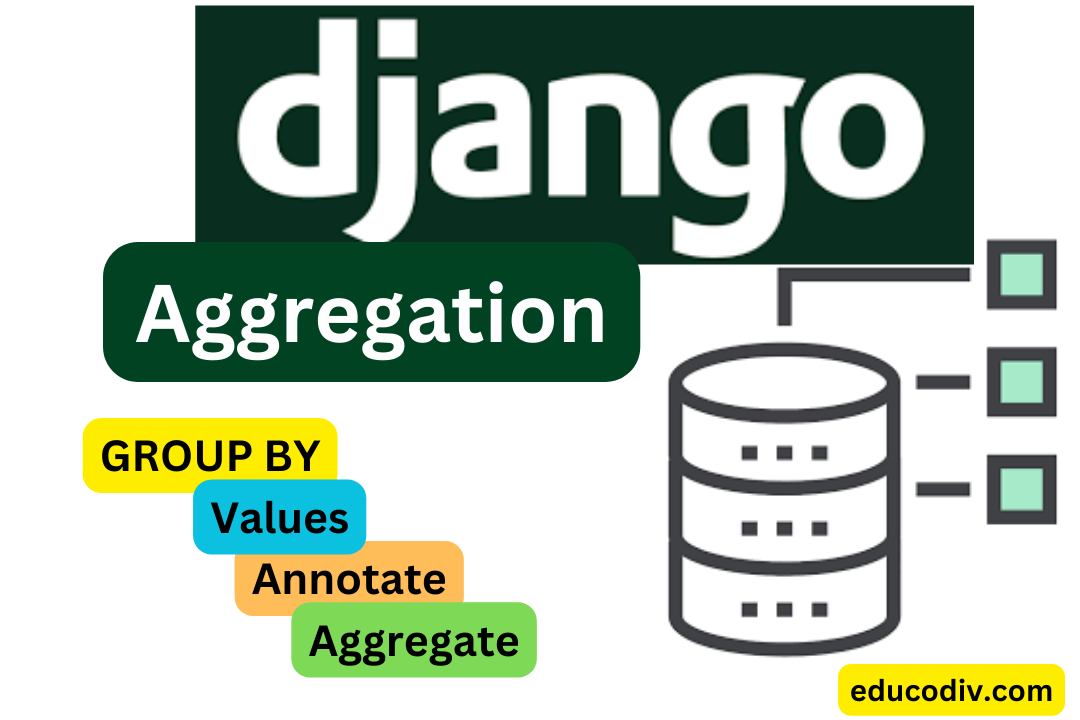
Aggregation refers to the process of summarizing and computing values from multiple rows of data into a single result. This often involves applying mathematical operations such as counting, summing, averaging, or finding the maximum/minimum values across a dataset.
Why do we need the aggregation function:
For instance, imagine a database table storing sales data with individual transactions. Aggregation could be used to calculate the total sales revenue, average transaction amount, or the count of transactions for a specific product or time period. Aggregation is a fundamental concept in database management and is crucial for data analysis, reporting, and extracting meaningful insights from large datasets.
What are aggregate function types:
Database aggregation functions are essential tools for extracting meaningful insights from large datasets by summarizing and computing values. These functions perform mathematical operations on sets of data, consolidating information and providing valuable statistics. Common aggregation functions include:
COUNT(): This function tallies the number of rows that meet a specified condition, offering a simple yet powerful way to determine the size of a dataset.
SUM(): Used for numeric fields, the SUM() function adds up the values in a column, providing a total sum for further analysis, such as calculating total revenue.
AVG(): AVG(), or Average, calculates the arithmetic mean of a set of numeric values. It is valuable for understanding the typical value in a dataset.
MIN() and MAX(): These functions identify the smallest and largest values in a column, respectively, helping to determine the range of data.
Aggregation functions are fundamental to database management, enabling efficient data analysis and reporting across various domains.
Django aggregation functions:
While the fundamental concept of aggregation remains consistent across databases, Django ORM introduces its own set of aggregation functions to facilitate data manipulation and analysis within the framework. Django provides a QuerySet API that includes aggregation functions for interacting with databases. Here's a brief comparison between general database aggregation functions and Django ORM aggregation functions:
COUNT():
- Database Aggregation: Counts the number of rows that meet a specified condition in the entire dataset.
- Django ORM Aggregation: Achieves the same result but is applied to a QuerySet, allowing for more dynamic and conditional counting.
from django.db.models import Count
queryset = MyModel.objects.annotate(num_records=Count('some_field'))
SUM():
- Database Aggregation: Adds up the values in a column across all rows in the dataset.
- Django ORM Aggregation: Performs a similar operation but can be applied selectively to a QuerySet, allowing for aggregation based on specific conditions.
from django.db.models import Sum
total_amount = MyModel.objects.aggregate(total=Sum('amount'))
AVG():
- Database Aggregation: Calculates the average of a numeric column across all rows in the dataset.
- Django ORM Aggregation: Like the database equivalent, computes the average but within the context of a QuerySet, offering more control over the subset of data.
from django.db.models import Avg
average_rating = MyModel.objects.aggregate(avg_rating=Avg('rating'))
MIN() and MAX():
- Database Aggregation: Identifies the smallest and largest values in a column, respectively, across all rows.
- Django ORM Aggregation: Provides the same functionality within a QuerySet, allowing for conditional application.
from django.db.models import Min, Max
min_value = MyModel.objects.aggregate(min_value=Min('some_field'))
max_value = MyModel.objects.aggregate(max_value=Max('some_field'))
annotate() in Django:
- Purpose: The annotate() function is used to add new fields or annotations to each object in a QuerySet based on some aggregate or computed value. It allows you to enhance individual instances with additional information without collapsing the entire QuerySet.
- Example: If you have a model representing books and you want to annotate each book with the average rating of all books, you can use annotate().
- Importance: annotate() is important when you want to extend your model instances with computed or aggregated values on a per-object basis. It maintains the granularity of individual records while providing additional insights derived from the dataset.
from django.db.models import Avg
books_with_avg_rating = Book.objects.annotate(avg_rating=Avg('rating'))
aggregate() in Django:
- Purpose: The aggregate() function is used to perform aggregate calculations on the entire QuerySet, collapsing it into a single result. It returns a dictionary containing the computed values for specified aggregate functions.
- Example: If you want to find the total sales across all orders, you can use aggregate().
- Importance: aggregate() is crucial when you need a single result summarizing the entire dataset. It's useful for obtaining global statistics or totals.
from django.db.models import Sum
total_sales = Order.objects.aggregate(total_sales=Sum('amount'))
In summary, annotate() and aggregate() are essential components of the Django ORM, providing developers with the tools to perform flexible and powerful data aggregations, enriching their applications with valuable insights.
To understand Django aggregation properly, you should have a clear concept of annotate(), aggregate(), and values() functions.
Follow this course to understand Django aggregation.