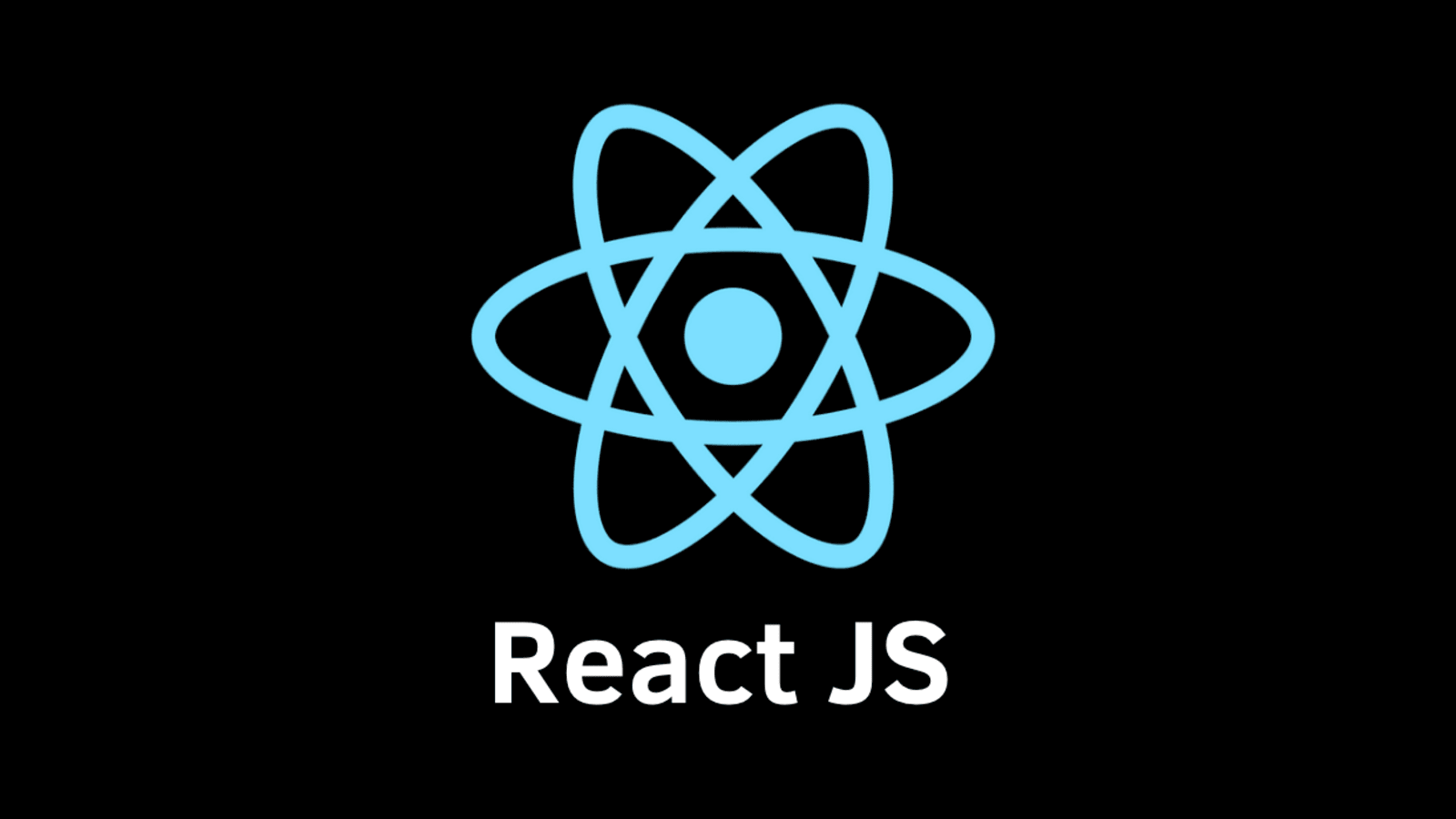
To get started with React, you'll need to set up your development environment. Follow these steps:
Install Node.js:
Node.js is an open-source runtime environment that executes JavaScript code outside of a web browser. It allows developers to build server-side applications using JavaScript, traditionally known as a client-side scripting language. Node.js is built on Chrome's V8 JavaScript engine, which provides high-performance execution of JavaScript code.
Key Features of Node.js:
- Asynchronous, Non-Blocking I/O: Node.js follows an event-driven, non-blocking I/O model, which allows it to handle a large number of concurrent connections efficiently. This makes it well-suited for building scalable and high-performance applications.
- Server-Side Development: With Node.js, developers can build web servers, APIs (Application Programming Interfaces), real-time applications, and other server-side applications. It provides a robust ecosystem of libraries and frameworks, such as Express.js, to simplify server-side development tasks.
- JavaScript Everywhere: Node.js enables developers to use JavaScript both on the client side (in web browsers) and the server side. This unifies the development experience and allows for sharing code between different layers of an application.
- Package Management: Node.js includes npm (Node Package Manager), a powerful package manager and dependency management tool. npm allows developers to easily install, manage, and share reusable JavaScript code packages, making it effortless to leverage third-party libraries and frameworks in Node.js projects.
- Cross-Platform Compatibility: Node.js is designed to be cross-platform, which means it can run on various operating systems such as Windows, macOS, and Linux. This flexibility allows developers to write code once and deploy it on multiple platforms without significant modifications.
Node.js has gained popularity for its performance, scalability, and extensive community support. It has become a go-to choice for building server-side applications and has influenced the rise of modern JavaScript-based full-stack development.
React projects require Node.js, a JavaScript runtime. Download and install the latest version of Node.js from the official website (https://nodejs.org).
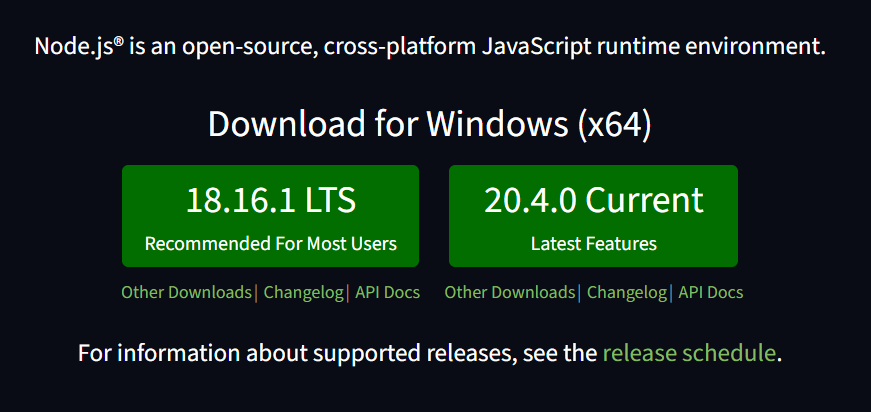
Install a Text Editor:
We will use Visual Studio Code as a text editor in this tutorial. Visual Studio Code (VS Code) is a popular text editor that provides a seamless development experience for various programming languages, including web development with React.
To install VS Code visit the official VS Code website at https://code.visualstudio.com/.
Then click on the "Download" button to download the installer for your operating system.
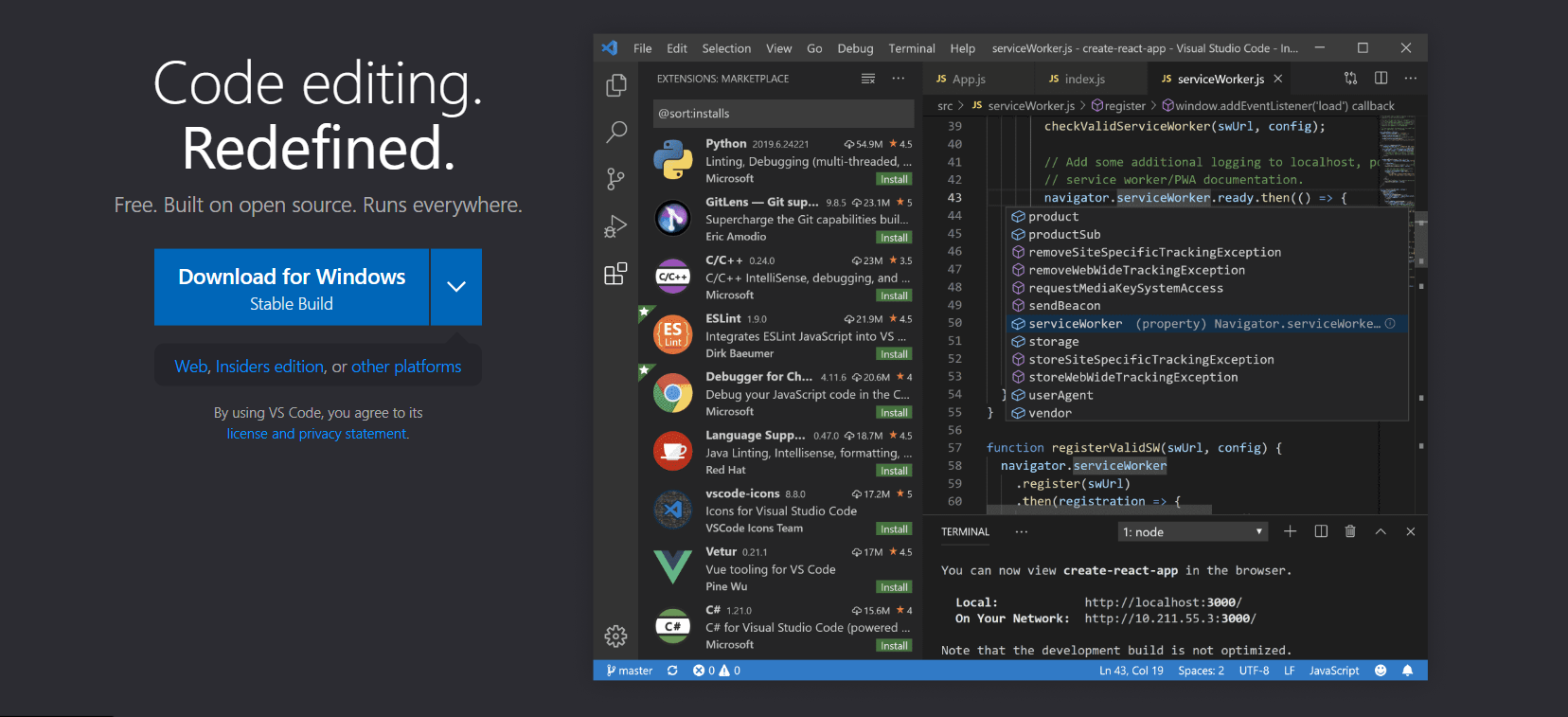
VS code Configuration for React development:
Visual Studio Code (VS Code) offers a wide range of extensions that enhance its functionality and cater to specific programming languages, frameworks, or development workflows. These extensions are used for :
1. Extending Functionality:
- VS Code extensions are add-ons that provide additional features, tools, and language support within the editor.
- Extensions can enhance productivity, simplify complex tasks, and integrate with various development tools and services.
2. Language Support:
- VS Code extensions offer language support for a vast array of programming languages, including JavaScript, TypeScript, Python, Java, HTML, CSS, and more.
- Language-specific extensions provide code syntax highlighting, autocompletion, code formatting, and documentation lookup.
3. Integrated Development Environment (IDE) Features:
- Many extensions transform VS Code into a powerful IDE for specific frameworks, such as React, Vue.js, Angular, or Node.js.
- These extensions provide features like IntelliSense, code snippets, debugging, project scaffolding, and build system integration.
4. Productivity Tools:
- VS Code extensions include productivity tools like code linters, formatters, Git integration, version control tools, code search utilities, and code snippet libraries.
- These tools streamline development workflows, automate tasks, and improve code quality and consistency.
5. Customization and Theming:
- Extensions allow for customizing the appearance of the editor, enabling themes, icons, and personalized styling.
- Customization extensions enhance the overall visual experience and provide a personalized development environment.
6. Community-Driven Ecosystem:
- VS Code has a vast extension ecosystem supported by a large community of developers.
- Extensions are created and maintained by individuals, open-source communities, and technology companies, ensuring a rich and diverse collection of offerings.
7. Easy Installation and Management:
- Installing extensions in VS Code is simple and can be done through the built-in Extension Marketplace.
- The extension management system provides options for enabling, disabling, updating, and uninstalling extensions.
8. Recommended Extensions:
- VS Code provides a list of recommended extensions for specific languages or frameworks.
- These recommendations can be found in the Extensions view, making it convenient to discover popular and useful extensions for your projects.
VS Code extensions are a powerful tool to tailor your development environment to your specific needs. With an extensive library of extensions available, you can customize VS Code to suit your preferred programming languages, frameworks, and development workflows, ultimately enhancing your productivity and making your coding experience more enjoyable.
To configure Visual Studio Code (VS Code) for React.js development, you can follow these steps:
1.Install the Required Extensions:
Open VS Code and go to the Extensions sidebar (Ctrl+Shift+X or Cmd+Shift+X).
Search for and install the following extensions:
1. "ESLint" by Dirk Baeumer: Linting and code quality tool.
2. "Prettier" by Prettier: Code formatter.
3. "Auto Import" by Steoates: Automatic imports for JavaScript/TypeScript.
4. "Reactjs code snippets" by Charalampos Karypidis: Snippets for React development.
2.Configure ESLint:
1. Open your React project in VS Code.
2. Create an .eslintrc or .eslintrc.json file in the project's root directory (or use an existing one).
3. Add the necessary ESLint rules and configurations to this file according to your preferences or project requirements. For example:
{
"extends": ["eslint:recommended", "plugin:react/recommended"],
"plugins": ["react"],
"parserOptions": {
"ecmaVersion": 2021,
"sourceType": "module",
"ecmaFeatures": {
"jsx": true
}
},
"env": {
"browser": true,
"es2021": true
},
"rules": {
// Add your ESLint rules here
}
}
3.Configure Prettier:
1. Create a .prettierrc or .prettierrc.json file in the project's root directory (or use an existing one).
2. Add your Prettier configurations to this file. For example:
{
"semi": true,
"singleQuote": true,
"tabWidth": 2,
"trailingComma": "es5"
}
3. In VS Code, go to "File" > "Preferences" > "Settings" (or use the shortcut Ctrl+, or Cmd+,).
4. Search for "Prettier: Require Config" and enable the option to ensure Prettier uses the project-specific configuration.
5. Configure VS Code Settings:
In VS Code, go to "File" > "Preferences" > "Settings" (or use the shortcut Ctrl+, or Cmd+,).
Add the following settings to your user or workspace settings (in settings.json):
{
"editor.formatOnSave": true,
"editor.defaultFormatter": "esbenp.prettier-vscode",
"editor.codeActionsOnSave": {
"source.fixAll.eslint": true
}
}
These settings enable automatic formatting on save and trigger ESLint to fix any linting errors.
4.Customizations and Additional Settings:
Feel free to explore and customize other VS Code settings to suit your preferences. For example, you can set up custom keybindings, change themes, or configure advanced features like IntelliSense or debugging.
With these configurations, you can enjoy a smooth development experience for React.js projects in VS Code. ESLint will catch code quality issues, Prettier will format your code automatically, and the installed extensions will provide helpful snippets and features specific to React development.
Now we are all set to create a new react project. In the next lesson we will learn how to create a new react.js project.