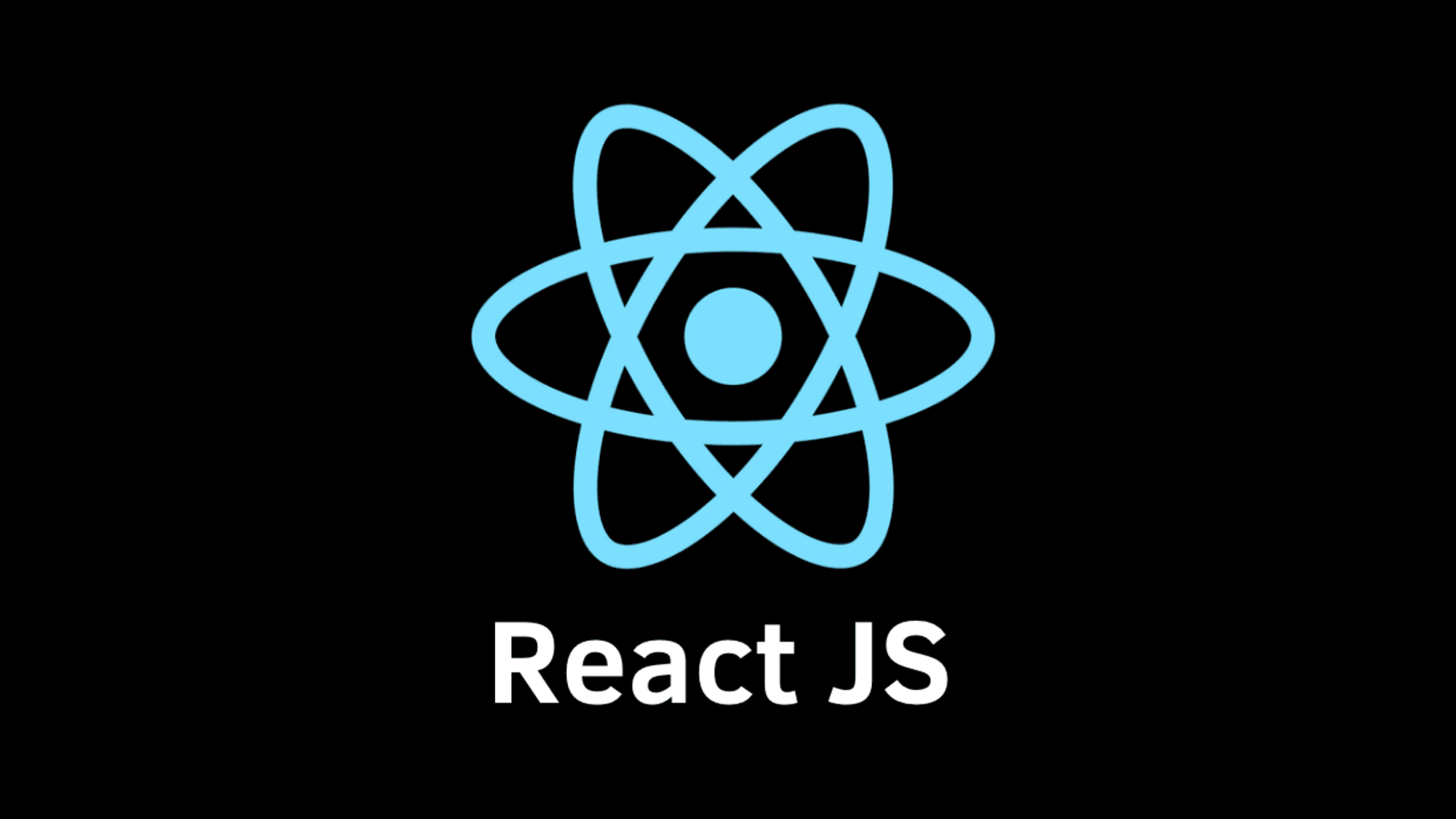
Query parameters are a powerful feature in web development that allow you to pass and retrieve data as key-value pairs in the URL. In React Router, query parameters provide a way to send and receive additional information to and from your components. This tutorial will walk you through the concept of query parameters in React Router and demonstrate how to use them effectively in your applications.
Understanding query parameters
Query parameters are key-value pairs that are added to the end of a URL to provide additional information or data to a web page. They are commonly used to modify or customize the content that is displayed on a page, without the need to navigate to a different URL. Query parameters are separated from the base URL by a question mark (`?`) and are separated from each other by an ampersand (`&`).
Here's the basic structure of a URL with query parameters:
https://example.com/path/to/page?param1=value1¶m2=value2
In this URL, `param1` and `param2` are the query parameters, and `value1` and `value2` are their respective values.
Query parameters can be used for various purposes, such as:
- Filtering and Searching: In a search page, query parameters can be used to pass search terms and filters to the server so that it can return relevant results.
- Pagination: Query parameters can be used to specify the page number and the number of items per page in a paginated list of content.
- Sorting: Query parameters can indicate how the content should be sorted, such as by date, popularity, or relevance.
- User Preferences: Query parameters can be used to customize a user's experience on a website, such as choosing a language or theme.
- Tracking: Query parameters can be used to track user interactions, such as tracking referral sources or campaign information.
In React Router, query parameters can be accessed and manipulated using the `useLocation` hook or the `location` prop. This allows React components to dynamically respond to changes in query parameters and update their rendering based on the provided values.
For example, if you have a URL like `https://example.com/search?q=react`, the query parameter `q` is used to pass the search query "react" to the page. The page can then use this query parameter to fetch search results or display relevant content related to the search term "react."
Query parameters are a versatile tool for building dynamic and interactive web applications, allowing you to create personalized and customizable user experiences by modifying the behavior and content of pages based on the information passed in the URL.
Advantages of using query parameters:
Using query parameters in web applications offers several advantages that enhance user experience, improve data handling, and simplify URL management. Here are some key advantages of using query parameters:
- Statelessness: Query parameters allow you to pass information between different pages without relying on server-side state. This makes your application more stateless, reducing the need for server-side storage and increasing scalability.
- Bookmarkable URLs: Query parameters enable users to bookmark specific pages with customized settings or filters. When users return to these URLs, they see the same content or settings, improving usability.
- Shareable Links: Users can easily share links with others that include specific settings, filters, or data. This is particularly useful for sharing search results, product configurations, or customized views.
- Dynamic Filtering and Sorting: Query parameters facilitate dynamic filtering, sorting, and pagination of content. Users can adjust parameters in the URL to quickly change how content is displayed without reloading the entire page.
- Customized User Experiences: Query parameters allow you to create personalized user experiences by tailoring content based on user preferences, settings, or selections.
- Backward Compatibility: When adding new features or parameters to your application, you can maintain backward compatibility by gracefully handling URLs without the new parameters.
- SEO Benefits: Search engines can index URLs with different query parameters, allowing users to find specific content more easily through search queries.
- Data Sharing: Query parameters can be used to pass small amounts of data between pages. This is especially useful when transitioning between steps in a multi-step process.
- A/B Testing: Query parameters can be used to implement A/B testing by passing different configurations to users and measuring their responses.
- Tracking and Analytics: Query parameters are commonly used to track user interactions, monitor campaign performance, and gather analytics data.
- Caching: URLs with different query parameters can be cached separately, optimizing performance by delivering appropriate content from cache.
- Flexible URL Structures: With query parameters, you can keep a consistent base URL while dynamically modifying the behavior of the page.
- Reusable Components: Query parameters allow you to reuse components for different purposes by passing relevant data as parameters.
- API Integration: Many APIs accept query parameters to customize data retrieval, filtering, and sorting. Your application can align its behavior with external APIs by using the same query parameter structure.
Example:
Step 1: Create Components
In this example, we'll create two components: Home and SearchResults. The SearchResults component will display search results based on query parameters.
Create a new file named Home.js inside the src folder:
// Home.js
import React from 'react';
import { Link } from 'react-router-dom';
const Home = () => {
return (
<div>
<h1>Welcome to the Home Page</h1>
<Link to="/search?query=react">Search for React</Link>
</div>
);
};
export default Home;
Create a new file named SearchResults.js inside the src folder:
// SearchResults.js
import React from 'react';
import { useLocation } from 'react-router-dom';
const SearchResults = () => {
const location = useLocation();
const queryParams = new URLSearchParams(location.search);
const query = queryParams.get('query');
return (
<div>
<h1>Search Results for: {query}</h1>
</div>
);
};
export default SearchResults;
Step 2: Set Up App Routing
Now, let's configure the routing in the App.js file using React Router DOM and implement query parameters.
Open the src/App.js file and replace its contents with the following code:
// App.js
import React from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
import Home from './Home';
import SearchResults from './SearchResults';
const App = () => {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/search" component={SearchResults} />
</Switch>
</Router>
);
};
export default App;
Using Query Parameters
In this example, we're using the Link component from React Router to create a link to the SearchResults component with a query parameter.
When a user clicks the "Search for React" link, they will be redirected to /search?query=react.
The SearchResults component will extract the value of the query parameter from the URL and display "Search Results for: react".
Best practices:
Utilizing query parameters effectively requires adherence to best practices to ensure a smooth and user-friendly experience in your web application. Here are some best practices to consider when working with query parameters:
1. Keep URLs Readable and Understandable:
- Use descriptive query parameter names that are easily understandable.
- Avoid using cryptic or abbreviated names that could confuse users.
2. Limit the Number of Parameters:
- Keep the number of query parameters to a reasonable minimum.
- Too many parameters can clutter the URL and make it difficult to manage.
3. Encode Values:
- Always encode query parameter values using URL encoding to handle special characters or spaces properly.
- Use functions like encodeURIComponent in JavaScript to encode values before appending them to the URL.
4. Default Values:
- Provide default values for query parameters whenever possible.
- This ensures that users see meaningful content even if they don't explicitly set the parameters.
5. Validation and Sanitization:
- Validate and sanitize query parameters on the server-side to prevent security vulnerabilities and unexpected behavior.
- Avoid relying solely on client-side validation, as it can be bypassed.
6. Use Descriptive Values:
- Use human-readable values in query parameters to enhance user understanding and readability.
7. Consistent Casing:
- Maintain consistent casing (e.g., lowercase, camelCase) for query parameter names across your application.
8. Provide Clear Feedback:
- If a query parameter is invalid or missing, provide clear error messages to users.
- Guide users on how to correct or complete the parameter.
9. Avoid Sensitive Data:
- Avoid passing sensitive information, such as passwords or private data, in query parameters.
- Use other methods like headers or request bodies for such data.
10. Test Edge Cases:
- Test your application with different parameter combinations, including edge cases, to ensure it behaves as expected.
11. Document Parameters:
- Document the purpose, format, and possible values of each query parameter in your codebase's documentation.
12. Combine with Route Parameters:
- Consider combining query parameters with route parameters to create more complex and dynamic URLs.
13. Clean Up Unused Parameters:
- Remove or clean up query parameters that are no longer needed in your application.
14. Implement Pagination:
- If using query parameters for pagination, consider including information about the total number of items or pages.
15. Useful Defaults:
- Set default values for query parameters that make sense for your application's context.
16. URL Length:
- Be mindful of URL length limitations imposed by browsers or web servers. Extremely long URLs can lead to issues.
17. Consistent User Experience:
- Maintain a consistent user experience when users navigate back and forth using browser history buttons.
Query parameters in React Router provide a flexible and convenient way to pass and retrieve data via the URL. By following the steps outlined in this tutorial, you've learned how to implement query parameters in your React application. Experiment with different query parameter values and integrate query parameters into your own projects to create more interactive and data-driven user experiences.