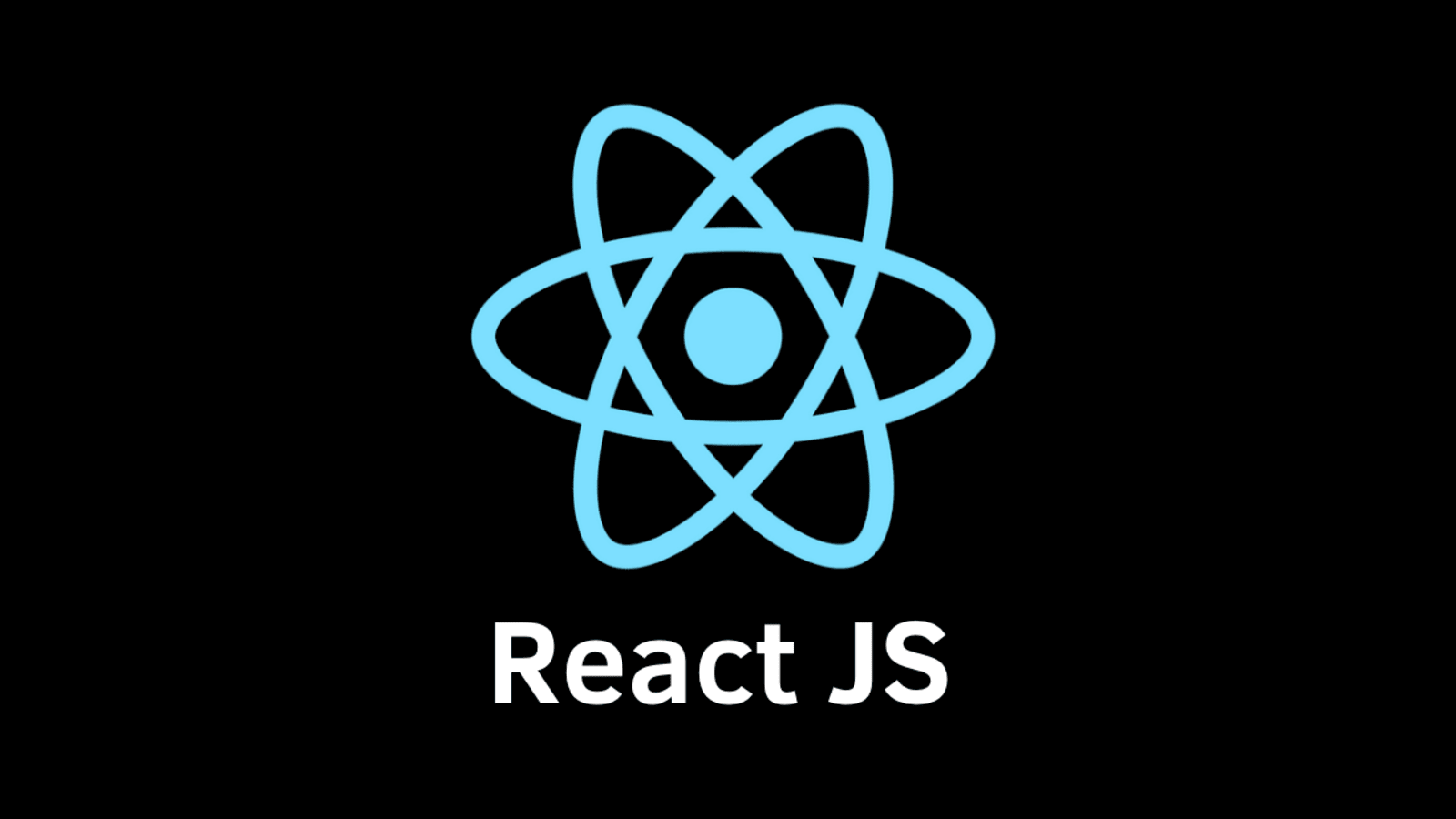
Managing state in a React application can become challenging as the complexity of your project grows. While React's built-in state management with `useState` and `useReducer` is effective for simpler applications, it may not be sufficient for larger projects. This is where MobX comes in. MobX is a powerful state management library that offers a simple and reactive way to manage the state of your React applications.
In this tutorial, we will explore MobX, understand its core concepts, and learn how to integrate it with React to build scalable and maintainable applications.
Introduction to MobX
MobX is a state management library that follows the principles of reactive programming. It allows you to create observables that automatically track changes, trigger reactions, and keep your user interface in sync with the data. MobX provides a simple and intuitive approach to state management, making it a popular choice among React developers.
Advantages:
- Reactive and Declarative: MobX follows a reactive programming approach, where components automatically update in response to changes in the state. This leads to a more declarative and intuitive way of managing state, reducing the need for manual updates and rendering logic.
- Simplicity and Ease of Use: MobX provides a simpler and more straightforward API compared to other state management libraries like Redux. With MobX, you can manage state with minimal boilerplate code, making it easy to learn and use.
- Efficient and Performant: MobX optimizes reactivity and only updates the components that depend on a specific piece of state. This makes MobX highly performant, especially for large and complex applications with multiple state updates.
- Centralized State: MobX allows you to organize the state in a centralized store, making it easier to manage and access state across different components in the application.
- Automatic Dependency Tracking: MobX automatically tracks dependencies between observables and reactions. When an observable changes, only the affected reactions are updated, avoiding unnecessary re-renders in other parts of the application.
- Computed Values: MobX supports computed values, which are derived state that depends on observables. Computed values are automatically updated and provide a way to efficiently calculate derived data.
- Asynchronous Actions Handling: MobX provides a straightforward way to handle asynchronous actions using the runInAction function. This ensures that asynchronous state updates are managed correctly.
- Reactions for Side Effects: With reactions, you can perform side effects, such as logging or API calls, in response to changes in the state. Reactions ensure that side effects are executed in a predictable and controlled manner.
- Minimal Learning Curve: MobX's simplicity and familiar JavaScript syntax make it easy for developers to get started with state management without the need for extensive learning or complex setup.
- Active Community and Ecosystem: MobX has a thriving community and a rich ecosystem of extensions and tools. This means you can find a wide range of libraries and resources to enhance your MobX-based applications.
- Integration with React: MobX integrates seamlessly with React using the useObserver hook or the observer higher-order component. This makes it easy to incorporate MobX into existing React applications.
- Mature and Stable: MobX has been around for several years and is widely adopted in the React community. Its stability and maturity make it a reliable choice for state management in production applications.
- Flexibility: MobX doesn't enforce strict patterns like Redux does. It gives developers the flexibility to choose how they want to structure their state and actions, making it suitable for a wide range of projects.
- Developer Productivity: MobX's reactive approach and minimal boilerplate code reduce the cognitive load for developers, allowing them to focus more on building features and less on managing state.
Core Concepts of MobX
Observables
Observables are the core concept of MobX. They represent values that can be observed for changes. When an observable is updated, any part of the application that uses that observable will automatically be re-rendered.
Actions
Actions in MobX are the functions that modify the state (observables). They are the only way to change the observables. Actions ensure that state modifications are tracked and that reactions are triggered accordingly.
Reactions
Reactions are functions that are automatically run whenever an observable they depend on is updated. They ensure that the user interface stays in sync with the state by automatically re-rendering components that use the observables.
Computed Values
Computed values are derived state that depends on one or more observables. Computed values are automatically updated when their dependencies change, but they are only recalculated when they are accessed, leading to better performance.
Setting Up MobX in a React Application
To use MobX in a React application, you need to install the `mobx` and `mobx-react-lite` packages.
npm install mobx mobx-react-lite
Next, create a store class using MobX `observable` and `action` decorators.
// store.js
import { observable, action } from 'mobx';
class AppStore {
@observable count = 0;
@action incrementCount() {
this.count += 1;
}
}
const store = new AppStore();
export default store;
Creating Observables and Actions
In MobX, you define the state (observables) and the functions that modify the state (actions) in the store class.
// store.js
import { observable, action } from 'mobx';
class AppStore {
@observable count = 0;
@action incrementCount() {
this.count += 1;
}
}
const store = new AppStore();
export default store;
Integrating MobX with React Components
To use MobX observables and actions in React components, you can use the `useObserver` hook from `mobx-react-lite` to create reactive components.
// CounterComponent.js
import React from 'react';
import { useObserver } from 'mobx-react-lite';
import store from './store';
const CounterComponent = () => {
return useObserver(() => (
<div>
<p>Counter: {store.count}</p>
<button onClick={() => store.incrementCount()}>Increment</button>
</div>
));
};
export default CounterComponent;
Computed Values for Derived State
Computed values in MobX are derived state that automatically update based on their dependencies.
// store.js
import { observable, computed, action } from 'mobx';
class AppStore {
@observable count = 0;
@computed get doubledCount() {
return this.count * 2;
}
@action incrementCount() {
this.count += 1;
}
}
const store = new AppStore();
export default store;
Reactions for Side Effects
Reactions in MobX allow you to perform side effects in response to changes in observables.
// store.js
import { observable, reaction, action } from 'mobx';
class AppStore {
@observable count = 0;
constructor() {
reaction(
() => this.count,
(count) => {
console.log(`Count changed: ${count}`);
}
);
}
@action incrementCount() {
this.count += 1;
}
}
const store = new AppStore();
export default store;
Asynchronous Actions with MobX
You can use MobX's `runInAction` to handle asynchronous actions.
// store.js
import { observable, action, runInAction } from 'mobx';
class AppStore {
@observable count = 0;
@action async fetchData() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
runInAction(() => {
this.count = data.count;
});
}
}
const store = new AppStore();
export default store;
Organizing MobX Code
As your application grows, it's essential to organize MobX code into separate files for observables, actions, and reactions. This improves code maintainability and readability.
Best Practices with MobX
- Use MobX sparingly and only for complex state management scenarios.
- Separate concerns by keeping UI components separate from MobX store logic.
- Use `useObserver` only on the components that need to be reactive.
- Avoid unnecessary re-renders by using computed values and selective reactivity.
Performance Considerations
MobX is optimized for performance, but it's essential to avoid unnecessary re-renders by using `useObserver` wisely and using computed values efficiently.
When to Use MobX
MobX is an excellent choice for applications with complex state management requirements, especially those involving deeply nested and interconnected components.
Comparison with Other State Management Libraries
MobX offers a simpler and more reactive approach compared to Redux, making it more suitable for certain types of applications. Consider the specific needs of your application before choosing a state management library.
MobX is a powerful state management library that offers a simple and reactive approach to managing state in React applications. By understanding the core concepts of MobX and integrating it with React components, you can build scalable and maintainable applications with ease. When used judiciously, MobX can greatly
enhance the development experience and improve the performance of your React applications. Happy coding!