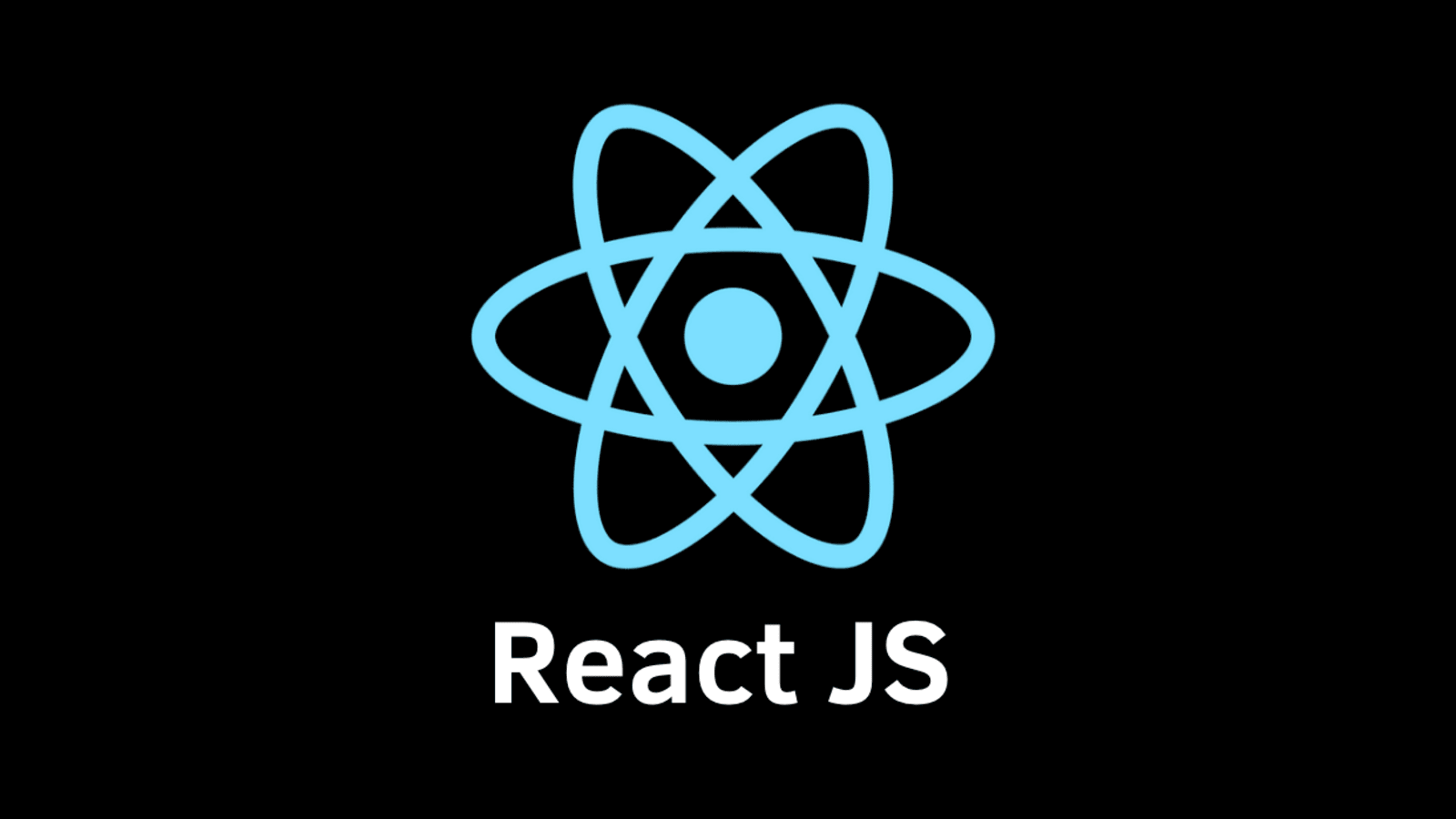
Both props and state play crucial roles in passing and managing data within components, but they have distinct purposes and use cases.
In this tutorial, we will delve into the core concepts of props and state in React, exploring their unique purposes, use cases, and when to use one over the other. Whether you're a beginner looking to strengthen your understanding of React fundamentals or an intermediate developer seeking to refine your component development skills, this guide has got you covered.
Understanding React Props:
What are Props in React?
In React, props (short for "properties") are a mechanism for passing data from a parent component to a child component. They are a way to provide configuration and data to components, allowing them to be dynamic and reusable. Props are immutable, meaning they cannot be changed within the child component.
How to Pass Props to Components?
To pass props to a component, you simply include the props as attributes when using the component in the parent component's JSX. For example:
// ParentComponent.js
import React from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = () => {
const name = 'John Doe';
return (
<div>
<ChildComponent name={name} />
</div>
);
};
// ChildComponent.js
import React from 'react';
const ChildComponent = (props) => {
return (
<div>
<h1>Hello, {props.name}!</h1>
</div>
);
};
export default ChildComponent;
In this example, the `ParentComponent` passes the `name` prop to the `ChildComponent`, which receives it as `props` and displays the name in the output.
Props Validation with PropTypes:
To ensure that the correct props are passed to a component and to provide documentation for developers, it is recommended to use PropTypes for prop validation. PropTypes help catch bugs and provide warnings when the wrong type of props is supplied.
To use PropTypes, you need to import it from the `prop-types` library and define the expected types for each prop:
// ChildComponent.js
import React from 'react';
import PropTypes from 'prop-types';
const ChildComponent = (props) => {
return (
<div>
<h1>Hello, {props.name}!</h1>
</div>
);
};
ChildComponent.propTypes = {
name: PropTypes.string.isRequired,
};
export default ChildComponent;
In this example, we define that the `name` prop should be a required string. If the `name` prop is not provided or if it is of the wrong type, React will display a warning in the console.
Advantages of Props:
- Component Communication: Props enable communication between parent and child components. They allow data to flow from higher-level components (parents) to lower-level components (children), facilitating a clear data flow in the application.
- Reusability: Props promote component reusability. By passing different data through props, you can reuse the same component in various parts of the application, reducing code duplication and improving maintainability.
- Predictability: Since props are immutable, they provide predictability and help prevent unintended side effects in components. This immutability ensures that the data received by a component remains consistent throughout its lifecycle.
- Easy Testing: Testing components that rely on props is straightforward since you can easily provide different sets of props to check how the component behaves with different data.
- Clear Component API: Props serve as a clear and well-documented API for a component. By defining the prop types and their requirements, it becomes evident what data a component expects to receive.
Understanding React State:
What is State in React?
In contrast to props, state is used to manage data within a component itself. State is mutable, and when the state changes, React automatically re-renders the component to reflect the updated data in the UI. Unlike props, state is specific to the component and not intended to be shared with other components directly.
How to Use State in Components?
To use state in a component, you need to use React's `useState` hook (for functional components) or the `this.state` object (for class components) to initialize and manage the state.
For functional components:
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
const decrement = () => {
setCount(count - 1);
};
return (
<div>
<h1>Count: {count}</h1>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
For class components:
import React, { Component } from 'react';
class Counter extends Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
increment = () => {
this.setState({ count: this.state.count + 1 });
};
decrement = () => {
this.setState({ count: this.state.count - 1 });
};
render() {
return (
<div>
<h1>Count: {this.state.count}</h1>
<button onClick={this.increment}>Increment</button>
<button onClick={this.decrement}>Decrement</button>
</div>
);
}
}
In both examples, the component manages its own state (`count` in this case) and updates it using the `setState` function.
Advantages of State:
- Dynamic User Interfaces: State enables dynamic user interfaces that respond to user interactions. By updating state, components can re-render and display the latest data in real-time.
- Internal Data Management: State allows components to manage their own internal data without relying on external sources. This encapsulation improves component modularity and makes it easier to reason about component behavior.
- Form Handling: State is commonly used to handle form input data. As users enter data into a form, the component's state can be updated, providing instant feedback to users and simplifying form validation.
- Interactive Components: State is crucial for building interactive components such as toggles, dropdowns, and modals. By tracking the state of these components, their behavior can be controlled based on user interactions.
- Asynchronous Updates: State updates in React are asynchronous, meaning multiple updates can be batched together for better performance. This optimization prevents unnecessary re-renders and ensures smooth user experiences.
Differences:
1. Definition and Purpose:
- Props: Props (short for "properties") are used to pass data from parent components to child components. They allow data to be transferred down the component tree and enable communication between components.
- State: State is used to manage data within a component itself. It represents the current state of the component and allows the component to keep track of data that can change over time.
2. Scope:
- Props: Props are read-only and can only be passed from parent components to their children. They are intended for one-way data flow and cannot be modified directly by the child component receiving them.
- State: State is mutable and belongs to the component where it is defined. Each component maintains its own state, and when the state changes, React automatically re-renders the component to reflect the updated data.
3. Ownership and Management:
- Props: Props are owned and managed by the parent component. The parent component provides props to its child components, and those child components consume the data passed as props.
- State: State is owned and managed by the component itself. The component initializes its state and updates it using useState hook (for functional components) or this.state and this.setState (for class components).
4. Immutability:
- Props: Props are immutable, meaning they cannot be changed or modified by the child component receiving them. A child component can only use the props passed to it, but it cannot modify the props directly.
- State: State is mutable, and the component can update its state using the setState function (for class components) or the state update function returned by useState hook (for functional components).
5. Data Flow:
- Props: Data flow with props is unidirectional, moving from parent to child components. Changes in props occur in the parent component, and those changes are passed down to child components for rendering.
- State: State data is also unidirectional, but it flows within the same component. When the state changes, React re-renders the component and its child components, if any, with the updated state.
6. Use Cases:
- Props: Props are ideal for passing data and configuration from parent components to child components. They are useful for making components reusable and for customizing component behavior based on parent's requirements.
- State: State is used for managing component-specific data that can change over time, such as form input values, toggles, and user interactions. It enables dynamic behavior in components.
7. Communication:
- Props: Props enable communication between components by allowing data to be passed down the component tree. Parent components can send data to child components using props.
- State: State is used for internal communication within a single component. It allows the component to maintain its own data and update it based on user interactions or other events.
When to Use Props or State:
- Use props when you need to pass data from a parent component to a child component, especially when you want to make the child component reusable and independent of its parent.
- Use state when you need to manage and handle internal component data that can change over time based on user interactions or other events.
- Avoid using state for data that can be derived from props. Instead, derive such data directly from the props in the component's render method.
- Strive for a unidirectional data flow, passing data down through props and handling changes through callbacks. Avoid using props to pass data back up to parent components; use callbacks and state lifting for that purpose.
In summary, Props and state are both essential tools for building dynamic and interactive React applications. Props facilitate component communication and reusability, while state enables dynamic behavior and internal data management. By understanding when to use props and state appropriately, you can create well-structured, maintainable, and efficient React components.