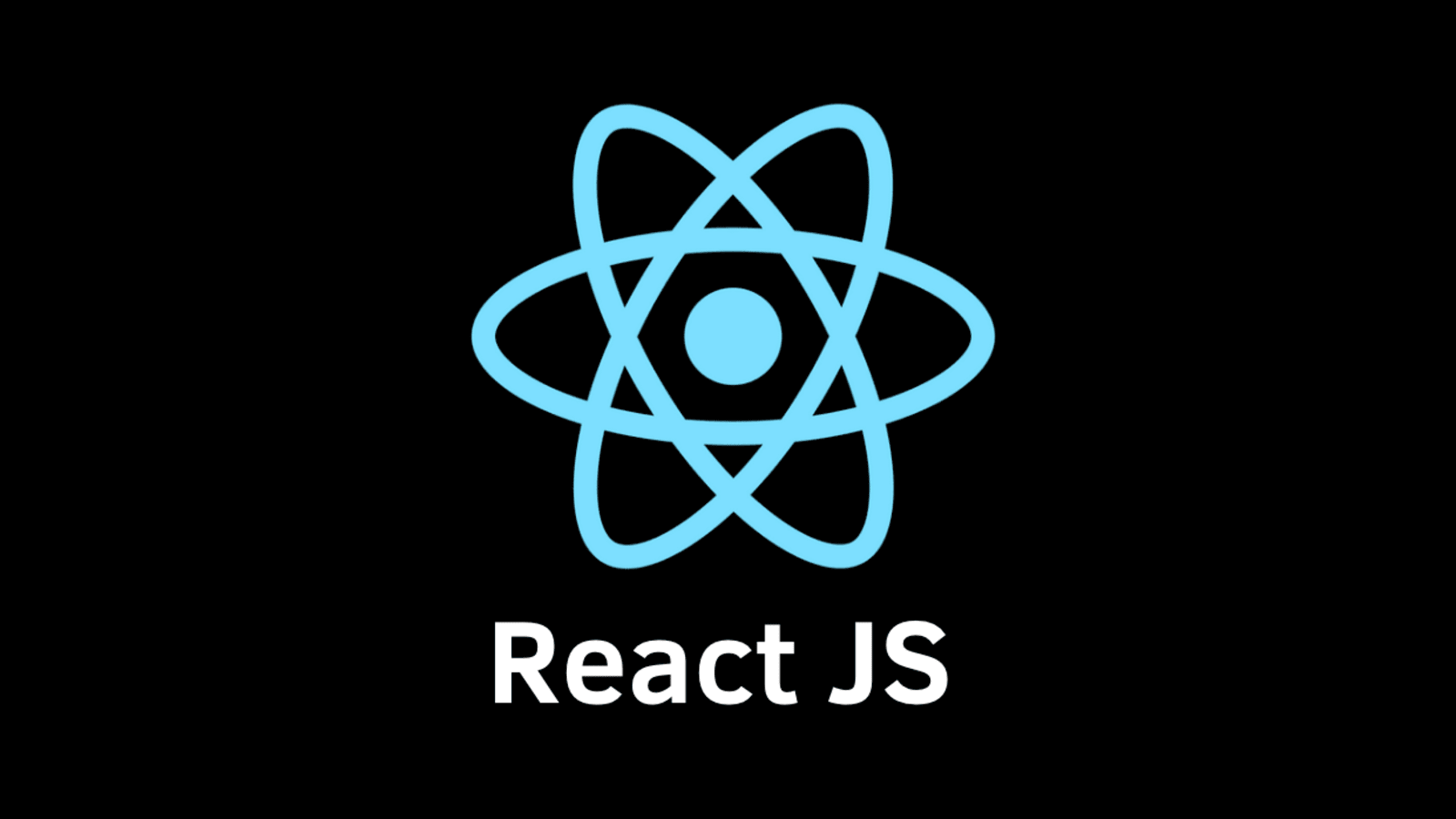
React is a powerful JavaScript library for building interactive and dynamic user interfaces (UIs). At the core of React's design philosophy lies the concept of UI components, which are the building blocks of modern web applications. In this blog post, we will explore what UI components are, why they are essential, and how they enable developers to create reusable and maintainable UIs in React.
What are UI Components in React?
UI components in React are small, self-contained units of code that encapsulate specific parts of the user interface. Each component can have its logic, state, and view, making it easy to manage and reuse across the application. Components can range from simple elements like buttons and input fields to complex elements like modals, tabs, or entire sections of the application.
Advantages of UI Components:
- Reusability: Components are reusable, meaning they can be used multiple times throughout the application without duplicating code. This reusability leads to a more maintainable codebase and faster development.
- Modularity: Components can be developed independently and combined to build complex UIs. This modularity improves code organization and makes it easier to manage different parts of the application.
- Abstraction: Components abstract away the implementation details, allowing developers to focus on the functionality and behavior of each component rather than worrying about how it is rendered.
- Consistency: Consistent components result in consistent user experiences. By using the same UI components across the application, developers ensure a cohesive and polished look.
- Testing: Components can be unit-tested in isolation, making it easier to identify and fix bugs, thereby improving the overall quality of the application.
- Creating UI Components in React: Creating a React component involves two essential steps: defining the component's structure and rendering the component.
Defining the Component:
A React component can be created as either a functional component or a class component.
Functional Component:
Functional components are stateless and rely on the props passed to them for rendering. They are easier to read and write.
Functional components are widely used in React applications due to their simplicity and ease of use. They are commonly used for presentational components, where the primary purpose is to render UI elements and handle minimal to no state management. But later, React Hooks, introduced in React version 16.8, allow functional components to use state and other React features that were previously exclusive to class components. The most commonly used React Hook for state management is the useState Hook.
import React from 'react';
const Button = ({ label, onClick }) => {
return <button onClick={onClick}>{label}</button>;
};
Advantages of functional component:
- Simplicity and Readability: Functional components have a more concise syntax compared to class components. They are essentially JavaScript functions, making them easier to read and write. This simplicity enhances code readability and maintainability.
- Performance: Functional components are lightweight and have a lower overhead than class components. They do not involve the complexity of managing state or lifecycle methods. As a result, functional components are generally faster and more performant.
- No this Keyword: Functional components do not use the this keyword, which eliminates the potential confusion and errors associated with the binding of methods in class components.
- Stateless by Default: Functional components are stateless by default, meaning they do not have their own internal state. This makes them more predictable and less prone to bugs related to state management.
- Functional Programming Paradigm: Functional components align with the principles of functional programming, encouraging a more declarative and functional approach to UI development. This can lead to cleaner and more maintainable code.
- Better for Presentational Components: Functional components are well-suited for presentational components, where the primary purpose is to render UI elements without handling complex state management or lifecycle methods.
- Support for React Hooks: With the introduction of React Hooks, functional components gained the ability to manage state and use other React features that were previously exclusive to class components. This has further enhanced the capabilities and flexibility of functional components.
- Ease of Testing: Functional components are easier to test since they are pure functions that take props as input and produce output based on those props. Testing functional components in isolation is straightforward, simplifying the testing process.
- Encourages Modularity: The simplicity of functional components encourages developers to break down complex UIs into smaller, reusable components. This modularity promotes better code organization and improved maintainability.
- Improved Debugging: Debugging functional components is generally easier due to their simplicity and reduced reliance on the this context.
Class Component:
Class components can hold and manage their state using React's setState method, making them suitable for more complex logic.
Class components in React are an older way of defining components compared to functional components. While functional components have gained popularity with the introduction of React Hooks, class components are still widely used and offer powerful features. Class components are JavaScript classes that extend the React.Component base class provided by the React library.
import React, { Component } from 'react';
class Counter extends Component {
state = {
count: 0,
};
handleIncrement = () => {
this.setState((prevState) => ({
count: prevState.count + 1,
}));
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.handleIncrement}>Increment</button>
</div>
);
}
}
Advantages of Class components:
- State Management: One of the primary advantages of class components is their built-in state management using this.state and this.setState(). State allows components to manage data that may change during the component's lifetime, enabling dynamic updates to the UI based on user interactions or data changes.
- Lifecycle Methods: Class components can take advantage of various lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount. These methods allow developers to perform specific actions at different stages of a component's lifecycle, such as data fetching, cleanup, or handling side effects.
- Legacy Code Support: Class components have been the traditional way of defining React components for a long time, and many older projects use class components. They are still fully supported by React and widely used in existing codebases.
- Organization and Encapsulation: Class components encapsulate behavior and state in a single class, promoting better organization and separation of concerns. This makes it easier to manage complex components with multiple methods and state variables.
- Performance for Large Projects: In some cases, class components can be more performant for large and deeply nested component trees compared to functional components. This is because class components can optimize rendering using lifecycle methods and shouldComponentUpdate to prevent unnecessary re-renders.
- Clearer Prop Types: With class components, prop types are explicitly defined using propTypes. This makes it easier to identify the expected props for a component and helps in debugging and maintaining code.
- HOCs (Higher-Order Components): While higher-order components can be used with functional components, class components have been historically more associated with HOC patterns due to their class-based nature.
Comparison: Functional component vs Class component
1. Syntax:
- Functional Components: Functional components are JavaScript functions that take in props as input and return JSX to define the component's UI. They are more concise and have a simpler syntax.
- Class Components: Class components are JavaScript classes that extend the React.Component base class. They have a more verbose syntax with a constructor and a render() method.
2. State Management:
- Functional Components: Until the introduction of React Hooks, functional components were stateless and couldn't manage their state. With React Hooks, functional components can now handle state using useState and other hooks.
- Class Components: Class components have built-in state management using this.state and this.setState(). They can manage state and perform dynamic updates to the UI based on changes in data or user interactions.
3. Lifecycle Methods:
- Functional Components: Prior to React Hooks, functional components didn't have access to lifecycle methods. With React Hooks (e.g., useEffect), functional components can now use lifecycle-like functionality.
- Class Components: Class components can utilize various lifecycle methods, such as componentDidMount, componentDidUpdate, and componentWillUnmount, to perform actions at different stages of a component's lifecycle.
4. Performance:
- Functional Components: Functional components tend to be more performant, especially when combined with React Hooks. Hooks allow for better optimization, avoiding unnecessary re-renders, and promoting better performance.
- Class Components: Class components can have a slight performance overhead due to their reliance on lifecycle methods. However, for large and deeply nested component trees, class components can sometimes offer better performance optimizations.
5. Code Organization:
- Functional Components: Functional components promote a more functional programming approach, leading to better code organization and reusability. They encourage breaking down complex UIs into smaller, more manageable components.
- Class Components: Class components encapsulate behavior and state in a single class, making them suitable for complex components that require lifecycle methods and advanced state management.
6. Testing:
- Functional Components: Functional components are easier to test since they are pure functions that take props as input and return JSX based on those props.
- Class Components: Class components require more setup and mocking of lifecycle methods during testing, making them relatively more complex to test.
7. Usage and Popularity:
- Functional Components: With the introduction of React Hooks, functional components have become more popular and are now the recommended approach for most use cases.
- Class Components: Class components are still widely used, especially in existing codebases and projects with more complex state management and lifecycle requirements.
Rendering the Component:
To use a component, you can simply import and include it in your JSX code.
import React from 'react';
import Button from './Button';
const App = () => {
return (
<div>
<h1>Hello, React Components!</h1>
<Button label="Click Me" onClick={() => alert('Button clicked!')} />
</div>
);
};
UI components are the foundation of building scalable and maintainable user interfaces in React. They enable developers to create reusable and modular code, resulting in consistent and interactive user experiences. By understanding the concept of UI components and following best practices in their creation, developers can harness the full power of React to create beautiful and robust web applications. As you continue your journey with React, remember that mastering the art of creating UI components is a fundamental step toward becoming a proficient React developer.