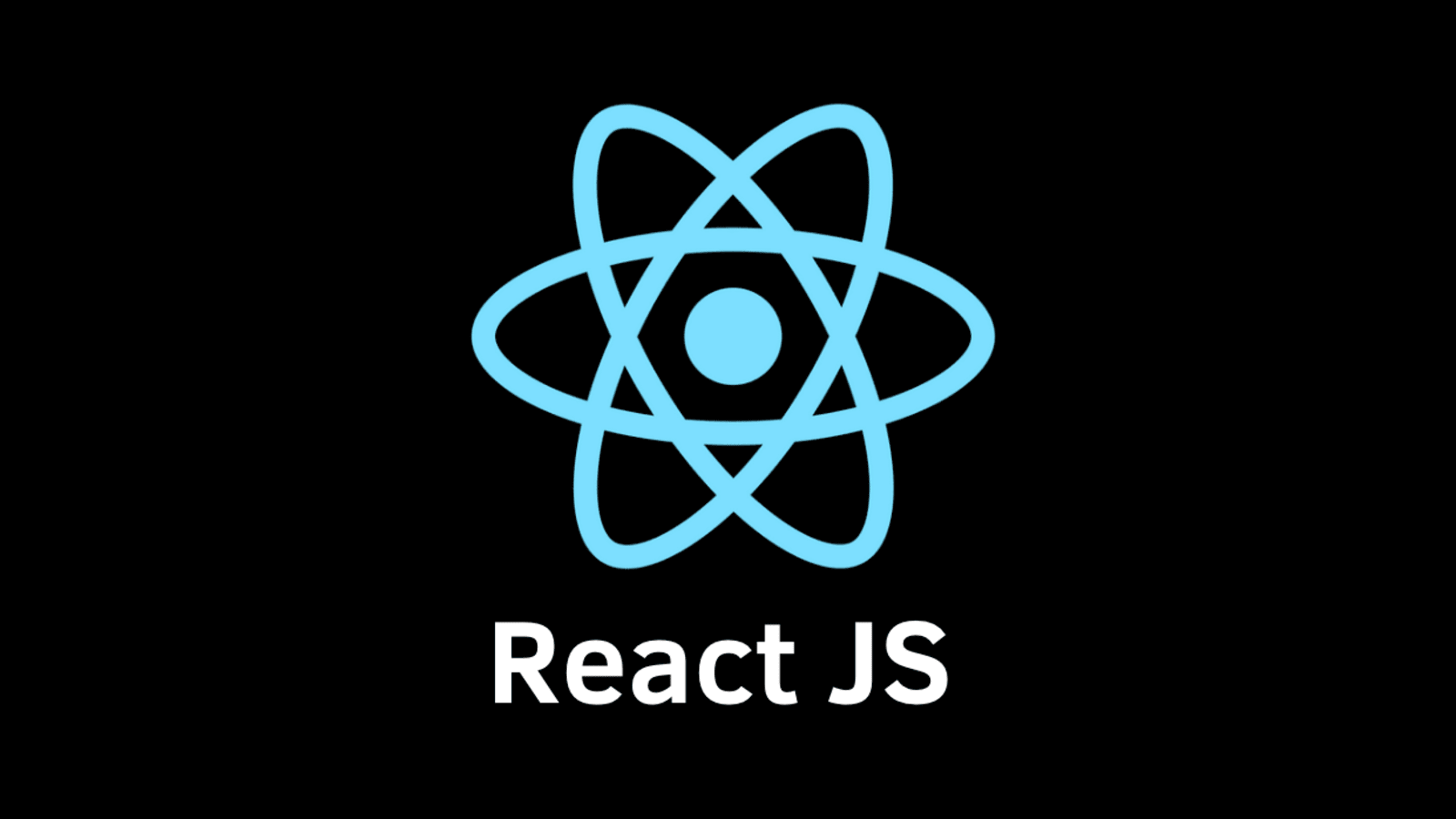
State management is a crucial aspect of building modern web applications, and React.js provides various tools and techniques to handle application state effectively. As applications grow in complexity and interactivity, managing and sharing data between components becomes challenging. State management in React helps maintain a centralized data store, making it easier to manage and synchronize state changes across the application.
In React, state is a fundamental concept that represents the internal data of a component. It allows components to store and manage dynamic data, and when the state changes, the component is re-rendered to reflect the updated data in the user interface. State is one of the core features of React that enables building interactive and dynamic user interfaces.
Understanding React Component State:
In React, a component's state is an internal data storage mechanism that allows a component to store and manage data. It enables components to maintain information and respond to user interactions dynamically. The state is mutable and can be updated using the `setState` method, triggering a re-rendering of the component.
Key Characteristics of React Component State:
- Mutable and Local to the Component:
State is mutable, which means its values can be changed over time. It is also local to the component, meaning it is specific to the component it belongs to and cannot be accessed by other components directly. - Managed by the Component Itself:
Each component manages its own state. It can initialize the state with default values during component initialization, and then modify it using the `setState` method provided by React. - Trigger for Re-rendering:
When the state of a component changes, React automatically triggers a re-render of the component. This allows the UI to reflect the updated state and show dynamic changes to the user. - Declarative:
React state enables a declarative approach to building user interfaces. Instead of manually manipulating the DOM, developers describe what the UI should look like based on the state, and React handles the updates accordingly. - Asynchronous Updates:
The `setState` method is asynchronous, which means React may batch multiple updates and apply them at once for performance optimization. Developers should not rely on the immediate state change after calling `setState`. - Immutable Updates:
To ensure predictability and maintain the integrity of state management, React enforces immutability when updating the state. Developers should never directly modify the state but instead create a new object with updated values and use `setState` to update the state.
Using State in React Components:
To utilize state in a React component, follow these steps:
1. Initialize State:
In the component's constructor or using the `useState` hook (in functional components), initialize the state with an initial value.
2. Accessing State:
Use `this.state` (in class components) or the state variable returned by `useState` (in functional components) to access the current state's values.
3. Updating State:
To update the state, use the `setState` method (in class components) or the state update function returned by `useState` (in functional components). Never modify the state directly.
4. Re-rendering:
When the state is updated, React will automatically trigger a re-render of the component. The new state values will be reflected in the UI.
Example of Using State in React Component:
import React,{useState} from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
const incrementCount=()=>{
setCount(count+1);
}
return (
<div>
<h1>Counter: {count}</h1>
<button onClick={incrementCount}>Increment</button>
</div>
);
}
export default Counter;
In this example, the `Counter` component maintains a state called `count`, which starts at 0. When the "Increment" button is clicked, the `incrementCount` method is called, updating the state and triggering a re-render of the component.
State management is a core concept in React, enabling components to hold and manage dynamic data. Understanding how to work with state is essential for building interactive and data-driven user interfaces in React applications.
Local State vs. Global State:
Local State:
Local state in React refers to the state that is confined and managed within a single component. It is used to store and manage data that is specific to that particular component and is not shared with other components. Local state is primarily used for managing component-level data and handling the internal behavior of a specific component. It is also known as component state.
Global State:
Global state, on the other hand, refers to the state that is shared and accessible across multiple components in the application. It allows data to be shared and synchronized among different parts of the application without the need for direct parent-child relationships between components.
Difference between Local State and Global State:
- Scope:
- Local state is specific to a single component and can only be accessed and modified within that component.
- Global state is accessible and shared across multiple components throughout the application, allowing data to be synchronized among different parts of the UI. - Management:
- Local state is managed within the component itself using the `useState` or `setState` method, making it simple and limited to the component's lifecycle.
- Global state is managed using state management libraries like Redux, MobX, or the Context API, providing centralized and predictable management of application-wide data. - Data Sharing:
- Local state is not shared with other components and is self-contained within the component.
- Global state allows data to be shared and accessed by any component within the application, promoting data reusability and consistency. - Complexity:
- Local state is suitable for simple component-specific data management, leading to less complex code.
- Global state is more suitable for managing complex application-wide data and maintaining a unified state throughout the application.
In summary, local state is used for component-specific data, while global state is employed for managing shared data across multiple components. The choice between local and global state depends on the specific use case and complexity of the application.
Common State Management Libraries:
React offers a rich ecosystem of state management libraries to manage global application state efficiently. Some popular libraries include Redux, MobX, Recoil, Zustand, and many more. These libraries provide predictable ways to manage state, promote unidirectional data flow, and facilitate separation of concerns.
- Redux:
Redux is one of the most widely used state management libraries in the React community. It follows a single source of truth principle, where the entire application state is stored in a single, immutable state tree. Components access the state using selectors and dispatch actions to modify the state. Redux's middleware system allows for advanced functionalities such as asynchronous actions. - MobX:
MobX is another popular state management library that employs observable data to track changes and automatically update components that depend on the data. It offers a more flexible and less boilerplate-driven approach than Redux, making it an excellent choice for applications with rapidly changing data. - Recoil:
Recoil is a relatively new state management library developed by Facebook. It leverages React hooks to create and manage state atoms, selectors, and derived data. Recoil's simplicity and familiarity with hooks make it a promising option for state management in React applications. - Context API:
React's built-in Context API enables you to share state between components without using external libraries. While it may not be suitable for complex applications, it can be a practical solution for managing state in smaller applications or scenarios where using a state management library is overkill.
Best Practices for State Management:
- Avoid prop drilling by lifting state up the component tree or using state management libraries.
- Use selectors to access data from the global state to ensure consistency and reusability.
- Limit the usage of global state to essential data that genuinely needs to be shared across components.
- Separate concerns and keep components as stateless as possible to enhance maintainability and readability.
State management is a crucial aspect of building robust and scalable React applications. Understanding the different approaches to state management and choosing the right library or technique for your specific use case is essential for building maintainable, efficient, and user-friendly applications. By adopting best practices and selecting appropriate state management tools, developers can ensure smooth data flow, efficient updates, and a delightful user experience in their React.js projects.