.
Examples:
useState():
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
};
export default Counter;
In this example, the useState() Hook is used to manage the count state variable. The count state starts with an initial value of 0, and the setCount function is used to update the state when the button is clicked. This Hook simplifies state management within functional components.
useEffect():
import React, { useState, useEffect } from 'react';
const UserProfile = () => {
const [user, setUser] = useState(null);
useEffect(() => {
// Simulating API call to fetch user data
fetch('https://api.example.com/user')
.then(response => response.json())
.then(data => setUser(data));
}, []);
return (
<div>
{user ? (
<div>
<h2>{user.name}</h2>
<p>Email: {user.email}</p>
</div>
) : (
<p>Loading user profile...</p>
)}
</div>
);
};
export default UserProfile;
In this example, the useEffect() Hook is used to fetch user data from an API when the component mounts. The empty dependency array [] ensures that the effect runs only once. The retrieved user data is stored in the user state, which is used to conditionally render the user profile or a loading message.
useContext():
import React, { useContext } from 'react';
const ThemeContext = React.createContext('light');
const ThemeToggle = () => {
const theme = useContext(ThemeContext);
return (
<div>
<p>Current theme: {theme}</p>
<button>Toggle Theme</button>
</div>
);
};
export default ThemeToggle;
In this example, the useContext() Hook is used to access the value of the ThemeContext created with React's Context API. It allows components to consume the shared theme data without having to pass it down through props manually. The theme value from the context is used to display the current theme and can be utilized for dynamic theming.
Best Practices and Considerations:
- Understand the rules of Hooks, such as calling them at the top level of a component and not inside loops or conditions.
- Utilize the power of Hooks to simplify and modularize your components.
- Separate concerns by creating custom Hooks to encapsulate and share logic.
- Leverage the useEffect() cleanup function to prevent memory leaks and perform cleanup tasks.
Conclusion:
React Hooks have transformed the way we write React applications, providing a more functional and concise approach to managing state and side effects. With useState(), useEffect(), and other Hooks, developers can streamline their code, increase reusability, and enhance performance. By embracing the power of Hooks, you can unlock a world of possibilities and build robust, scalable React applications with ease. So, dive into the world of Hooks, and elevate your React development to new heights!