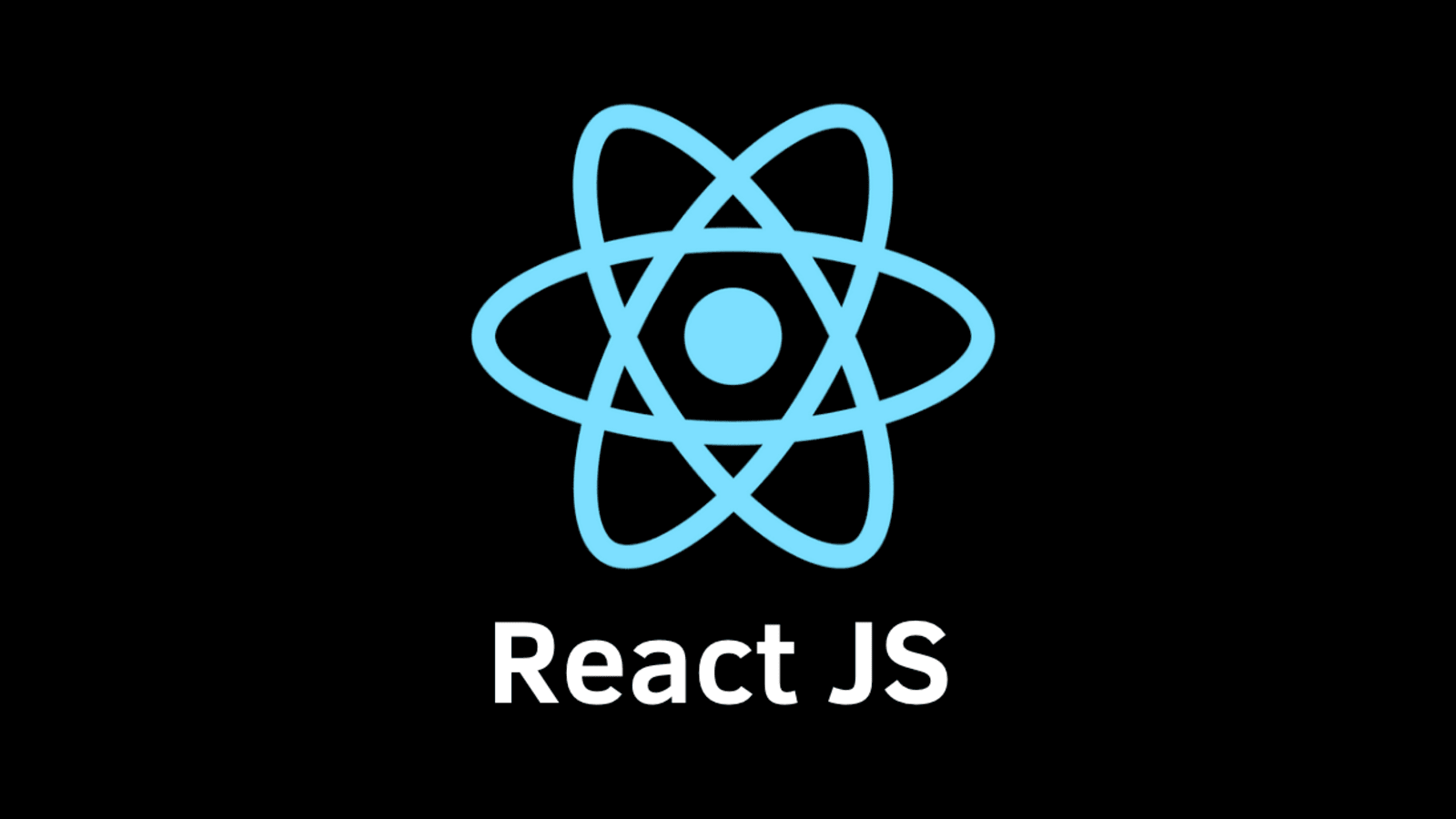
In the realm of web development, creating rich and interactive single-page applications (SPAs) is a common goal. React, a versatile JavaScript library, offers an excellent framework for building user interfaces, but it doesn't inherently handle navigation between different pages or views. This is where React Router, a widely-used routing library, steps in. React Router enables developers to manage navigation effortlessly, transforming React applications into dynamic and multi-view SPAs.
Why Do We Need React Routing?
React, by design, encourages the creation of component-based UIs, allowing developers to build modular and reusable pieces of the application. However, when dealing with multiple pages or views within a web application, a mechanism is needed to transition between these views while maintaining a smooth user experience. This is particularly important for SPAs, where traditional navigation through URL changes and browser refreshes isn't desirable.
Benefits of React Routing:
- Seamless Navigation: React Router facilitates seamless navigation between different views of a web application. Users can move from one section to another without the need for full page reloads, resulting in a more fluid and engaging user experience.
- SPAs with Multiple Views: With React Router, you can create SPAs with multiple views or pages while still having a single HTML page. This approach enhances application performance by reducing the overhead of loading new HTML pages for each view.
- Dynamic URLs: React Router enables dynamic URLs by allowing route parameters. This is crucial for creating routes that depend on user inputs or specific data, such as a profile page with a unique user ID.
- Route-Based Code Splitting: React Router can be integrated with code splitting techniques. This means that you can load only the necessary JavaScript code for the current route, optimizing the initial load time of your application.
- Route Guards and Authentication: React Router provides mechanisms for implementing route guards, which are essential for handling authentication and authorization. You can restrict access to certain routes based on user roles or authentication status.
- Better User Experience: By enabling navigation through different views without the need for full page reloads, React Router contributes to a smoother and more responsive user experience.
React Router DOM:
React Router DOM, available as an npm package, serves as an indispensable tool for implementing dynamic routing within web applications. It empowers you to seamlessly display different pages and enables users to navigate through them. This library provides comprehensive routing solutions for both client and server-side scenarios, making it a key asset in the realm of React development. React Router Dom facilitates the creation of single-page applications (SPAs) – applications with numerous components or pages that are loaded dynamically based on the URL, eliminating the need for page refreshes.
React Router Dom boasts the ability to uphold a smooth and engaging user experience. It achieves this by eliminating the requirement for page refreshes when navigating between links. This streamlined navigation, coupled with its performance optimization, contributes to an improved user experience and overall application performance.
The library comprises several essential components that collectively enable full-fledged routing functionality:
- Router (typically imported as BrowserRouter): This parent component acts as a container for all other routing components. Any content within this component falls under the routing functionality.
- Switch: The Switch component ensures that only the first route that matches the current location is rendered, instead of rendering all matching routes. This enhances the control over the displayed content.
- Route: Routes are pivotal components that examine the current URL and render the associated component when the URL matches the specified path. All routes are nested within the Switch component.
- Link: The Link component is used for creating navigational links to different routes, ensuring seamless movement between various parts of the application.
The Route component, a centerpiece of React Router Dom, requires two parameters. The first parameter defines the URL path, while the second parameter specifies the component to be displayed if the current URL aligns with the specified path.
By enabling such dynamic routing capabilities, React Router Dom significantly enhances the user experience, application responsiveness, and performance. Its set of components provides a holistic solution for creating sophisticated navigation systems in React applications.
Installing React Router DOM:
To install the npm package input the following command in terminal:
npm i react-router-dom
Example:
App.jsx:
import React from 'react';
import { BrowserRouter as Router, Switch, Route, Link } from 'react-router-dom';
import Home from './components/Home';
import About from './components/About';
import Contact from './components/Contact';
const App = () => {
return (
<Router>
<div>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
<li>
<Link to="/contact">Contact</Link>
</li>
</ul>
</nav>
<Switch>
<Route exact path="/">
<Home />
</Route>
<Route path="/about">
<About />
</Route>
<Route path="/contact">
<Contact />
</Route>
</Switch>
</div>
</Router>
);
};
export default App;
In this example, we've imported the necessary components from react-router-dom: BrowserRouter, Switch, Route, and Link.
- The Router component wraps around the entire app, providing the routing context.
- Inside the Router, we've created a navigation menu using the Link component. The to prop in the Link specifies the URL path to navigate to.
- The Switch component ensures that only the first matching Route is rendered. This prevents multiple routes from rendering simultaneously.
- Each Route is defined with a path prop and a corresponding component. When the URL matches the specified path, the associated component is rendered.
Now, let's assume you have three separate component files: Home.jsx, About.jsx, and Contact.jsx.
Home.jsx:
import React from 'react';
const Home = () => {
return <h1>Welcome to the Home Page!</h1>;
};
export default Home;
About.jsx:
import React from 'react';
const About = () => {
return <h1>About Us</h1>;
};
export default About;
Contact.jsx:
import React from 'react';
const Contact = () => {
return <h1>Contact Us</h1>;
};
export default Contact;
With this setup, when you run your React application, you'll see a navigation menu with links to "Home," "About," and "Contact." Clicking on each link will render the corresponding component content without refreshing the entire page.
This example demonstrates the basics of using React Router to create a simple navigation structure for your single-page application. As you delve deeper into React Router, you can explore more advanced features like route parameters, nested routes, authentication guards, and more, enabling you to build complex and interactive web applications.
In the ever-evolving landscape of web development, user experience and interactivity play pivotal roles in shaping the success of modern applications. React, with its component-driven architecture, has revolutionized how we build user interfaces. Yet, the journey doesn't end there. Enter React Router – the dynamic routing powerhouse that transforms React applications into seamless single-page experiences.
In this introductory exploration of React Router, we've embarked on a journey to understand the significance and benefits of this essential library. By enabling the creation of multi-view single-page applications, React Router elevates user engagement and performance to new heights.
We've discovered that React Router liberates us from the constraints of traditional page navigation. No longer must we endure cumbersome page reloads as we transition between different sections of our application. Instead, React Router empowers us to navigate effortlessly, rendering views dynamically and delivering a fluid user experience.
Through the lens of components like Router, Switch, Route, and Link, we've laid the foundation for a navigation structure that defies the limitations of conventional websites. We've witnessed the power of the Switch component, ensuring that only the first matching route is rendered, and the Link component, granting us the ability to create intuitive and efficient navigation menus.