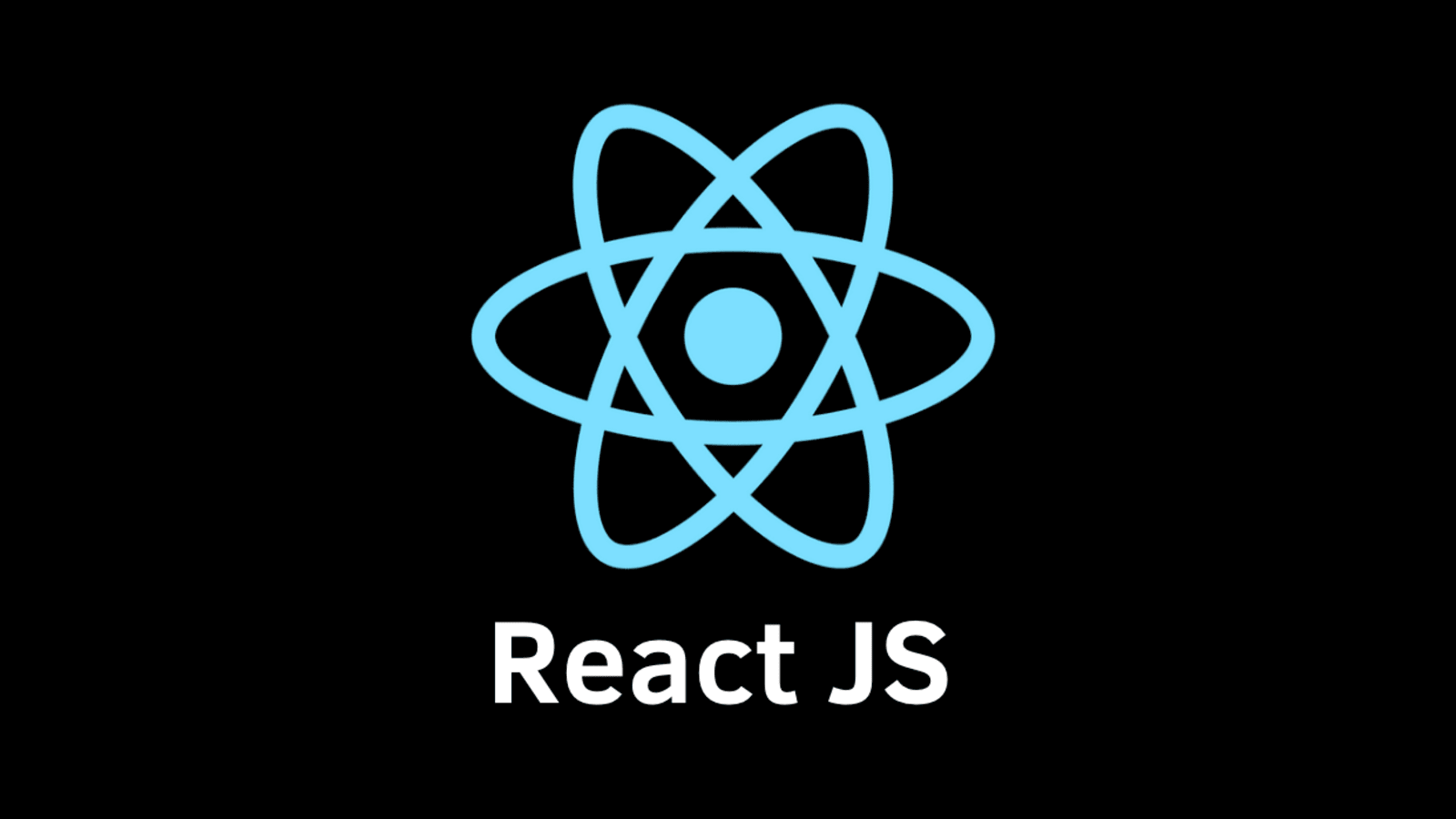
React Fragments, introduced in React 16.2, are a powerful and often underutilized feature that allows developers to group multiple elements without introducing unnecessary DOM nodes. Fragments play a crucial role in optimizing component structure and improving the overall performance of React applications. In this blog tutorial, we will explore the concept of React Fragments, their benefits, and how to use them effectively in your projects.
Understanding React Fragments:
What are Fragments?
React Fragments are a lightweight and straightforward concept that allows developers to group multiple elements without the need for a wrapper div or other container elements. Fragments act as invisible containers and do not introduce any extra DOM nodes, resulting in cleaner and more efficient component structures.
The Need for Fragments:
Before the introduction of Fragments, developers often used wrapper divs or other elements to group multiple elements within a component. This approach, however, added unnecessary divs to the DOM tree, which could impact performance and hinder the ability to style components effectively.
Advantages of Using Fragments:
- Reduced DOM Nodes: One of the primary benefits of using Fragments is the reduction in the number of DOM nodes. Without Fragments, when you wrap multiple elements within a container element (e.g., a div), each additional wrapper introduces an extra DOM node. Fragments, however, act as invisible containers and group elements without adding new nodes to the DOM. This optimization results in a lighter DOM tree, leading to improved rendering performance.
- Cleaner Component Structure: Fragments allow you to group elements together without the need for additional wrapper elements. This leads to a cleaner and more concise component structure, making the code easier to read and maintain. With Fragments, you can avoid unnecessary div soup and keep your JSX markup tidy.
- Better Code Organization: Using Fragments enables you to organize your JSX markup more efficiently. Fragments are particularly useful when rendering lists, tables, or other structures that consist of multiple adjacent elements. Instead of creating separate wrapper elements for each item, you can use Fragments to group them together logically.
- No Impact on Styling: Unlike wrapper elements, Fragments don't introduce any additional styling challenges. Since Fragments don't create new DOM nodes, they won't impact your CSS styles or cause unwanted layout changes.
- Improved Performance: The reduced number of DOM nodes and cleaner component structure offered by Fragments result in better performance. By optimizing the DOM tree, React can render your components more efficiently, leading to a smoother user experience, especially in complex or data-heavy applications.
- Conditional Rendering Simplification: Fragments are particularly useful for conditional rendering scenarios. You can use Fragments to conditionally render different sets of elements without introducing unnecessary wrapper elements. This helps keep your conditional rendering logic cleaner and more straightforward.
- Mapping and Iteration Enhancement: Fragments work seamlessly with mapping and iteration over arrays. When rendering a list of elements, using Fragments allows you to avoid creating wrapper elements for each item, making your code more concise and efficient.
- Accessibility Benefits: Fragments improve the accessibility of your application. By using Fragments instead of unnecessary container elements, you reduce the number of extraneous nodes read by screen readers and other assistive technologies, resulting in a more accessible user interface.
- Compatibility and Browser Support: React Fragments are widely supported and work with all modern browsers. They are a stable feature of React, ensuring cross-platform compatibility and consistency in your applications.
Basic Usage of Fragments:
- Using Empty Tags <> </>:
The simplest way to use React Fragments is by using empty tags `<>` and `</>`. Any elements or components wrapped within these tags will be grouped together without adding any additional DOM nodes.
const MyComponent = () => {
return (
<>
<h1>Hello</h1>
<p>Welcome to the world of React Fragments!</p>
</>
);
};
We used React Fragments (<> and </>) as parent containers to group multiple elements together. In this example, we have two elements inside the top-level fragment:
- <h1>Hello</h1>: This is a level-1 heading element displaying the text "Hello".
- <p>Welcome to the world of React Fragments!</p>: This paragraph element displays the text "Welcome to the world of React Fragments!".
When React renders MyComponent, it treats the top-level fragment as an invisible container. Instead of creating a new DOM node for the fragment, React directly includes the child elements (h1 and p) in the parent's DOM node. Therefore, the HTML output will look like this:
<h1>Hello</h1>
<p>Welcome to the world of React Fragments!</p>
- Using React.Fragment:
Alternatively, you can use the `React.Fragment` syntax to achieve the same result.
import React from 'react';
const MyComponent = () => {
return (
<React.Fragment>
<h1>Hello</h1>
<p>Welcome to the world of React Fragments!</p>
</React.Fragment>
);
};
- Key Prop in Fragments:
When mapping over an array of elements within a Fragment, you may need to provide a unique `key` prop to each child element. This ensures efficient element reordering and helps React identify each element correctly.
Fragments and JSX:
- Nesting Elements within Fragments:
Fragments can be nested to group multiple sets of elements within a component.
const MyComponent = () => {
return (
<>
<h1>Header</h1>
<>
<p>Paragraph 1</p>
<p>Paragraph 2</p>
</>
</>
);
};
In this example,
We use React Fragments (<> and </>) as parent containers to group multiple elements together. In this example, we have three elements inside the top-level fragment:
- <h1>Header</h1>: This is a level-1 heading element displaying the text "Header".
- <></> (Nested Fragments): Inside the top-level fragment, we have another fragment with two paragraph elements.
- <p>Paragraph 1</p>: This paragraph element displays the text "Paragraph 1".
- <p>Paragraph 2</p>: This paragraph element displays the text "Paragraph 2".
- Conditional Rendering with Fragments:
Fragments can also be used in conditional rendering scenarios, allowing you to render different sets of elements without introducing additional wrapper elements.
const MyComponent = ({ isLoggedIn }) => {
return (
<>
{isLoggedIn ? (
<p>Welcome, you are logged in!</p>
) : (
<button>Login</button>
)}
</>
);
};
Inside the top-level fragment, we have a ternary conditional expression:
isLoggedIn ? <p>Welcome, you are logged in!</p> : <button>Login</button>;
- If isLoggedIn is true, the expression will render a paragraph element displaying the text "Welcome, you are logged in!".
- If isLoggedIn is false, the expression will render a button element with the label "Login".
In this case, using Fragments ensures that the paragraph or button element is rendered without introducing any extra DOM nodes. If we didn't use Fragments and instead used a regular div as a container, we would end up with an unnecessary wrapper element in the DOM tree. With Fragments, we maintain a cleaner and more efficient DOM structure.
Fragment Performance and Use Cases:
- Reducing DOM Nodes:
React Fragments reduce DOM nodes by providing a way to group multiple elements without introducing an extra wrapper element (e.g., a div). When you use Fragments, they act as invisible containers, effectively grouping the elements without creating a new node in the actual DOM tree. - Improving Performance with Fragments:
Using Fragments is particularly beneficial when rendering lists of elements, such as tables or lists. Wrapping each item with a Fragment reduces the number of unnecessary container elements. - Use Cases for Fragments:
Fragments are valuable in scenarios where you need to group elements or components without introducing extra markup. Common use cases include conditional rendering, mapping over arrays, and returning multiple elements from a component.
Fragment Short Syntax (Experimental):
- Using Fragment Short Syntax:
React offers an experimental short syntax for Fragments using the `<>` and `</>` syntax without importing `React.Fragment`. Note that this syntax may change in future React versions.
const MyComponent = () => {
return (
<>
<h1>Hello</h1>
<p>Welcome to the world of React Fragments!</p>
</>
);
};
- Limitations and Browser Support:
Keep in mind that the Fragment short syntax is an experimental feature and may not be supported in all environments or tools. Be cautious when using it in production applications.
Best Practices and Tips:
- Properly Organizing Fragments:
When using Fragments, ensure that your component's structure remains organized and easy to understand. Avoid nesting too many levels of Fragments, as it may lead to decreased code readability. - Limiting the Use of Fragments:
While Fragments are powerful, refrain from using them excessively. In simple cases where a single wrapper element is sufficient, prefer using a standard div or a semantic element. - Using Fragments in Lists:
Take advantage of Fragments when rendering lists of elements, as it can significantly improve performance by reducing the number of container elements.
In conclusion, React Fragments are a valuable tool for optimizing component structure and improving performance. By using Fragments to group multiple elements without adding extra DOM nodes, developers can create cleaner, more efficient, and maintainable React applications. Whether using the empty tags syntax or `React.Fragment`, Fragments offer a cleaner alternative to wrapper divs and enhance the readability of your code. By following best practices and understanding the use cases for Fragments, you can harness the full potential of this powerful React feature in your projects. Happy coding with React Fragments!