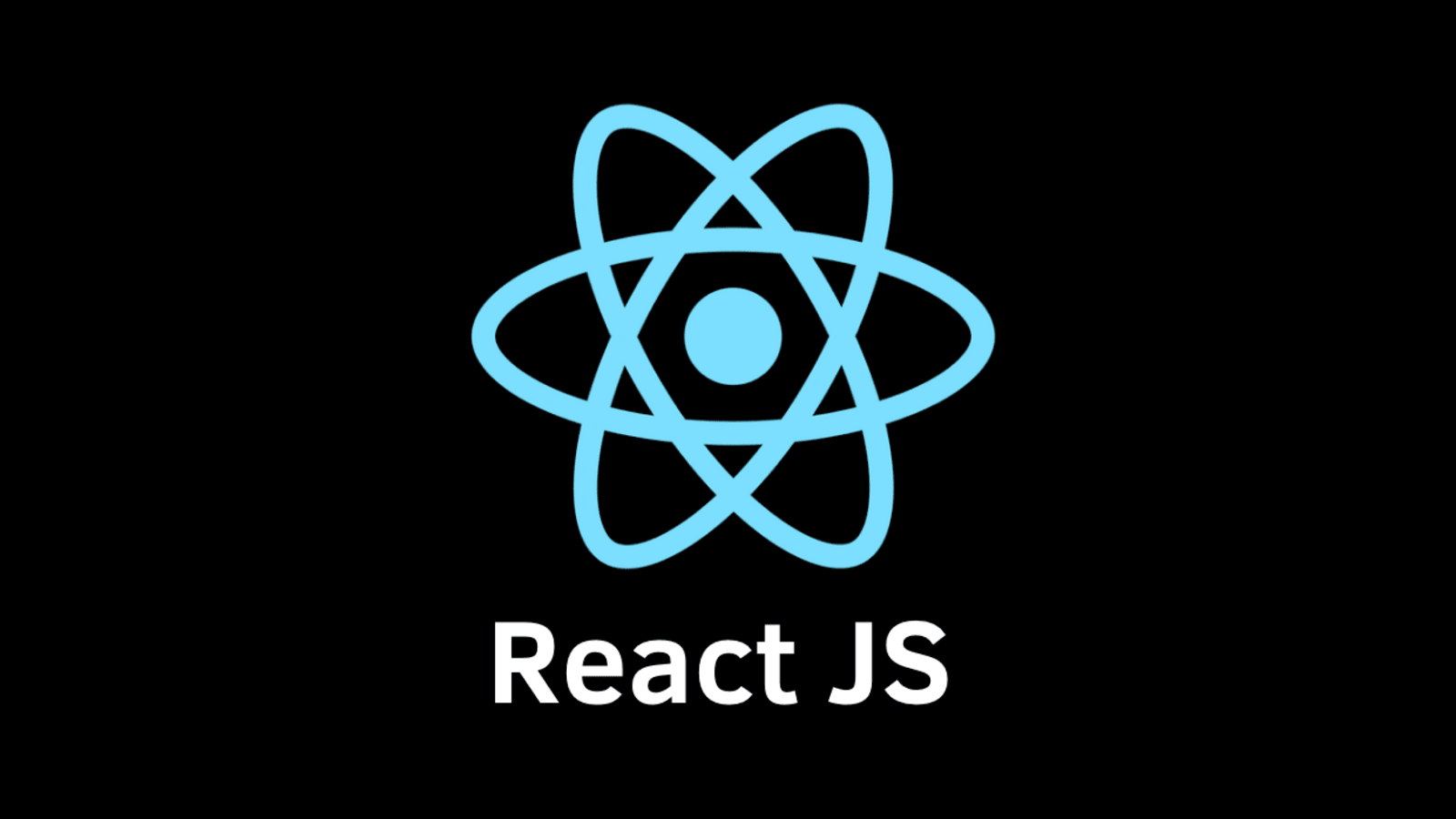
Routing is a critical aspect of building dynamic and complex web applications. With the introduction of React Router v6, managing nested routes has become even more streamlined and intuitive. Nested routes allow you to create a more organized and structured navigation system, enabling you to build sophisticated user interfaces with ease.
In this tutorial, we will delve into the concept of nested routes using React Router v6. We'll guide you through setting up a sample project, explain the syntax of nested routes, and provide step-by-step instructions to implement nested routing in your React applications.
What is nested routing?
Nested routing in React Router DOM refers to the practice of defining routes within other routes, creating a hierarchical structure for handling different components and views based on the URL. This approach allows you to encapsulate components and their associated routes within a parent component, enabling you to build more complex and organized user interfaces.
Characteristics of Nested Routing:
- Hierarchical Structure: Nested routing introduces a hierarchy of routes. Parent components can have child components with their own routes, creating a tree-like structure.
- Component Composition: Each level of nesting can correspond to a different level of component composition. This makes it easier to manage and maintain code by breaking down complex UIs into smaller, reusable components.
- Isolation: Nested routes provide isolation between different sections of your application. This separation of concerns makes it easier to understand, modify, and debug individual parts of your app.
- Dynamic UI: Nested routing enables the dynamic loading of components based on the URL. As users navigate different routes, the corresponding components are rendered without full page reloads.
- URL Parameters: Nested routes can also have their own URL parameters that can be used to customize and filter the content within those routes.
- Code Splitting: Nested routes facilitate code splitting, allowing you to load only the necessary code for a specific route, improving initial page load performance.
- Layouts and Templates: Parent components can act as layouts or templates for their child routes, providing consistent styling and structure.
- Context Sharing: Data and context can be shared between parent and child components, allowing you to pass down props, state, or other data.
- Conditional Rendering: You can conditionally render different components and UI elements based on the current route, enhancing user experience and interactivity.
Benefits of Nested Routing:
- Modularity: Components and their routes are modular, making the codebase easier to maintain and scale.
- Organization: Nested routing offers a more organized approach to handling different sections of your app.
- Reusability: Components can be reused across different parts of your app, enhancing code efficiency.
- Flexibility: You can easily add, remove, or modify sections of your app without affecting unrelated parts.
- User Experience: Dynamic loading of components and content provides a smoother user experience.
- SEO Optimization: Nested routing can improve SEO as search engines can crawl different pages of your app.
- Clear Separation: Each nested route can have its own logic and state, providing clear separation of concerns.
Example:
Create Components
In this example, we'll create three components: AuthLayout, Login, and Dashboard. The AuthLayout component will serve as the main container for our nested routes, and the Login and Dashboard components will represent sub-sections.
Create a new file named AuthLayout.js inside the src folder:
// AuthLayout.js
import React from 'react';
import { Outlet } from 'react-router-dom';
const AuthLayout = () => {
return (
<div>
<h1>Auth Layout</h1>
<Outlet />
</div>
);
};
export default AuthLayout;
Create two new files named Login.jsx and Dashboard.jsx inside the src folder:
// Login.js
import React from 'react';
const Login = () => {
return <h2>Login Page</h2>;
};
export default Login;
// Dashboard.js
import React from 'react';
const Dashboard = () => {
return <h2>Dashboard Page</h2>;
};
export default Dashboard;
Set Up App Routing
Now, let's configure the routing in the App.jsx file using React Router v6's nested route syntax.
Open the src/App.jsx file and replace its contents with the following code:
// App.jsx
import React from 'react';
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom';
import AuthLayout from './AuthLayout';
import Login from './Login';
import Dashboard from './Dashboard';
const App = () => {
return (
<Router>
<Routes>
<Route path="/" element={<AuthLayout />}>
<Route path="login" element={<Login />} />
<Route path="dashboard" element={<Dashboard />} />
</Route>
</Routes>
</Router>
);
};
export default App;
Implementing Nested Routes
In the provided example, we've set up nested routes inside the AuthLayout component. When the user accesses different paths, the corresponding components will be displayed within the AuthLayout container.
Step 1: Understanding Nested Routes
In the code snippet above, we're using the Routes and Route components from React Router v6 to define our nested routes.
The Routes component wraps all the nested Route components. It ensures that only the first matching route is rendered, similar to a Switch component in previous versions of React Router.
Inside the Routes component, we define the parent route using the Route component with the element prop set to <AuthLayout />. This means that whenever the URL matches the parent route's path (i.e., /), the AuthLayout component will be rendered.
Step 2: Using Nested Routes
In the AuthLayout component, we use the Outlet component from React Router v6 to indicate where the nested routes should be rendered. The Outlet acts as a placeholder for the content of the nested routes.
// AuthLayout.js
import React from 'react';
import { Outlet } from 'react-router-dom';
const AuthLayout = () => {
return (
<div>
<h1>Auth Layout</h1>
<Outlet />
</div>
);
};
export default AuthLayout;
Best Practices:
Implementing nested routing in your React application comes with certain best practices to ensure a clean and maintainable codebase. Here are some recommended practices:
- Plan Your Route Hierarchy: Before implementing nested routes, plan out your route hierarchy and component structure. This will help you visualize how different components will be organized within parent and child routes.
- Use Meaningful Component Names: Choose clear and meaningful names for your components and routes. This makes it easier for you and other developers to understand the purpose of each component.
- Separate Concerns: Divide your components based on their responsibilities. Use parent components to manage layout and state, and child components for rendering specific content.
- Route Configuration: Define your nested routes in a structured and organized manner. Consider using route configuration files to keep your routing logic separate from your components.
- Layout Components: Create layout components for different sections of your app. These layouts can contain common elements such as headers, footers, and navigation menus. Nest your child routes within these layouts.
- Use Outlet Wisely: The Outlet component should be placed where you want your nested routes to be rendered within the parent component. It's a placeholder for child routes and should not be overused.
- Avoid Deep Nesting: While nested routing is powerful, avoid creating deeply nested routes. Deep nesting can make your codebase harder to understand and maintain. Try to keep your route hierarchy reasonably shallow.
- Centralize Route Definitions: Define all your routes in one central location, such as a Routes.js or RoutesConfig.js file. This promotes consistency and makes it easier to manage routes as your app grows.
- Use Parameters and URL Segments: Utilize URL parameters and segments to create dynamic routes. This is especially useful when you have sections with shared layouts but different content based on the URL.
- Use useParams and useLocation: React Router provides hooks like useParams and useLocation to access route parameters and location information. These hooks are handy for reading URL data within your components.
- Code Splitting: Leverage code splitting to load only the necessary components for specific routes. This improves performance by reducing the initial load time.
- Testing: Write tests for your route components to ensure that navigation and rendering behave as expected. Tools like React Testing Library can help with this.
- Documentation: Document your routing structure, component hierarchy, and any routing-related decisions. This documentation will be valuable for you and your team members.
- Consistent Styling: Maintain consistent styling across your routes by applying global CSS styles or using a CSS-in-JS library. This ensures a cohesive look and feel throughout the app.
Nested routes provide a powerful way to organize and structure your React applications, especially when dealing with multi-level navigation systems. With React Router v6's improved syntax, implementing nested routes has become more intuitive and straightforward. By following the steps outlined in this tutorial, you've learned how to set up a basic nested route structure and create an authentication flow with sub-sections. Feel free to build upon this example and explore more advanced use cases of nested routes in your projects.