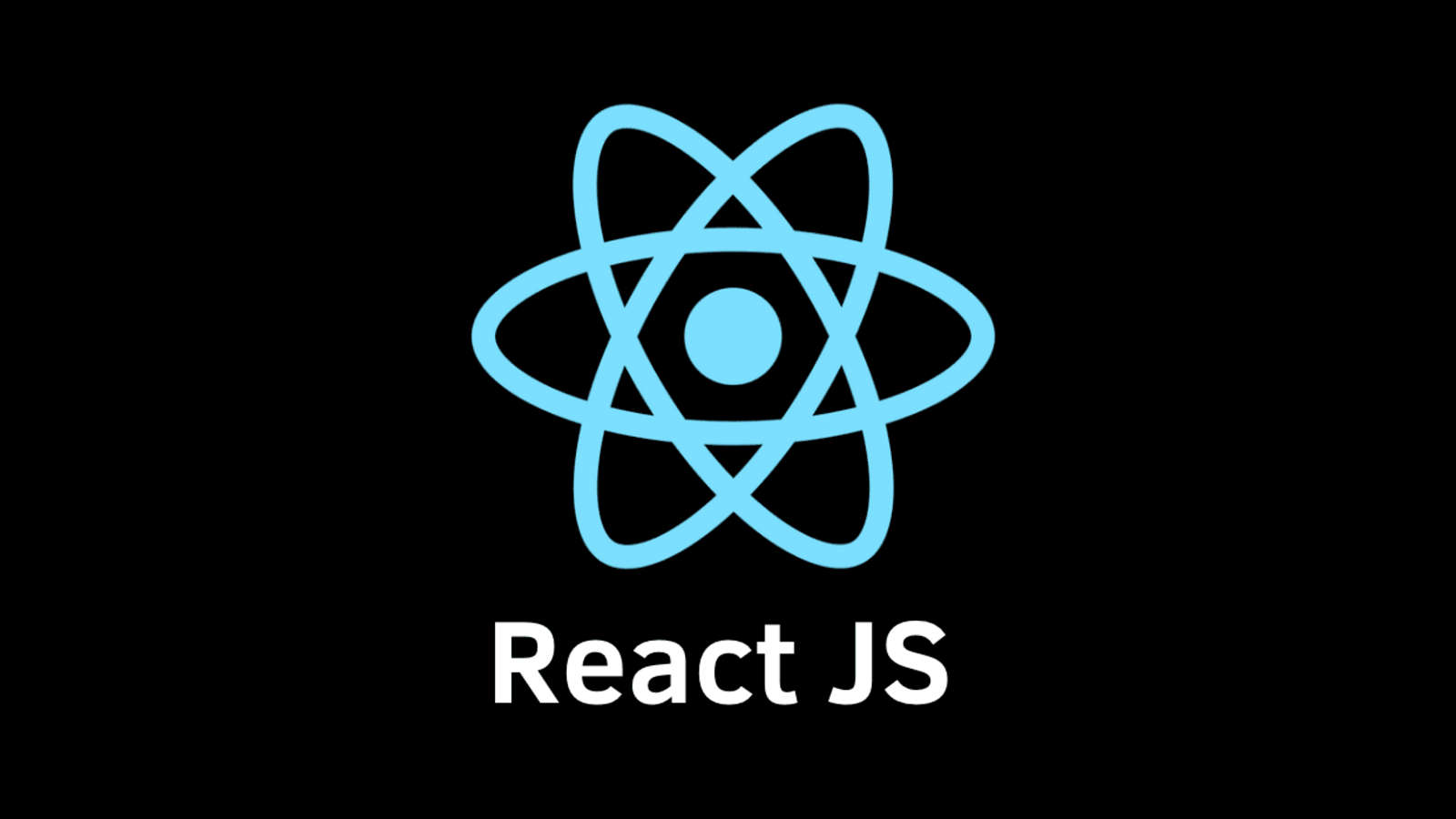
Introduction:
In the realm of web development, React has emerged as a dominant force, enabling developers to build dynamic and interactive user interfaces. At the heart of React lies JSX (JavaScript XML), a syntax extension that combines JavaScript and HTML-like syntax. In this blog post, we will dive into JSX syntax and explore its benefits, showcasing how it enhances the development experience and contributes to the success of React-based projects.
What is JSX?
- JSX is an XML-like syntax extension for JavaScript, introduced by React to define the structure and appearance of UI components.
- It allows developers to write HTML-like code within JavaScript files, making it easier to visualize and compose complex user interfaces.
- JSX resembles a blend of HTML and JavaScript, but it ultimately compiles down to regular JavaScript code.
Examples:
- Basic JSX Element:
const element = <h1>Hello, JSX!</h1>;
In this example, JSX is used to create a simple <h1> heading element with the text "Hello, JSX!". This JSX code will be compiled into regular JavaScript by a tool like Babel.
- Embedding JavaScript Expressions:
const name = "John";
const greeting = <p>Hello, {name}!</p>;
Here, the JavaScript variable name is embedded within curly braces {} inside the JSX code. The value of name will be dynamically rendered within the <p> element, resulting in a personalized greeting.
- Rendering JSX within a Component:
function Greeting() {
return <h2>Welcome to my website!</h2>;
}
ReactDOM.render(<Greeting />, document.getElementById('root'));
In this example, a functional component Greeting is defined, which returns a JSX element <h2> with a welcoming message. The component is then rendered using ReactDOM.render() and appended to an HTML element with the id of "root".
- Applying CSS Classes and Inline Styles:
const btnStyle = {
backgroundColor: 'blue',
color: 'white',
padding: '10px',
};
const button = <button className="btn" style={btnStyle}>Click me</button>;
In this snippet, JSX is used to create a <button> element with a CSS class of "btn" and inline styles defined by the btnStyle object. The styles are applied using the style attribute in JSX.
- Conditional Rendering in JSX:
function Greeting({ isLoggedIn }) {
return (
<div>
{isLoggedIn ? <p>Welcome back!</p> : <p>Please sign in.</p>}
</div>
);
}
Here, the Greeting component receives a prop isLoggedIn. Based on its value, JSX conditionally renders different messages using a ternary operator. If isLoggedIn is true, it renders "Welcome back!" in a <p> element; otherwise, it renders "Please sign in."
These examples showcase the flexibility and power of JSX in combining HTML-like syntax with JavaScript expressions to create dynamic and interactive UI components in React.
Advantages of JSX:
Expressive and Readable:
- JSX brings the power of HTML-like syntax to JavaScript, allowing developers to describe the structure and appearance of components in a more intuitive and readable manner.
- It simplifies the creation of complex UI hierarchies by leveraging familiar HTML tags and attributes, reducing cognitive load and enhancing code comprehension.
Component-Based Approach:
- JSX promotes a component-based architecture, where each UI element is encapsulated within its own reusable component.
- By defining components using JSX syntax, developers can seamlessly combine HTML-like markup with JavaScript logic, creating self-contained and modular building blocks.
JavaScript Integration:
- JSX seamlessly integrates JavaScript expressions and logic within the markup, enabling dynamic content and efficient data manipulation.
- Developers can embed JavaScript expressions within curly braces {} to incorporate variables, functions, and other JavaScript features directly into the JSX code.
Tooling and Static Analysis:
- JSX benefits from excellent tooling support, including syntax highlighting, linting, and code completion, which enhance productivity and help catch potential errors during development.
- Static analysis tools such as ESLint and TypeScript can analyze JSX code for potential issues, improving code quality and maintainability.
Ecosystem Compatibility:
- JSX has become a standard in the React ecosystem and enjoys broad community support, which translates into an extensive library of UI components, tools, and resources.
- It simplifies collaboration among developers by providing a common syntax that is widely adopted and understood within the React community.
JSX Limitations and Considerations:
- JSX introduces a new syntax that may require a learning curve for developers who are accustomed to working with HTML and JavaScript separately.
- It requires a build step to transform JSX code into regular JavaScript using a tool like Babel.
- Mixing logic and markup in JSX can sometimes lead to code that is difficult to maintain and test. It is essential to follow best practices and keep components focused and modular.
Conclusion:
JSX has revolutionized the way we approach UI development with React. By combining the best of JavaScript and HTML-like syntax, it offers a powerful and expressive way to build dynamic and interactive user interfaces. With its readability, component-based approach, seamless JavaScript integration, and robust tooling support, JSX has become an integral part of the React ecosystem. Embrace JSX and unlock the full potential of React to create stunning and efficient web applications.