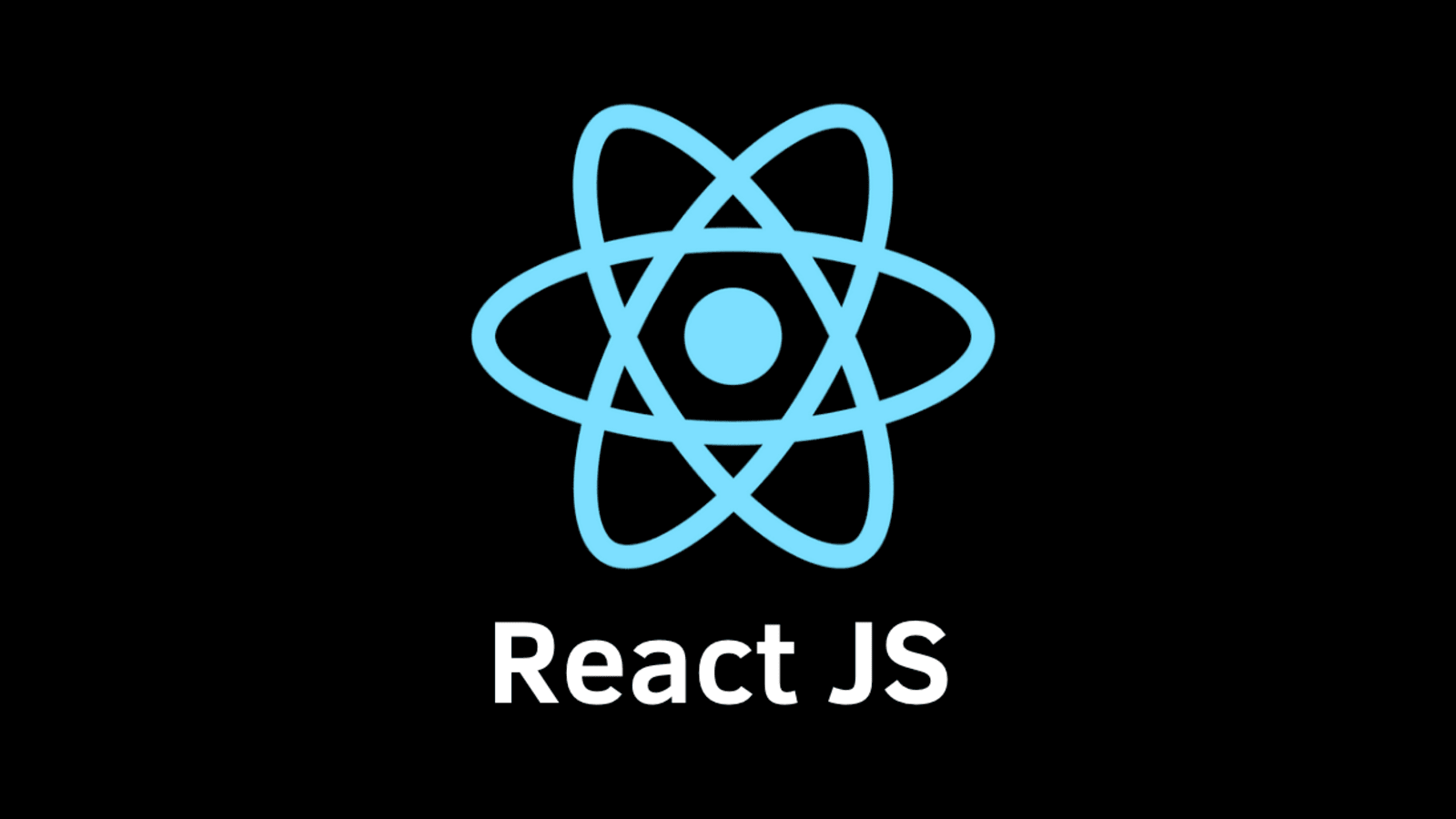
CSS frameworks are collections of pre-designed CSS styles and components that simplify web development by providing ready-made design patterns and responsive layouts. When combined with React, a popular JavaScript library for building user interfaces, CSS frameworks can enhance the visual appeal and functionality of React applications while reducing the need for custom styling. In this blog post, we'll delve deeper into the process of integrating CSS frameworks with React, providing comprehensive examples and detailed explanations.
Understanding CSS Frameworks:
CSS frameworks offer a set of standardized styles, layout structures, and reusable UI components. They aim to streamline web development by providing a consistent design language, reducing development time, and ensuring cross-browser compatibility. Popular CSS frameworks include Bootstrap, Ant design, Foundation, Bulma, and Semantic UI, Tailwind among others.
The Benefits of Integrating CSS Frameworks with React:
- Rapid Prototyping: CSS frameworks offer pre-designed components and styles, enabling developers to quickly create and test user interfaces without spending time on styling from scratch.
- Consistent Design Language: CSS frameworks follow design principles that create uniformity in appearance and behavior across the application, fostering a cohesive user experience.
- Responsive Design: Many CSS frameworks are mobile-first and responsive by design, ensuring that React applications adapt smoothly to various devices and screen sizes.
- Cross-Browser Compatibility: CSS frameworks handle browser-specific inconsistencies, saving developers from the need to write extensive CSS hacks for different browsers.
- Community Support: Popular CSS frameworks have large and active communities, providing regular updates, bug fixes, and extensive documentation.
Bootstrap:
Bootstrap is a popular and widely-used front-end framework that provides a collection of CSS and JavaScript components for building responsive and visually appealing websites and web applications. It was originally created by Twitter and is now an open-source project maintained by a community of developers.
Let's walk through the steps to integrate a Bootstrap CSS framework with React:
Step 1: Install the CSS Framework: Use a package manager like npm or yarn to install the desired CSS framework. For example, to install Bootstrap, run:
npm install bootstrap
Step 2: Import the CSS File: In your main application file (e.g., index.jsx or App.jsx), import the CSS file of the CSS framework. For Bootstrap, import the CSS file as follows:
import 'bootstrap/dist/css/bootstrap.min.css';
Step 3: Use the Components: With the CSS framework imported, you can now use its components throughout your React application. For instance, Bootstrap offers ready-to-use components such as buttons, forms, navigation bars, modals, and more. You can integrate these components into your React components' JSX seamlessly.
Example:
Integrating Bootstrap with React:
Let's illustrate the integration of Bootstrap with React through an example of a simple form:
import React from 'react';
const CssFrameworkBootstrap = () => {
return (
<div className="container mt-5">
<h1>CSS Framework (Bootstrap)</h1>
<h2>Sign Up Form</h2>
<form>
<div className="form-group">
<label htmlFor="name">Name:</label>
<input type="text" className="form-control" id="name" />
</div>
<div className="form-group">
<label htmlFor="email">Email:</label>
<input type="email" className="form-control" id="email" />
</div>
<button type="submit" className="btn btn-primary mt-2 mx-auto d-block w-100">Submit</button>
</form>
</div>
);
};
export default CssFrameworkBootstrap;
In this example, we utilize Bootstrap's CSS classes, such as container, mt-5, form-group, form-control, btn, and btn-primary, to style the form. These classes are part of the Bootstrap CSS framework and enable us to create a clean and responsive form layout without writing custom CSS styles.
Ant Design:
Ant Design is a popular and comprehensive React UI library that provides a wide range of pre-designed components and styles to build modern and responsive web applications. It is specifically designed for React and follows the principles of great user experience and consistent design language.
To use Ant Design with React, follow these steps:
Step 1: Install Ant Design: Install the Ant Design library and its required dependencies:
npm install antd
Step 2: Import CSS: Import the Ant Design CSS file in your application's entry point (usually index.js or App.js):
import 'antd/dist/reset.css';
Step3: Using Ant Design Components: You can now use Ant Design components in your React components. For example, using the Button component:
import React from 'react';
import { Button } from 'antd';
const CssFrameworkAntd = () => {
return (
<>
<h1>CSS Framework (Antd)</h1>
<Button type="primary">Click Me</Button>
</>
);
};
export default CssFrameworkAntd;
In this example we used the Button component from the Ant design framework.
Customization: Ant Design allows customization through CSS variables, themes, and less/sass variables. You can modify the default styles to match your application's design requirements.
Using Ant Design Icons: Ant Design also provides a set of icons that you can use in your application. To use icons, you need to install the @ant-design/icons package:
npm install @ant-design/icons
Then, import and use the desired icons in your components:
import { SmileOutlined } from '@ant-design/icons';
const MyComponent = () => {
return (
<>
<h1>Hello, Ant Design with React!</h1>
<Button type="primary" icon={<SmileOutlined />}>
Click Me
</Button>
</>
);
};
In this example, we used the SmileOutlined icon from antd icons within a Button component.
Using Ant Design with React allows you to build professional and feature-rich user interfaces quickly and efficiently. The library provides an extensive set of components, utilities, and styles to enhance your development experience and create visually appealing applications. With its focus on great design and user experience, Ant Design is an excellent choice for building modern web applications with React.
Foundation:
Foundation is a responsive front-end framework that offers a collection of CSS and JavaScript components for building modern and mobile-friendly web applications.
Here's a step-by-step guide on how to use Foundation CSS in a React.js project:
Step 1: Install Foundation CSS: Install the Foundation CSS framework and its dependencies using npm:
npm install foundation-sites
Step 2: Import and Initialize Foundation: In your application's entry point (usually index.js or App.js), import the Foundation CSS file and initialize it by calling the $(document).foundation() function:
import React from "react";
import ReactDOM from "react-dom";
import "foundation-sites/dist/css/foundation.min.css";
import $ from "jquery"; // Make sure to install jquery using npm or yarn
import "foundation-sites";
import App from "./App";
$(document).foundation();
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById("root")
);
Step 3: Using Foundation Components: Now you can use Foundation components in your React components. For example, using the Button component:
import React from 'react';
const CssFrameworkFoundation =() => {
return (
<>
<h1>CSS Framework (Foundation)</h1>
<button className="button">Click Me</button>
</>
);
};
export default CssFrameworkFoundation;
Customization: Foundation provides various customization options through its settings and SCSS variables. You can override the default styles and adapt the framework to your project's design requirements.
Foundation JavaScript Components: Besides CSS, Foundation includes JavaScript components like accordions, modals, tooltips, and more. You can also use these components in your React application, but make sure to properly import and initialize the required JavaScript files.
Remember to install any additional dependencies that Foundation might require, like jQuery or Popper.js. Check the Foundation documentation for specific usage details and the required dependencies.
By integrating Foundation CSS into your React.js project, you can take advantage of its responsive layout system, pre-designed components, and modern design elements, making it easier to create visually appealing and mobile-friendly web applications.
Integrating CSS frameworks like Bootstrap, Antd, Foundation with React can significantly enhance the development process and elevate the aesthetics and functionality of your applications. By following the simple steps of installation, importation, and usage, developers can leverage the power of CSS frameworks alongside the reactivity and versatility of React. Whether you're building a small personal project or a large-scale web application, integrating CSS frameworks with React is a valuable approach to boost productivity, ensure design consistency, and craft visually appealing user interfaces.