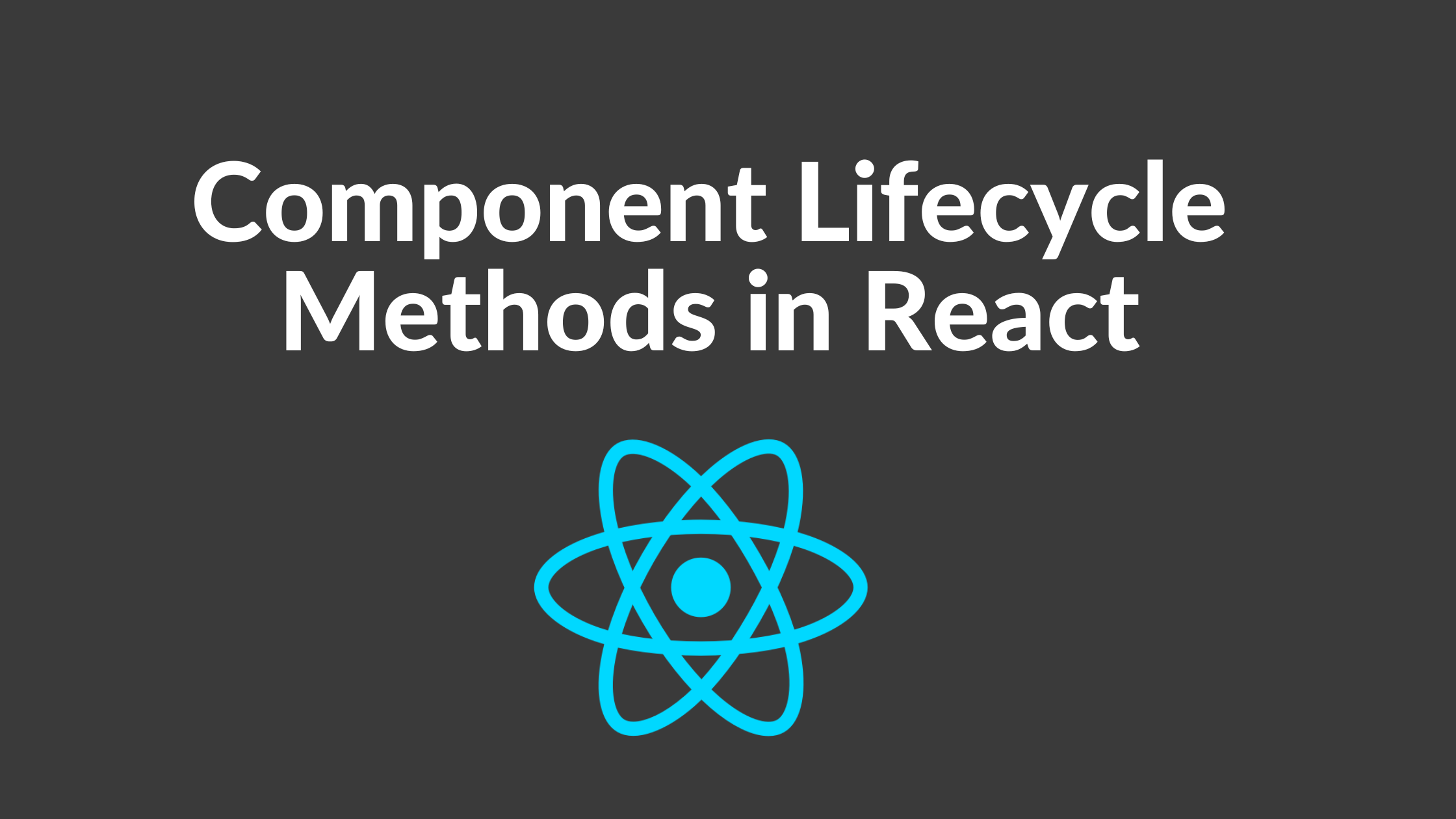
Introduction:
React.js, a popular JavaScript library, revolutionized the way we build user interfaces by introducing a component-based architecture. React components are the building blocks of a React application, encapsulating logic, UI structure, and behavior. In this blog post, we will delve into React components and explore their lifecycle, understanding how they go through different phases from initialization to unmounting. By grasping the intricacies of component lifecycles, developers can effectively manage state, handle side effects, and optimize the performance of their React applications.
What are React Components?
React components are independent, reusable entities that encapsulate specific UI elements and their functionality. They can represent small UI elements like buttons or larger, more complex structures such as forms or entire sections of a web application. Components allow for modular development, making it easier to maintain, test, and reuse code.
In React, there are two main types of components: functional components and class components. Let's explore the characteristics of each type:
Functional Components:
- Functional components are typically defined as JavaScript functions.
- They are simpler and more concise compared to class components.
- Functional components are commonly used for presentational or UI-focused components.
- They receive data through props and return JSX elements to describe the UI structure.
- Functional components do not have their own instance or lifecycle methods.
- With the introduction of React Hooks, functional components can now have state and lifecycle-like behavior using built-in Hooks such as useState, useEffect, and useContext.
- Functional components are recommended for new development and encouraged for their simplicity and ease of testing.
import React from 'react';
const FunctionalComponent = () => {
return (
<div>
<h1>Hello, I am a functional component!</h1>
</div>
);
};
export default FunctionalComponent;
In this example, we defined a functional component called FunctionalComponent. It returns a JSX element containing an <h1> tag with a greeting message.
Class Components:
- Class components are defined as ES6 classes that extend the React.Component class.
- They have their own instance and lifecycle methods.
- Class components were commonly used in earlier versions of React and are still in use in legacy codebases.
- They are suitable for components that require more complex logic, state management, and access to lifecycle methods.
- Class components can define state using this.state and update it using this.setState.
- Lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount are available in class components for handling side effects and managing the component's lifecycle.
- However, class components can lead to more verbose code and potentially slower performance compared to functional components.
import React, { Component } from 'react';
class ClassComponent extends Component {
render() {
return (
<div>
<h1>Hello, I am a class component!</h1>
</div>
);
}
}
export default ClassComponent;
In the class component example, we extend the Component class from the react module. The component contains a render() method that returns the JSX element. The class component provides additional features such as state management and lifecycle methods.
With the introduction of React Hooks, functional components gained the ability to handle state and side effects, making them the recommended choice for most use cases. Hooks provide a more cohesive and concise way of managing component behavior and eliminating the need for class components in many scenarios. However, class components are still supported and may be necessary in certain legacy or specific use cases where Hooks might not be sufficient.
Overall, functional components are preferred for their simplicity, while class components are still used in some cases where advanced features or lifecycle methods are required. The React community encourages using functional components and Hooks as they provide a more modern and streamlined approach to building React applications.
The Lifecycle of React Components:
React components go through a series of lifecycle phases, starting from creation to removal. Each phase offers specific lifecycle methods that developers can utilize to perform actions at different stages of a component's existence.
- Mounting Phase:
- - constructor(): The constructor method is called when a component is being initialized, allowing for setting the initial state and binding event handlers.
- - render(): The render method is responsible for rendering the component's UI structure based on its props and state.
- - componentDidMount(): This method is invoked immediately after the component is rendered on the screen. It is commonly used to perform side effects such as fetching data from an API or subscribing to events.
- Updating Phase:
- - componentDidUpdate(prevProps, prevState): This method is called when the component's props or state change. It allows for handling side effects or updating the component based on the changes.
- - shouldComponentUpdate(nextProps, nextState): This method determines if the component should update or not, based on changes in props or state. It can be used to optimize performance by preventing unnecessary re-renders.
- Unmounting Phase:
- - componentWillUnmount(): This method is invoked just before the component is unmounted and removed from the DOM. It is used to clean up resources, event listeners, or subscriptions created during the component's lifecycle.
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
componentDidMount() {
console.log('Component mounted');
}
componentDidUpdate(prevProps, prevState) {
if (prevState.count !== this.state.count) {
console.log('Count state changed');
}
}
componentWillUnmount() {
console.log('Component will unmount');
}
incrementCount = () => {
this.setState((prevState) => ({
count: prevState.count + 1,
}));
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.incrementCount}>Increment</button>
</div>
);
}
}
export default MyComponent;
In this example, we have a MyComponent class component that demonstrates the usage of different lifecycle methods.
- constructor(): The constructor is called when the component is being initialized. Here, we initialize the state by setting count to 0.
- componentDidMount(): This method is invoked immediately after the component is rendered on the screen. In this example, we log a message to the console indicating that the component has mounted.
- componentDidUpdate(prevProps, prevState): This method is called after the component's state or props change. Here, we compare the previous state's count value with the current state's count value. If they are not equal, we log a message to the console indicating that the count state has changed.
- componentWillUnmount(): This method is invoked just before the component is unmounted and removed from the DOM. In this example, we log a message to the console indicating that the component is about to be unmounted.
- incrementCount(): This is a custom method defined within the component that increments the count state when the button is clicked.
Within the render() method, we display the current count value and provide a button that triggers the incrementCount() method.
When the component mounts, it logs a message to the console. On subsequent updates, the componentDidUpdate() method compares the previous and current state, logging a message if the count value has changed. When the component is about to be unmounted, the componentWillUnmount() method logs a message.
These lifecycle methods allow developers to handle side effects, perform necessary updates, and clean up resources at appropriate stages of a component's lifecycle, ensuring a smooth and controlled operation of the React application.
React Hooks and Lifecycle Methods:
With the introduction of React Hooks, there are alternative approaches to handle component lifecycles. Hooks such as useEffect() and useLayoutEffect() provide a unified way to manage side effects and replace some of the traditional lifecycle methods.
Best Practices and Considerations:
- Understand the purpose of each lifecycle method and utilize them appropriately.
- Be mindful of the performance implications of lifecycle methods and optimize them if needed.
- Consider using React Hooks for simpler and more concise lifecycle management.
- Avoid excessive reliance on lifecycle methods, and favor functional and declarative approaches when possible.
React components and their lifecycle play a crucial role in building robust and interactive user interfaces. By understanding the various phases of a component's lifecycle, developers gain control over state management, side effects, and optimization. Whether it's initializing state, performing asynchronous operations, or cleaning up resources, the lifecycle methods provide a structured approach to handle these scenarios. With React's component-based architecture and lifecycle management, developers can create scalable and maintainable applications with ease.