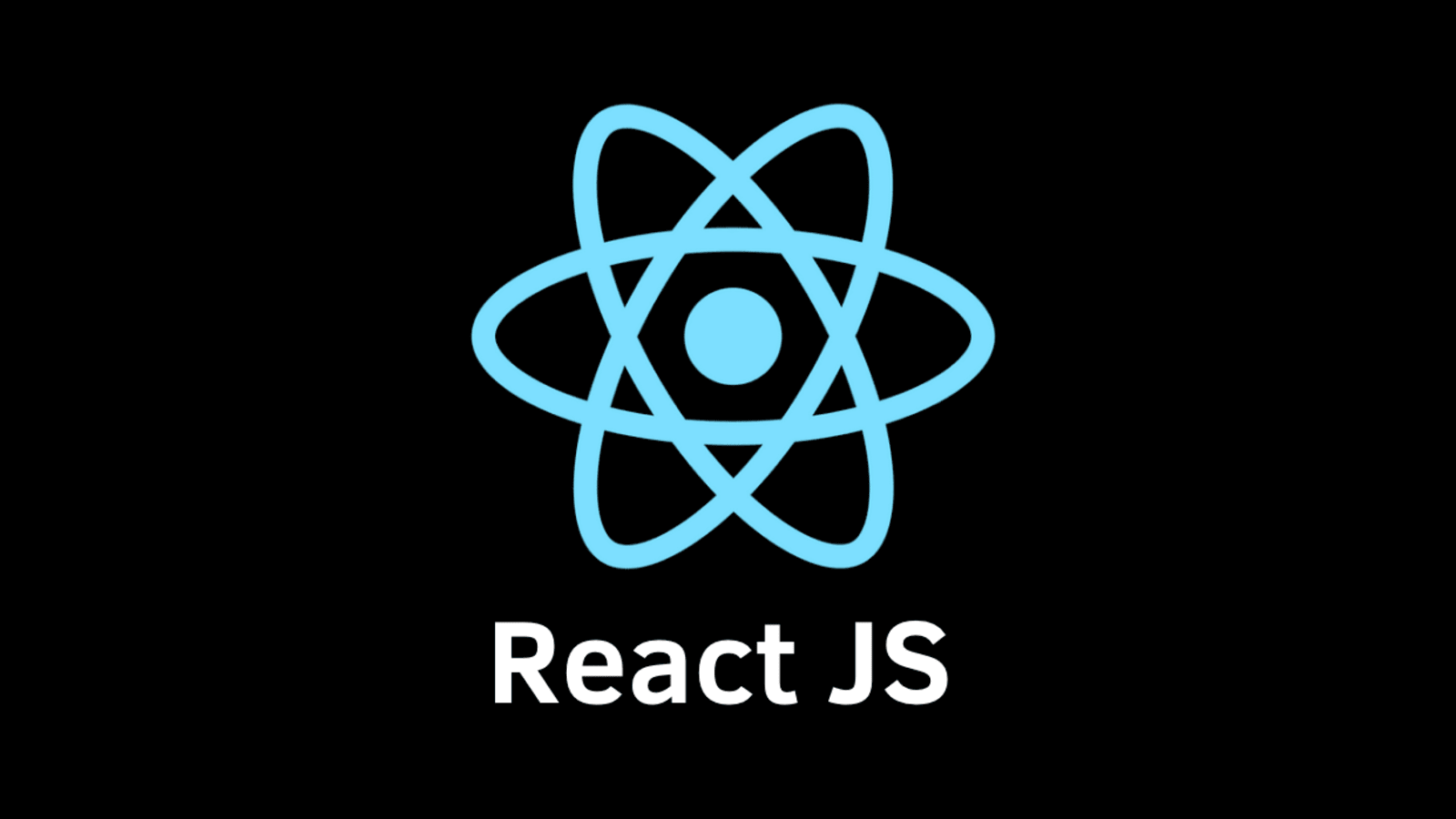
In the world of web development, creating a smooth and seamless user experience is essential. One key aspect of this is navigation between different views or pages within a web application. React, a popular JavaScript library for building user interfaces, offers a powerful solution to this challenge: the React Router library. In this comprehensive tutorial, we will delve into the basics of routing in React using the BrowserRouter and Route components provided by React Router.
What is BrowserRouter?
BrowserRouter is a key component provided by the React Router library that enables declarative routing in your React applications. It essentially helps you manage the navigation and URL changes within a single-page application (SPA) by synchronizing the UI with the URL.
How BrowserRouter Works:
When you wrap your application components with BrowserRouter, it listens to changes in the URL and renders the appropriate components based on the current URL. Unlike traditional multi-page applications where clicking on a link triggers a full page reload, React Router's BrowserRouter ensures that only the necessary components are re-rendered when the URL changes.
What is Route?
In React Router, the Route component is a fundamental building block that enables you to declare how your application's UI should be displayed based on the current URL. It allows you to associate specific UI components with particular URL paths, ensuring that the correct component is rendered when the URL matches a defined path.
Key Concepts of Route:
- Path Matching: The Route component matches the current URL with the path prop you provide. If the URL matches the specified path, the associated component is rendered.
- Component Rendering: When the URL matches the path, the Route component renders the specified component. This component could be a page, a section, or any other UI element you want to display.
- Route Props: The Route component passes a set of props to the rendered component, including information about the route and any URL parameters.
Using `BrowserRouter` and `Route` components from React Router offers several advantages over traditional methods of navigation and routing in web applications. Let's explore the benefits and differences:
Advantages of Using BrowserRouter and Routes:
- Single-Page Application (SPA) Experience: React Router facilitates the creation of SPAs, where navigation between different views happens without full page reloads. This results in a smoother and more responsive user experience.
- Component-Centric Approach: React Router promotes a component-centric architecture, where each route corresponds to a specific UI component. This makes it easier to organize and maintain your application's codebase.
- Declarative Routing: React Router's declarative approach allows you to define routes and their corresponding components in a straightforward manner. You specify what should be rendered based on the URL, and React Router takes care of the rest.
- Dynamic Routing: React Router enables dynamic routing, where URLs can include variables or parameters. This is particularly useful for scenarios where you need to display content based on user input or data from a database.
- URL Parameter Handling: React Router handles URL parameters and passes them as props to the corresponding components. This makes it seamless to access dynamic values from the URL within your components.
- Nested Routes: React Router supports nested routes, allowing you to define routes within routes. This is valuable for creating complex user interfaces with nested views.
- Browser History Manipulation: React Router integrates with the browser's history API, allowing you to programmatically navigate, go back, or go forward without reloading the entire page.
- Code Splitting: React Router can be combined with tools like webpack's code splitting to load only the necessary components when navigating to different routes. This can significantly improve initial page load times.
- Server-Side Rendering (SSR) Support: React Router supports server-side rendering, which can improve search engine optimization (SEO) and initial loading performance.
Differences from Traditional Methods:
- Page Reloads: In traditional multi-page applications, clicking on a link or navigating to a different page typically triggers a full page reload. React Router eliminates the need for such reloads, resulting in a more seamless user experience.
- DOM Manipulation: In traditional applications, navigation often involves manipulating the DOM to replace content. React Router, on the other hand, updates components and their state while keeping the DOM manipulation abstracted.
- State Preservation: React Router helps preserve the state of your components when navigating between different views. In traditional navigation, state preservation can be more complex to manage.
- User Experience: React Router enhances the user experience by providing smoother transitions, quicker navigation, and a more app-like feel, similar to native mobile applications.
- Dynamic Content Loading: React Router allows you to load content dynamically based on the URL, helping to reduce initial page load times compared to loading multiple full pages in traditional navigation.
In summary, using `BrowserRouter` and `Route` components from React Router offers a more efficient, flexible, and user-friendly way to handle navigation and routing in your web applications compared to traditional methods that involve full page reloads and DOM manipulation.
Creating Routes with BrowserRouter:
The BrowserRouter component is a fundamental part of React Router. It provides the routing functionality to your application. To begin routing, you need to wrap your application components with the BrowserRouter.
src/index.js:
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { BrowserRouter } from 'react-router-dom';
ReactDOM.render(
<BrowserRouter>
<App />
</BrowserRouter>,
document.getElementById('root')
);
Here, we've wrapped the App component with the BrowserRouter, making routing available throughout our application.
Creating Routes with Route:
The Route component is used to define individual routes within your application. Each Route component matches a specific path and renders a corresponding component when the path matches the current URL.
Create a new file named Home.js in the src directory:
import React from 'react';
const Home = () => {
return <h1>Welcome to the Home Page!</h1>;
};
export default Home;
Now, let's define a route for the Home component. Open your src/App.js file and modify it as follows:
import React from 'react';
import { BrowserRouter as Router, Route } from 'react-router-dom';
import Home from './Home';
import About from './About';
import Contact from './Contact';
const App = () => {
return (
<Router>
<div>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
<li>
<Link to="/contact">Contact</Link>
</li>
</ul>
</nav>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</div>
</Router>
);
};
export default App;
In this example:
- We import the necessary components from react-router-dom: BrowserRouter, Route, and potentially Link for navigation links.
- Inside the Router, we define navigation links using the Link component.
- We use the Route component to associate URL paths with corresponding components. For example, when the URL is /, the Home component will be rendered. Similarly, when the URL is /about, the About component will be rendered.
- The exact prop ensures that the route is matched only if the path is an exact match. Without this prop, /about would match both the / and /about paths.
In this tutorial, you've learned how to set up basic routing in a React application using the BrowserRouter and Route components provided by React Router. By wrapping your application with the BrowserRouter and defining routes with the Route component, you can create a navigation structure that allows users to seamlessly move between different views. This is just the beginning – React Router offers a wealth of features for creating sophisticated routing systems in your applications. Stay tuned for more advanced routing concepts and techniques!