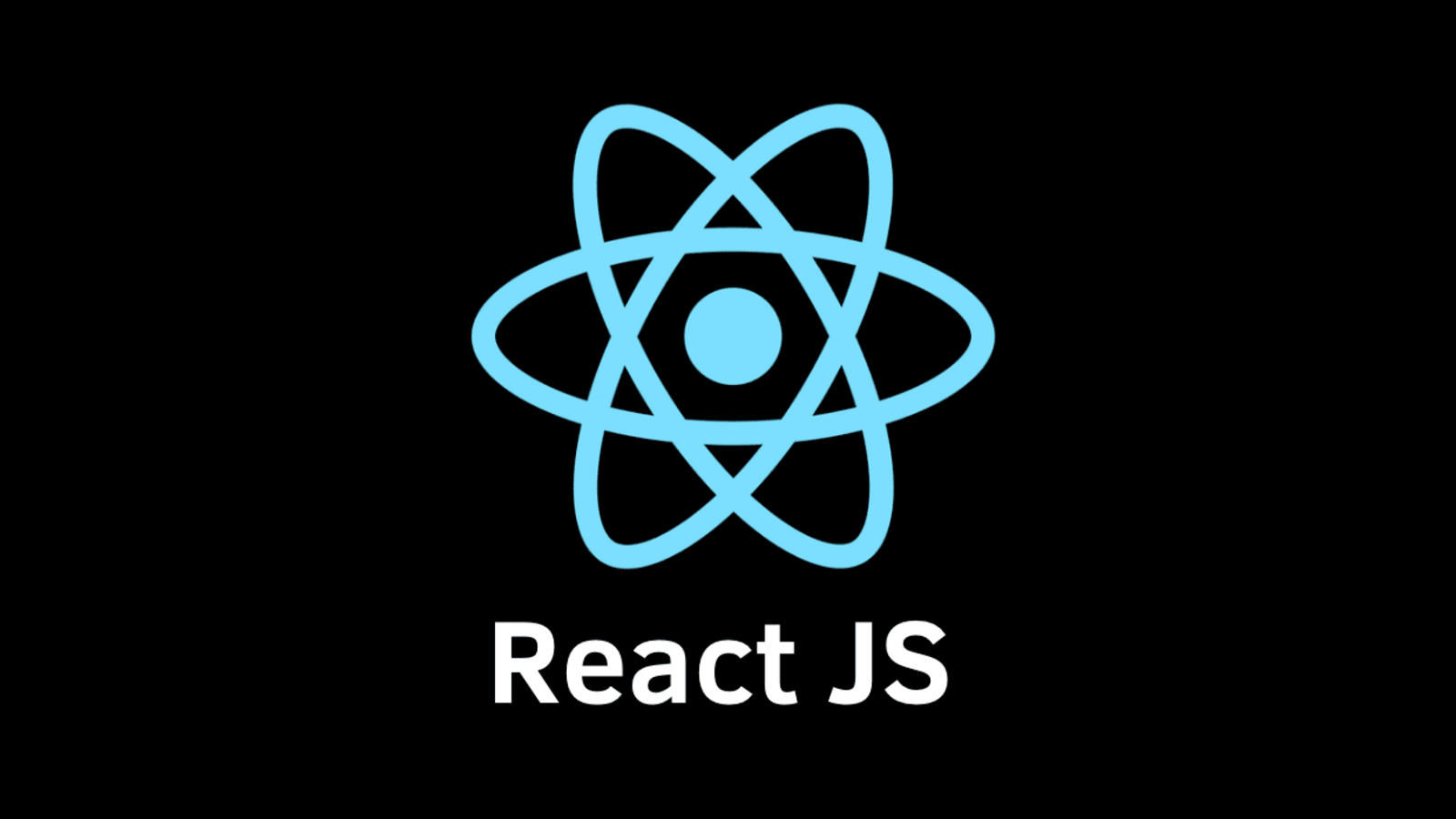
Navigation is a core functionality of web applications, allowing users to move between different views or pages seamlessly. In React applications, navigation is made easy and efficient using the React Router library. In this blog post, we will delve into two important components for navigating between routes: `Link` and `NavLink`. We'll explore their characteristics, benefits, and why you should consider using them.
Introducing `Link` and `NavLink`
In React Router, both `Link` and `NavLink` are components designed to create navigation links that allow users to move between routes. These components help maintain a single-page application experience while updating the URL and rendering the appropriate components based on the selected route.
The `Link` Component
In React Router DOM, the Link component is a fundamental element used to create navigation links in your application. It provides an elegant and efficient way to handle client-side navigation between different routes without causing full page reloads. The Link component helps maintain a single-page application experience while updating the URL and rendering the corresponding route's content. Let's delve into the details of the Link component's usage and characteristics.
import { Link } from 'react-router-dom';
// Usage
<Link to="/about">About Us</Link>
In the above example, when the user clicks the "About Us" link, the URL in the browser's address bar will be updated to /about, and the corresponding route's content will be rendered on the page. Notably, this navigation process occurs without causing a full page reload, resulting in a smoother and more responsive user experience.
Key Characteristics of the Link Component
- Preventing Page Reloads: The primary advantage of the Link component is its ability to prevent full page reloads when navigating between routes. This behavior is crucial for maintaining a seamless single-page application experience.
- URL Update: When a user clicks a Link component, the URL in the address bar is updated to match the specified route. This URL update is important for bookmarking, sharing, and improving search engine optimization (SEO) and accessibility.
- Dynamic Rendering: The Link component contributes to the dynamic rendering of components associated with different routes. This dynamic rendering creates the illusion of navigating between distinct pages within a single-page application.
- Easy-to-Use: Integrating the Link component into your application requires minimal effort. You only need to provide the to prop with the desired route path.
Benefits of Using the Link Component
- Single-Page Application Feel: By using the Link component, you can achieve the look and feel of a multi-page application without the performance drawbacks of full page reloads.
- Improved User Experience: The absence of page reloads enhances the user experience by reducing waiting times and providing smoother navigation.
- SEO and Accessibility: The Link component ensures that search engines and screen readers can interpret the different views within your application, contributing to better SEO and accessibility.
- Code Simplification: The Link component abstracts away the complexities of URL manipulation and navigation logic, resulting in cleaner and more maintainable code.
In summary, the Link component in React Router DOM is an essential tool for creating navigation links that allow users to move between routes in your application. Its ability to prevent full page reloads, update URLs, and enable dynamic rendering enhances the overall user experience and contributes to the efficient functioning of single-page applications.
The `NavLink` Component
In React Router DOM, the NavLink component is a versatile tool for creating navigation links in your application. Similar to the Link component, NavLink enables users to navigate between different routes without causing full page reloads. However, NavLink offers additional features, such as automatic active route highlighting, making it an excellent choice for navigation menus and providing visual feedback to users. Let's dive into the details of the NavLink component's usage, characteristics, and advantages.
import { NavLink } from 'react-router-dom';
// Usage
<NavLink to="/about" activeClassName="active">About Us</NavLink>
The key distinction of the NavLink component is its ability to apply an active class to the rendered element when the current route matches the specified to path. This enables you to style the active navigation link differently, providing users with a visual indication of their current location within the application.
Key Characteristics of the NavLink Component
- Automatic Active Route Highlighting: The standout feature of the NavLink component is its automatic application of an active class to the rendered element when the current route matches the to prop. This simplifies the process of indicating the active route to users.
- Preventing Page Reloads and URL Updates: Similar to the Link component, NavLink also prevents full page reloads and updates the URL while navigating between routes.
- Dynamic Rendering: Like the Link component, NavLink contributes to the dynamic rendering of components associated with different routes.
Benefits of Using the NavLink Component
- Active Route Highlighting: The primary advantage of the NavLink component is its automatic highlighting of active navigation links. This feature enhances the user experience by providing clear visual feedback about the user's current location within the application.
- Single-Page Application Feel: Like the Link component, NavLink maintains the benefits of single-page applications by avoiding full page reloads during navigation.
- Simplified Styling: By applying the active class, the NavLink component simplifies the process of styling active navigation links, allowing you to create distinct visual cues for users.
- Improved Navigation Menu UX: NavLink is particularly useful for creating navigation menus, where highlighting active links helps users quickly identify their position in the application's structure.
Use Cases for NavLink
- Navigation Menus: Use NavLink to build responsive navigation menus that clearly show users which section of the website they are currently viewing.
- Sidebars: Implement NavLink in sidebars to offer users a straightforward way to navigate between different sections or categories of content.
- Tab Navigation: When you have tabbed content, you can use NavLink to highlight the active tab, making it easier for users to understand the context.
- Pagination: If you're implementing pagination and want to indicate which page is currently active, NavLink can provide visual cues.
So, the NavLink component in React Router DOM is an excellent tool for creating navigation links that not only allow users to navigate between routes without page reloads but also provide a visually enhanced user experience. By offering automatic active route highlighting, NavLink simplifies the process of indicating the user's current location within your application and contributes to the creation of user-friendly and visually appealing navigation menus.
Best practices for using the Link and NavLink components in React Router DOM:
Link Component:
- Use for Basic Navigation: Use the Link component for basic navigation between routes when you don't need to indicate the active route or apply specific styling to active links.
- Keep Navigation Clear: Ensure that the text or content within the Link component clearly represents the destination route, helping users understand where the link will take them.
- Use Descriptive Link Text: Use descriptive link text that reflects the purpose of the link. Avoid using generic text like "Click here."
- Minimize Inline Styling: Apply styling to Link components through CSS classes or external stylesheets rather than inline styles, promoting consistency and maintainability.
NavLink Component:
- Highlight Active Links: Use the NavLink component when you want to indicate the currently active route. This is particularly useful for navigation menus and providing users with context.
- Apply Active Styling: Leverage the activeClassName prop of NavLink to apply custom styling to active links, enhancing the visual distinction between active and inactive routes.
- Use for Navigation Menus: NavLink is an excellent choice for navigation menus, especially when users need to navigate between various sections of your application.
- Avoid Overstyling: While styling active links is important, avoid excessive styling that distracts users from the content. Choose a subtle and clear visual indication.
- Keep Link Text Consistent: Maintain consistent link text within your application. Using different wording for the same link across different sections can confuse users.
Remember, the primary goal of both Link and NavLink is to enhance user navigation and provide a smooth and intuitive experience. By following these best practices, you can ensure that your navigation links are effective, user-friendly, and visually appealing.
In this blog post, we've explored the `Link` and `NavLink` components in React Router for navigating between routes in a React application. We discussed their characteristics, advantages, and why they should be your preferred choice for implementing navigation links. By leveraging these components, you can provide users with a seamless and efficient navigation experience while maintaining the benefits of a single-page application.