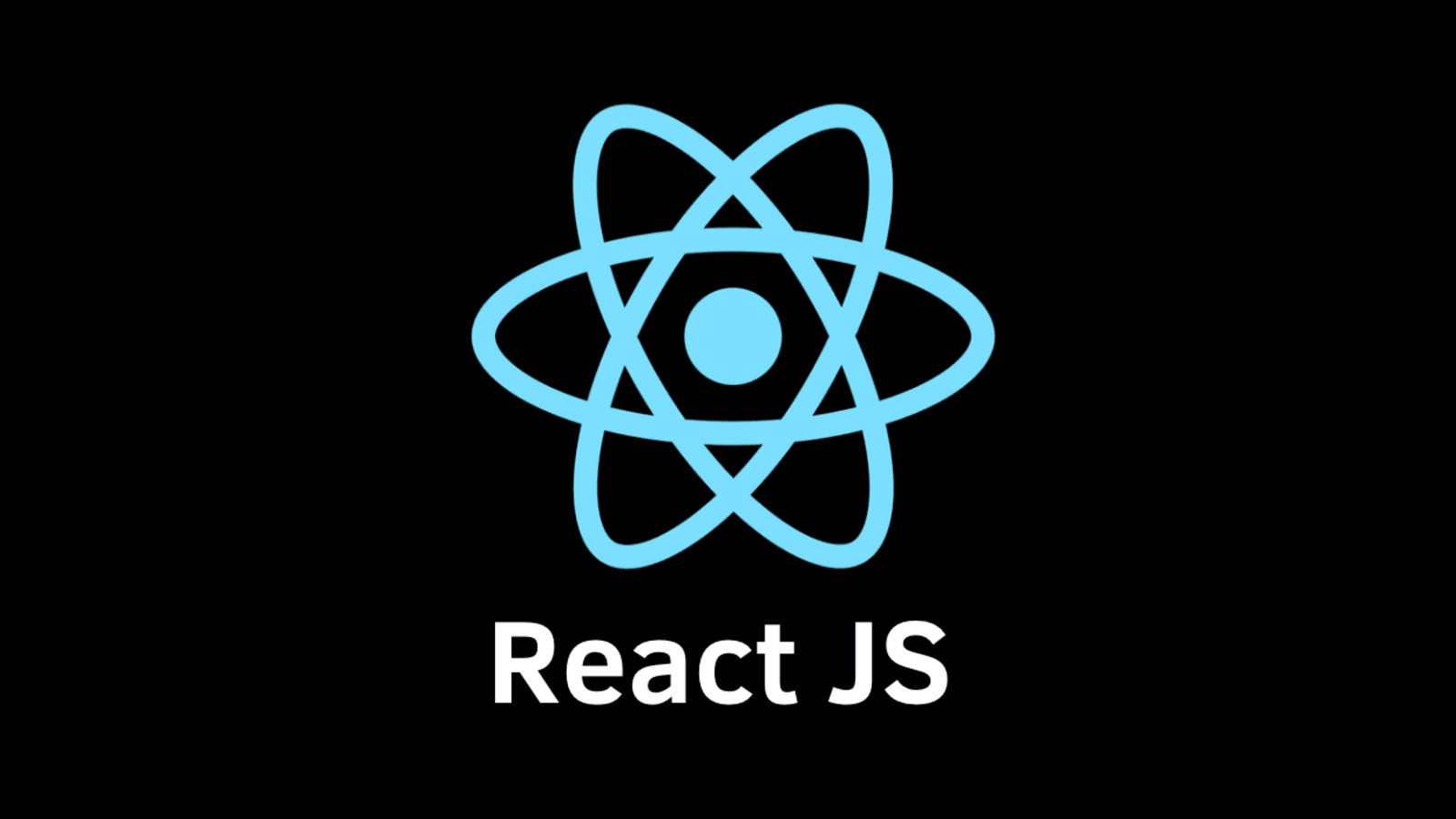
Create a New React Project:
We will use vite to create a new react project.
Vite is a fast and opinionated build tool and development server for modern web applications. It is specifically designed to optimize the development experience by leveraging native ES modules in modern browsers.
Key Features of Vite.js:
- Lightning-Fast Development Server: Vite.js provides an extremely fast development server that leverages native ES modules in modern browsers. It uses the browser's built-in module loading capabilities, resulting in rapid HMR (Hot Module Replacement) and instant reloading during development.
- Blazing-Fast Builds: Vite.js optimizes build performance by only bundling the modules that are explicitly imported, rather than bundling the entire application. This on-demand bundling approach significantly speeds up build times and allows for efficient caching.
- Supports Multiple Frameworks: Vite.js is framework-agnostic and works seamlessly with popular frontend frameworks like React, Vue.js, and Preact. It provides out-of-the-box support and optimizations for these frameworks, allowing developers to enjoy a smooth development experience.
- Dependency Pre-Bundling: Vite.js leverages a feature called "dependency pre-bundling" to speed up the initial load time of your application. It analyzes your project's dependencies and bundles them into a highly optimized format, resulting in faster load times.
- Plugin System: Vite.js offers a flexible plugin system that allows developers to customize and extend its functionality. Developers can create and use plugins to enhance their development workflow, add custom features, or integrate with other tools.
- Support for TypeScript and CSS Pre-processors: Vite.js has built-in support for TypeScript, allowing developers to write type-safe code seamlessly. Additionally, it supports CSS pre-processors like Sass, Less, and Stylus, making it easy to use pre-processed CSS in your project.
- Ecosystem Integration: Vite.js integrates well with the wider JavaScript ecosystem, including tools like Babel, ESLint, and PostCSS. This ensures compatibility with existing workflows and enables developers to leverage their preferred tools and configurations.
- Vite.js focuses on improving developer productivity and build performance, making it an excellent choice for modern web application development. Whether you're building a small prototype or a large-scale application, Vite.js can help streamline your development process and deliver a faster, more efficient user experience.
To install vite:
npm i -g create-vite
To create a new react project:
npx create-vite@latest getting-started --template react
This command initializes a new project using Vite's template for React.
Navigate to the Project Directory:
Change to the newly created project directory using the command:
cd getting started
To install the packages required for the react app:
npm install
Start the Development Server:
Start the development server by running:
npm run dev
This will launch your React application in a development environment.
In my case the the app is started at port 5173. So I can browse the app by visiting http://127.0.0.1:5173 .
If you open the app on your browser you will find this -
We can edit the page by editing /App.jsx file:
import { useState } from 'react'
import reactLogo from './assets/react.svg'
import viteLogo from '/vite.svg'
import './App.css'
function App() {
const [count, setCount] = useState(0)
return (
<>
<div>
<a href="https://vitejs.dev" target="_blank" rel="noreferrer">
<img src={viteLogo} className="logo" alt="Vite logo" />
</a>
<a href="https://react.dev" target="_blank" rel="noreferrer">
<img src={reactLogo} className="logo react" alt="React logo" />
</a>
</div>
<h1>Vite + React</h1>
<div className="card">
<button onClick={() => setCount((count) => count + 1)}>
count is {count}
</button>
<p>
Edit <code>src/App.jsx</code> and save to test HMR
</p>
</div>
<p className="read-the-docs">
Click on the Vite and React logos to learn more
</p>
</>
)
}
export default App
Let's change the code to:
import './App.css'
function App() {
return (
<>
<h1>Hello World!</h1>
</>
)
}
export default App
Now if we see in the browser we get: