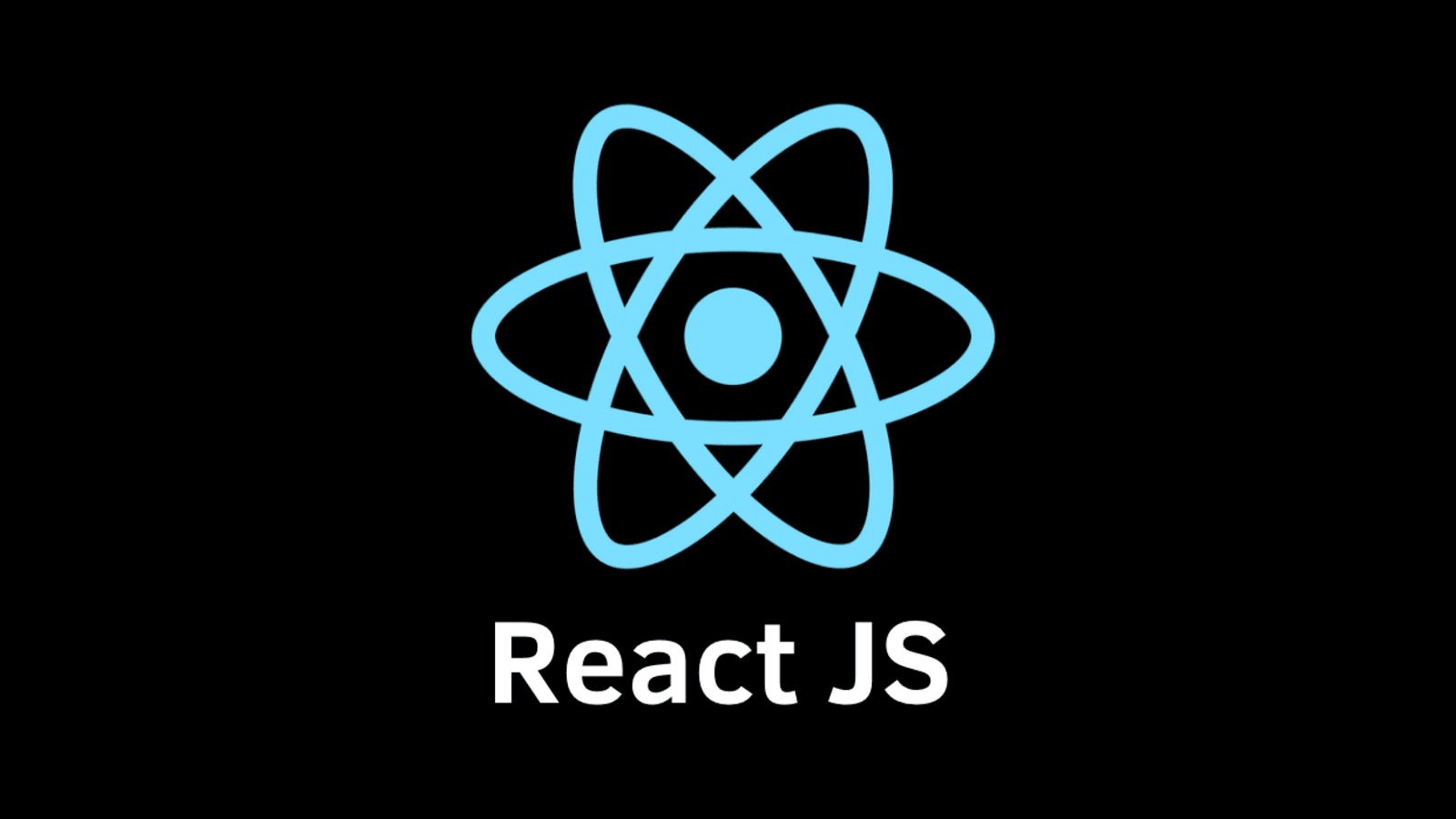
Introduction:
In the ever-evolving landscape of web development, React.js has emerged as a powerful and widely adopted JavaScript library for building dynamic and interactive user interfaces. Developed by Facebook, React.js provides a component-based architecture that enables developers to create reusable UI components and efficiently manage complex state and data flows. In this blog post, we will embark on a journey into the fundamentals of React.js, exploring its key concepts, benefits, and how it revolutionizes the way we build modern web applications.
What is React.js?
React.js is an open-source JavaScript library that allows developers to build UI components and manage the state and behavior of these components efficiently. It follows a declarative and component-based approach, where developers can break down the user interface into reusable and self-contained components. These components can then be composed together to create complex UI structures.
The Virtual DOM:
One of the core concepts of React.js is the Virtual DOM. Unlike traditional web development approaches, React.js introduces a virtual representation of the DOM, a lightweight in-memory copy of the actual browser DOM. When a component's state or props change, React.js efficiently calculates the difference between the virtual DOM and the real DOM, and only updates the necessary parts. This approach minimizes DOM manipulations and enhances the application's performance.
React utilizes its reconciliation process, which involves the following steps:
- React compares the previous Virtual DOM representation (before the state change) with the updated Virtual DOM representation (after the state change) to identify the differences.
- React determines the minimal set of changes needed to update the actual browser DOM to match the updated Virtual DOM.
- Instead of directly manipulating the entire DOM tree, React applies the necessary changes only to the specific elements that require updating. This approach is more efficient and avoids unnecessary re-renders.
By leveraging the Virtual DOM, React minimizes the number of direct manipulations on the real DOM. It performs a diffing process to identify the precise changes and updates only the necessary parts of the DOM tree. This optimized approach results in better performance, especially when dealing with complex and frequently changing UIs.
JSX: Declarative Syntax:
React.js utilizes JSX (JavaScript XML), an extension of JavaScript, to define the structure and behavior of UI components. JSX combines HTML-like syntax with JavaScript functionality, making it intuitive and expressive. With JSX, developers can write declarative code, expressing what the UI should look like based on the current state and props.
import React from 'react';
const ProductCard = ({ product }) => {
const { name, price, description } = product;
return (
<div className="product-card">
<h2>{name}</h2>
<p className="price">${price}</p>
<p>{description}</p>
<button onClick={() => console.log('Add to cart clicked!')}>Add to Cart</button>
</div>
);
};
export default ProductCard;
In this example, we have a ProductCard component that receives a product object as a prop. The product object contains properties such as name, price, and description.
Within the component's JSX, we use curly braces to interpolate the values of the name, price, and description properties dynamically. The <h2> element displays the product name, the <p> element with the price class shows the product price, and the second <p> element displays the product description.
Additionally, we have a <button> element with an onClick event handler attached to it. When the button is clicked, it executes the provided callback function, which logs a message to the console.
By using JSX's declarative syntax, we can easily incorporate dynamic data into our component's UI structure. This allows us to build reusable components that can represent various products, each with its own name, price, and description, without explicitly mentioning specific names or details.
The flexibility of JSX enables us to create dynamic and data-driven UIs, making it an essential tool for building interactive and personalized applications with React.
Component-Based Architecture:
React.js encourages a modular and reusable approach to building user interfaces through components. Components are independent, isolated entities that encapsulate their own logic and UI structure. This modular design allows for easier maintenance, testing, and collaboration among developers. React components can be classified as functional components or class components, with functional components being the preferred approach in modern React development.
import React from 'react';
const Button = ({ text, onClick }) => {
return (
<button onClick={onClick} className="button">
{text}
</button>
);
};
export default Button;
In this example, we have a functional component called Button that accepts two props: text and onClick. The text prop represents the text content of the button, while the onClick prop is a callback function that will be executed when the button is clicked.
Within the component's JSX, we define a <Button> element that renders the button on the page. The onClick prop is attached to the button's onClick event, so when the button is clicked, the provided callback function will be triggered.
To use this button component in another component, you can import it and include it in the JSX markup. For example:
import React from 'react';
import Button from './Button';
const App = () => {
const handleClick = () => {
console.log('Button clicked!');
};
return (
<div>
<h1>My App</h1>
<Button text="Click Me" onClick={handleClick} />
</div>
);
};
export default App;
In this example, we import the Button component and include it within the App component's JSX markup. We pass the text prop as "Click Me" and the onClick prop as the handleClick function, which simply logs a message to the console when the button is clicked.
By creating reusable components like this, you can easily customize and reuse the button throughout your application, providing consistent functionality and appearance while promoting code reusability.
One-Way Data Flow:
React.js enforces a unidirectional data flow, making it easier to understand how data changes propagate through the application. Data flows from parent components to child components through props, while child components can trigger events via callbacks to modify the parent's state. This predictable data flow ensures better code organization and simplifies debugging.
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
};
export default Counter;
In this example, we have a Counter component that manages a count state using the useState hook from React. The initial value of count is set to 0.
Within the component's JSX, we render the current value of count within a <p> element using curly braces {} to interpolate the value. Below that, we have a <button> element with an onClick event handler that calls the increment function.
When the button is clicked, the increment function is executed. Inside the increment function, we update the count state by calling the setCount function with the updated value of count + 1. React then re-renders the component with the new count value, reflecting the updated count on the UI.
This example demonstrates the one-way data flow in React. The count value is managed by the component's internal state and can only be modified by invoking the setCount function. The component itself is responsible for handling the state changes and rendering the updated UI.
By enforcing this unidirectional flow of data, React simplifies the understanding and debugging of state changes within an application. It ensures that the application's state is predictable and that data mutations occur in a controlled manner, leading to more maintainable and scalable codebases.
The one-way data flow in React helps prevent unexpected side effects and makes it easier to trace how data changes propagate through the components, resulting in a more reliable and manageable application.
React Ecosystem:
React.js has a vibrant ecosystem with a vast array of libraries, tools, and frameworks that enhance its capabilities. Libraries like Redux, React Router, and Axios extend React's functionality for state management, routing, and data fetching. Frameworks like Next.js enable server-side rendering and provide additional features for building complete web applications.
Benefits of React.js:
React.js offers several benefits that have contributed to its widespread adoption in the web development community:
- Enhanced performance through the efficient Virtual DOM diffing algorithm.
- Reusability and maintainability with modular component-based architecture.
- Declarative syntax (JSX) for expressing UI structures concisely.
- A thriving ecosystem with abundant community support and resources.
- Seamless integration with existing JavaScript frameworks and libraries.
Conclusion:
React.js has revolutionized the way we build dynamic user interfaces in web applications. With its component-based architecture, efficient Virtual DOM, and declarative syntax, React enables developers to create scalable, reusable, and high-performing UI components. By embracing React.js, developers can unlock new possibilities and build engaging user experiences. Whether you are starting a new project or looking to upgrade your skills, React.js is undoubtedly a valuable tool in your web development toolkit.