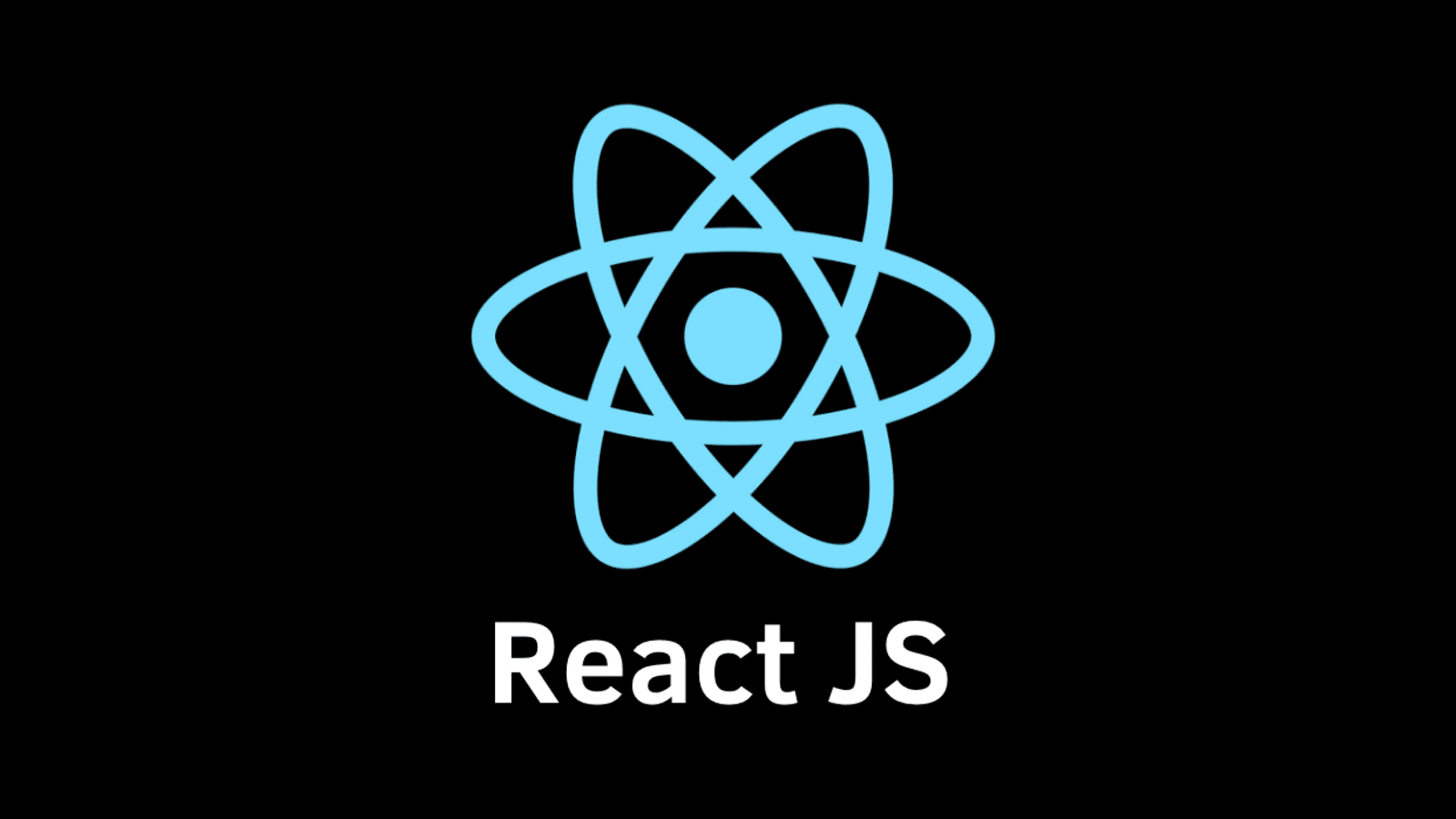
Selecting the right state management solution for your React project is a crucial decision that can impact development speed, code maintainability, and performance. With several options available, including `useReducer`, Context API, Redux, Redux Toolkit, and MobX, understanding their strengths and when to use them is essential. In this comprehensive tutorial, we'll guide you through these state management solutions, explain their use cases, and provide detailed code examples for each.
Importance of State Management:
State management is a fundamental aspect of modern frontend development, and its importance cannot be overstated. In a React application, state refers to the data that defines the current condition of the user interface, including user inputs, fetched data, and dynamic UI changes. Effective state management is essential for several reasons:
- Maintains Data: Keeps data consistent and accessible across components and interactions.
- Facilitates Communication: Enables components to communicate and share data without excessive prop passing.
- Manages Global State: Handles global data like authentication status, themes, and shopping carts.
- Supports Reusability: Promotes component reusability by separating data from components.
- Enables Dynamic UI: Triggers automatic UI updates based on changing data.
- Ensures Predictability: Provides a structured flow of data for easier debugging and maintenance.
- Optimizes Performance: Some solutions optimize performance through memoization and selective rendering.
- Simplifies Scaling: Organized state management aids teamwork and supports scalability.
- Enhances Debugging: Well-structured state management aids in identifying and resolving issues.
In summary, state management is at the core of delivering a seamless and interactive user experience in modern web applications. It empowers developers to handle data effectively, ensures consistent and predictable behavior, and enables the creation of maintainable, scalable, and performant codebases. Choosing the right state management solution tailored to your project's needs is a critical decision that can greatly impact the overall success of your application.
`useReducer`: A Simple Built-in Option:
useReducer is a React hook that allows you to manage state using a reducer function. It takes an initial state and a dispatch function. The reducer calculates the next state based on dispatched actions, which are objects describing state changes. It's suitable for complex state transitions, atomic updates, and can optimize performance. It's a flexible alternative to useState, especially for managing more intricate state logic.
When to Use `useReducer
- Complex Logic: Complex state transitions or calculations that involve multiple variables.
- Multiple Updates: Simultaneous updates to different parts of the state with a single action.
- Shared Logic: Consolidation of state-related logic shared among multiple components.
- Performance Optimization: Preventing unnecessary re-renders by using memoized dispatch functions.
- Context API Integration: Managing global state within a Context API provider.
- Custom Hooks: Creating custom hooks that encapsulate advanced state management.
- Predictable Updates: Enforcing predictability in state changes for debugging.
Example: Creating a Simple Counter:
import React, { useReducer } from 'react';
// Reducer function
const counterReducer = (state, action) => {
switch (action.type) {
case 'INCREMENT':
return state + 1;
case 'DECREMENT':
return state - 1;
default:
return state;
}
};
const Counter = () => {
const [count, dispatch] = useReducer(counterReducer, 0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => dispatch({ type: 'INCREMENT' })}>Increment</button>
<button onClick={() => dispatch({ type: 'DECREMENT' })}>Decrement</button>
</div>
);
};
Context API: Global State with Less Boilerplate:
The Context API is a feature provided by React that enables you to manage global state and share data across components without the need for prop drilling. It's a powerful tool for passing data through the component tree without explicitly passing props at each level. Context API is particularly useful when you have data that many components in your application need to access.
When to Use Context API:
- Share global data across many components.
- Avoid excessive prop drilling in your component tree.
- Manage theming, user authentication, or localization settings.
- Handle dynamic UI configuration or feature toggles.
- Inject dependencies into components.
- Manage complex state transitions without a full state management library.
- Improve the reusability of components across different parts of your app.
- Integrate with third-party libraries or APIs that require configuration.
Example: Managing Dark Mode State:
import React, { createContext, useContext, useState } from 'react';
// Create context
const DarkModeContext = createContext();
const App = () => {
const [darkMode, setDarkMode] = useState(false);
return (
<DarkModeContext.Provider value={{ darkMode, setDarkMode }}>
<div className={darkMode ? 'dark' : 'light'}>
<ToggleDarkModeButton />
</div>
</DarkModeContext.Provider>
);
};
const ToggleDarkModeButton = () => {
const { darkMode, setDarkMode } = useContext(DarkModeContext);
return (
<button onClick={() => setDarkMode(!darkMode)}>
Toggle Dark Mode
</button>
);
};
Redux: Predictable State Container:
Redux is a state management library for JavaScript applications, commonly used with React but also compatible with other frameworks. It provides a predictable and centralized way to manage the state of your application, making it easier to understand, debug, and maintain.
When to Use Redux:
- Complex State Logic: When your application's state interactions become complex and managing them with local component state becomes challenging.
- Global State Needs: If your app requires a central store for managing global state, like user authentication, theme settings, or shopping carts.
- Large-scale Apps: For large projects with many interconnected components, Redux provides a structured way to manage state without excessive prop passing.
- Predictable State Updates: When you need a predictable and controlled way of updating state, making debugging and understanding changes easier.
- Time Travel Debugging: Redux's immutability and action history enable powerful time-travel debugging, helping you track down bugs more effectively.
- Asynchronous Actions: If your app deals with asynchronous operations or side effects, Redux middleware like Redux Thunk or Redux Saga can streamline the process.
- Consistency in Teams: Redux establishes consistent state management patterns within teams, promoting collaboration and maintainability in larger projects.
Example: Setting Up Redux for a Todo List:
import React from 'react';
import { createStore } from 'redux';
import { Provider, useSelector, useDispatch } from 'react-redux';
// Reducer function
const todoReducer = (state = [], action) => {
switch (action.type) {
case 'ADD_TODO':
return [...state, action.payload];
default:
return state;
}
};
const store = createStore(todoReducer);
const App = () => (
<Provider store={store}>
<TodoList />
</Provider>
);
const TodoList = () => {
const todos = useSelector(state => state);
const dispatch = useDispatch();
const addTodo = text => {
dispatch({ type: 'ADD_TODO', payload: { id: Date.now(), text } });
};
return (
<div>
<ul>
{todos.map(todo => (
<li key={todo.id}>{todo.text}</li>
))}
</ul>
<input type="text" onKeyDown={e => e.key === 'Enter' && addTodo(e.target.value)} />
</div>
);
};
Redux Toolkit: Simplifying Redux:
Redux Toolkit is a package that simplifies and streamlines the process of working with Redux. It provides a set of utilities, including predefined actions, reducers, and store configurations, to help you write Redux code more efficiently and with less boilerplate. Redux Toolkit is designed to make common Redux tasks easier while encouraging best practices.
When to Use Redux Toolkit:
- Simplified Setup: Use Redux Toolkit when you want to set up a Redux store quickly and with minimal configuration using the configureStore() function.
- Reduced Boilerplate: If you aim to write Redux code with less boilerplate, Redux Toolkit's createSlice() can help generate reducers, actions, and action creators concisely.
- Async Operations: When dealing with asynchronous operations and managing loading and error states for Redux actions, createAsyncThunk() simplifies the process.
- Immutability Made Easy: Redux Toolkit's integration with Immer makes it a great choice when you want to update state immutably without manually copying state objects.
- Developer Tooling: If you value an improved debugging experience with enhanced DevTools integration, Redux Toolkit offers this benefit.
- Best Practices: Use Redux Toolkit to follow Redux best practices by default, resulting in cleaner, more maintainable code.
- Backward Compatibility: If you have an existing Redux project, Redux Toolkit can be gradually integrated while maintaining compatibility.
Example: Using Redux Toolkit for a Counter:
import React from 'react';
import { createSlice, configureStore, useDispatch, useSelector } from '@reduxjs/toolkit';
const counterSlice = createSlice({
name: 'counter',
initialState: 0,
reducers: {
increment: state => state + 1,
decrement: state => state - 1,
},
});
const store = configureStore({ reducer: counterSlice.reducer });
const Counter = () => {
const count = useSelector(state => state);
const dispatch = useDispatch();
return (
<div>
<p>Count: {count}</p>
<button onClick={() => dispatch(counterSlice.actions.increment())}>Increment</button>
<button onClick={() => dispatch(counterSlice.actions.decrement())}>Decrement</button>
</div>
);
};
MobX: Reactive State Management:
MobX is a state management library for JavaScript applications that focuses on simplicity and reactivity. It provides a way to manage application state that automatically updates components whenever the underlying data changes. MobX uses observable data structures to track changes and ensures that any components using that data are automatically re-rendered when changes occur.
When to Use MobX:
- Reactive Applications: When you want components to automatically update whenever the underlying data changes, MobX's reactivity offers a streamlined way to achieve this.
- Simplicity and Ease of Use: If you're looking for a state management solution that's simple to set up and doesn't require extensive configuration, MobX is a great choice.
- Small to Medium-sized Apps: MobX's simplicity makes it well-suited for smaller to medium-sized applications where setting up complex state management might be overkill.
- Fluid User Interfaces: If your application requires highly interactive and fluid user interfaces that update in real-time based on data changes, MobX's reactivity can help achieve this seamlessly.
- Incremental Adoption: MobX can be adopted incrementally in existing projects, allowing you to use it only in the parts of the application where reactivity is crucial.
- Simple State Logic: When your state logic is relatively straightforward and doesn't require extensive middleware or complex state transitions, MobX can provide an efficient solution.
Example: Implementing MobX in a Shopping Cart:
import React from 'react';
import { makeObservable, observable, action } from 'mobx';
import { observer } from 'mobx-react';
class CartStore {
cart = [];
constructor() {
makeObservable(this, {
cart: observable,
addItem: action,
removeItem: action,
});
}
addItem(item) {
this.cart.push(item);
}
removeItem(id) {
this.cart = this.cart.filter(item => item.id !== id);
}
}
const cartStore = new CartStore();
const ShoppingCart = observer(() => (
<div>
<h2>Shopping Cart</h2>
<ul>
{cartStore.cart.map(item => (
<li key={item.id}>
{item.name}
<button onClick={() => cartStore.removeItem(item.id)}>Remove</button>
</li>
))}
</ul>
</div>
));
Comparison:
1. Complexity:
- useReducer: Moderately complex. Suitable for local state management and simple state transitions.
- Context API: Moderate complexity. Great for simple to medium-sized state sharing without external libraries.
- Redux: More complex. Ideal for large-scale applications and complex state management needs.
- Redux Toolkit: Simplified Redux. Offers an easier entry into Redux with reduced boilerplate.
- MobX: Simple. Focused on reactivity and ease of use.
2. State Sharing:
- useReducer: Primarily for local component state management.
- Context API: Suitable for moderate state sharing between components.
- Redux: Ideal for global state sharing and complex state interactions.
- Redux Toolkit: Same as Redux but with simplified setup and actions.
- MobX: Well-suited for reactive state sharing across components.
3. Boilerplate:
- useReducer: Minimal, but can become verbose for complex state transitions.
- Context API: Moderate. Less verbose than prop drilling but can be tedious for larger apps.
- Redux: High. Requires setting up actions, reducers, and store configuration.
- Redux Toolkit: Reduced. Simplifies actions, reducers, and store setup.
- MobX: Low. Emphasizes minimal setup and concise code.
4. Performance:
- useReducer: Good for local state management and straightforward updates.
- Context API: Good for moderate state sharing, but may not be as optimized as other solutions.
- Redux: Offers performance optimizations for large-scale apps.
- Redux Toolkit: Similar to Redux, with optimizations included.
- MobX: Excellent reactivity for automatically updating components.
5. Learning Curve:
- useReducer: Low to moderate. Familiarity with reducer patterns is beneficial.
- Context API: Low. Straightforward to understand and use.
- Redux: Moderate to high due to its concepts and required boilerplate.
- Redux Toolkit: Moderate. Eases entry into Redux but still requires understanding its principles.
- MobX: Low. Designed for simplicity and ease of use.
6. Ecosystem and Tooling:
- useReducer: Built-in React hook, limited external tooling.
- Context API: Built into React, with some third-party enhancements.
- Redux: Rich ecosystem with DevTools, middleware, and widespread community support.
- Redux Toolkit: Enhanced Redux experience with integrated DevTools and utilities.
- MobX: Robust ecosystem, integrates well with React and has tooling support.
7. Use Cases:
- useReducer: Component-level state transitions and simple logic.
- Context API: Moderate state sharing without extra libraries.
- Redux: Complex global state management, large-scale applications.
- Redux Toolkit: Simplified Redux for all types of applications.
- MobX: Reactive state management, real-time interfaces, simple applications.
8. Adoption in Existing Projects:
- useReducer: Easily integrated into React components, especially functional components.
- Context API: Incremental integration, particularly when migrating from prop drilling.
- Redux: Possible with careful migration, better suited for new projects.
- Redux Toolkit: Suitable for both existing projects and new ones.
- MobX: Incremental integration, especially for adding reactivity.
In conclusion, choosing the right state management solution depends on your project's complexity, performance needs, learning curve, and existing ecosystem. useReducer and Context API are suitable for simpler scenarios, while Redux, Redux Toolkit, and MobX cater to more complex applications with varying levels of ease and performance optimizations.