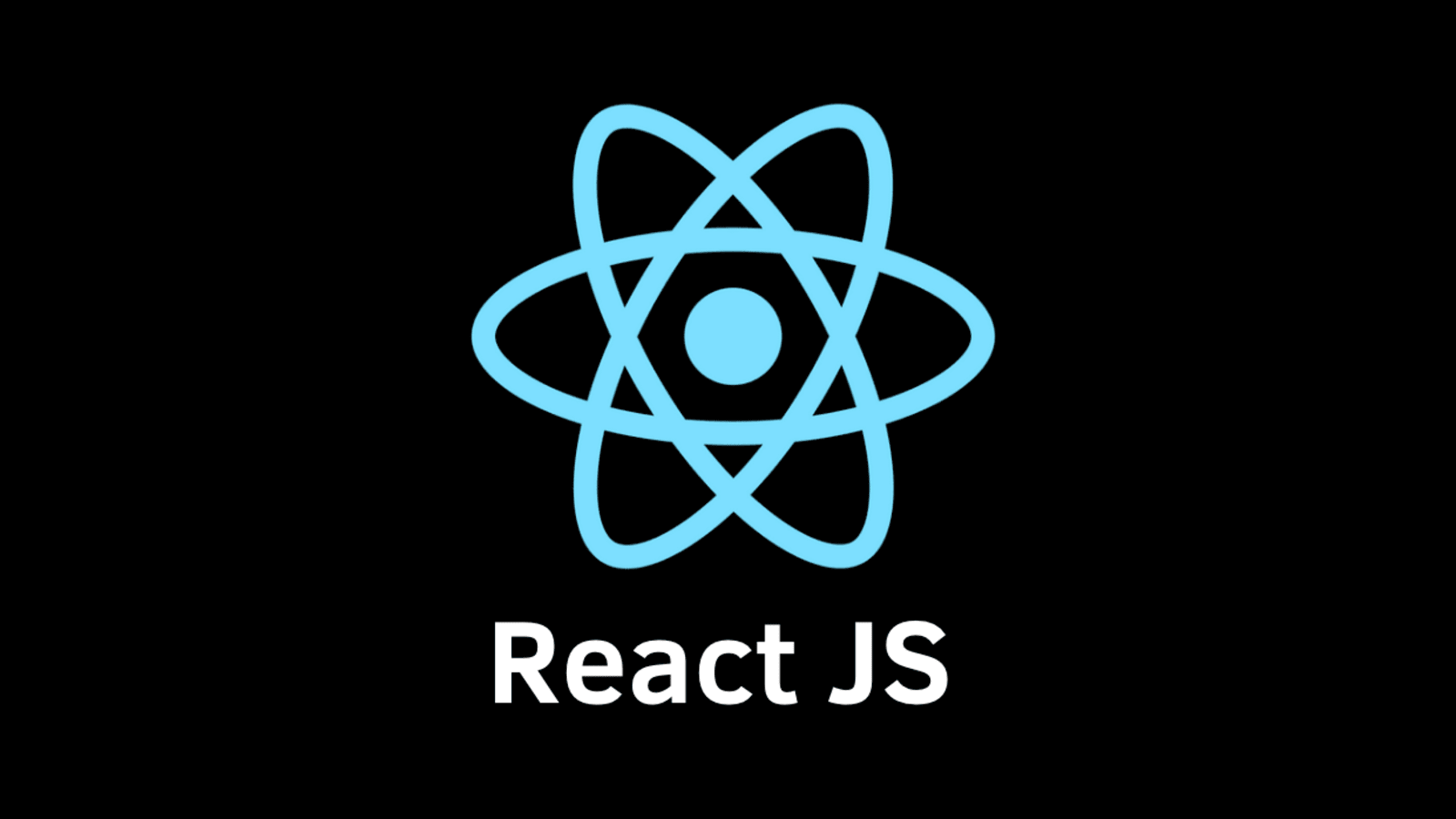
In our previous blog post, we explored the art of creating custom reusable components in React. We learned how to design versatile, flexible, and efficient components that can be utilized across various projects, fostering a more consistent and scalable codebase. Now, armed with a collection of powerful components, it's time to bring them to life by placing them on the main view of our React application.
Why Use Custom Reusable Components:
Before we dive into the practical aspects, let's briefly recap the importance of custom reusable components. By creating reusable components, we can streamline our development process, reduce redundancy, and save valuable development time. Furthermore, these components promote a modular architecture, making our codebase more maintainable and easier to navigate. Leveraging custom reusable components allows developers to focus on building new features and enhancing user experiences, rather than reinventing the wheel.
Integrating Custom Reusable Components:
1. Setting Up the Main View:
To begin, we need a main view to serve as the foundation of our application. This can be a top-level component, such as a Dashboard, Home, or Landing page. The main view acts as the canvas on which we'll place our custom reusable components.
2. Importing Custom Reusable Components:
With our main view ready, we can import our custom reusable components into the main view component file. These components could include buttons, cards, modals, form inputs, and more, which we previously designed following best practices.
3. Component Placement and Configuration:
Next, we arrange and configure the custom reusable components within the main view. This involves specifying the appropriate props for each component, customizing their appearance, and deciding on the layout.
4. Prop Drilling and Context API:
In some cases, we may need to pass data and functions between nested components. This can be achieved through prop drilling or by using React's Context API to manage global state and share data without passing it explicitly through props.
5. Conditional Rendering:
Depending on the application's logic, certain custom reusable components may only appear under specific conditions. Leveraging conditional rendering in React allows us to control when and where these components should be displayed.
6. Event Handling:
Custom reusable components often require interactivity, such as handling button clicks, form submissions, or user interactions. We ensure that these events are properly handled, and the necessary actions are executed.
Now, if we look at the main.jsx file:
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App.jsx';
import './index.css';
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<App />
</React.StrictMode>,
);
It renders the <App/> component. It is the main component of our project. The App component is a functional component that returns JSX elements to define the UI of the application.
function App() {
return (
<>
<h1>Hello World!</h1>
</>
)
}
export default App
In the App.jsx we see it renders a h1 tag. Now what if we want to render a custom component in our main component (i.e. in the App.jsx)
Firstly, we have to import the custom component then the component be placed in the main view. Previously we had created a custom button component which is placed in the /src/components directory in our project.
import React from 'react';
const Button = ({ text, color, size, onClick }) => {
const buttonStyle = {
backgroundColor: color,
fontSize: size === 'large' ? '24px' : '16px',
padding: '10px 20px',
borderRadius: '5px',
color: '#fff',
cursor: 'pointer',
};
return (
<button style={buttonStyle} onClick={onClick}>
{text}
</button>
);
};
export default Button;
Now, in the main view(App.jsx) we have to import this component and render it providing its props.
import Button from './components/Button';
function App() {
const clickHandler=()=>{
alert("Custom button clicked!")
}
return (
<>
<h1>Hello World!</h1>
<Button text="Custom button" color="#31506F" size="large" onClick={clickHandler}/>
</>
)
}
export default App
We have placed the custom button component in our main view(App.jsx). The Button component has text, color, size and onClick props. We created a function named clickHandler(). Which simply generates an alert saying “Custom button clicked!”. We provided this function in the onClick props. So that, when the button is clicked the alert be shown.
Benefits of Placing Custom Reusable Components:
- Code Reusability: By placing our custom reusable components on the main view, we can reuse the same components across multiple parts of the application, reducing code duplication and improving maintainability.
- Consistency and Branding: Integrating custom reusable components ensures a consistent and cohesive user interface throughout the application, enhancing the user experience and promoting a strong brand identity.
- Faster Development: Leveraging custom reusable components accelerates the development process, as developers can quickly assemble complex UIs without having to build each component from scratch.
- Scalability: As our application grows, custom reusable components allow us to easily scale and extend its features, keeping our codebase organized and manageable.
When placing custom reusable components on the main view in a React application, following best practices is essential to ensure efficiency, maintainability, and a seamless user experience. Here are some best practices to consider:
- Single Responsibility Principle (SRP): Ensure that each custom reusable component has a single responsibility and is focused on a specific task. This helps maintain a clean and modular codebase, making it easier to manage and understand.
- Props and Composition: Design custom components with a flexible API using props. Allow users to customize the component's behavior and appearance through props. Utilize composition to combine smaller components into more complex ones, promoting code reuse.
- Conditional Rendering: Use conditional rendering to control when and where custom components are displayed on the main view. This allows for a dynamic and interactive user interface based on the application's logic.
- Default Props and Prop Types: Specify default props for optional values and use prop types to enforce prop validation. This ensures that the correct data types are passed to the components, reducing the risk of bugs and errors.
- State Management: Avoid managing complex state within reusable components. Instead, rely on props to pass data down and use callback functions to communicate changes back to the parent components.
- Accessibility: Ensure that custom components are accessible to all users, including those with disabilities. Use semantic HTML elements, add appropriate ARIA attributes, and test components with screen readers and other assistive technologies.
- Event Handling: Implement proper event handling within custom components to ensure interactivity. Use event listeners and state updates appropriately to maintain a consistent user experience.
- Testing: Write comprehensive unit tests for custom reusable components to ensure they work as expected and remain stable when changes are made. Use testing libraries like Jest and Enzyme for thorough test coverage.
- Documentation: Provide clear and detailed documentation for each custom component. Describe the purpose of the component, available props, and usage examples. This enables other developers to use the components effectively.
- Versioning and Semantic Versioning: If you plan to publish your custom components as npm packages, follow semantic versioning (SemVer) to manage changes and updates. Increment the version number appropriately when making breaking changes.
- CSS-in-JS or Styled Components: Consider using CSS-in-JS libraries or styled components to keep the styles encapsulated within the custom components. This prevents CSS conflicts and allows for easy customization of styles.
- Performance Optimization: Optimize the performance of custom components by avoiding unnecessary re-renders, using React.memo or shouldComponentUpdate, and leveraging the useMemo and useCallback hooks for expensive computations and event handlers.
- Compatibility: Ensure that custom components are compatible with different browsers to deliver a consistent experience across various environments.
- Cross-Platform Responsiveness: Design custom components to be responsive across different devices and screen sizes, ensuring a seamless user experience on both desktop and mobile devices.
By adhering to these best practices, you can successfully place custom reusable components on the main view of your React application, creating a robust, scalable, and maintainable user interface. This approach not only enhances development efficiency but also improves the overall user experience, leading to a more successful and user-friendly application.
Placing custom reusable components on the main view of our React application is a powerful strategy to enhance development efficiency, maintainability, and user experience. By creating versatile components with a single responsibility and adhering to best practices, we empower ourselves to build sophisticated user interfaces with ease.
With the tools and knowledge acquired from our previous blog post and the practical insights shared in this post, you're well-equipped to take your React applications to the next level. Embrace the power of custom reusable components and witness the transformative impact on your development workflow and the quality of your software products. Happy coding!