Using SELECT query we can fetch data from database table. Also we can fetch data conditionally. For example, according to this course,
- Find name and address whose mobile number is 017xxxxxxx.
- Find the names and mobile numbers of people who are over 18 years old.
- Find data who are live in Bangladesh.
- Find data who are foreigner(Do not live in Bangladesh).
Using WHERE clause, we can solve all those questions.
Syntax:
SELECT * FROM table_name WHERE condition;
First see all data of contact table of account database:
SELECT * FROM contact;
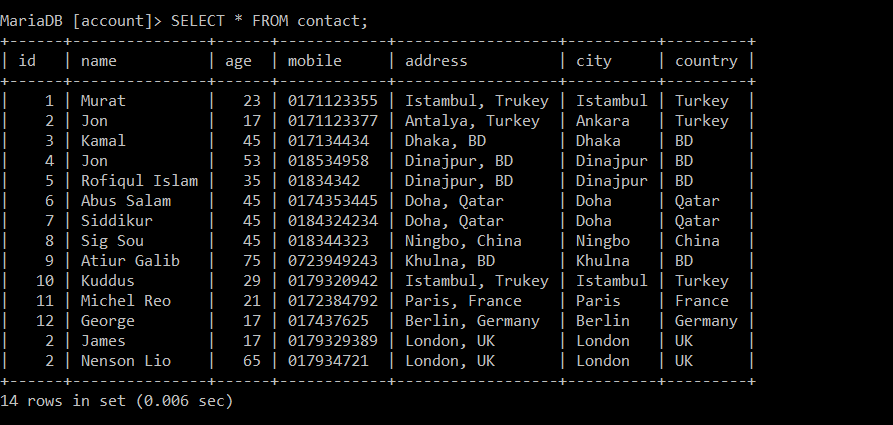
Using SQL find name and address whose mobile number is 0171123377:
SELECT name, address FROM contact WHERE mobile = "0171123377";
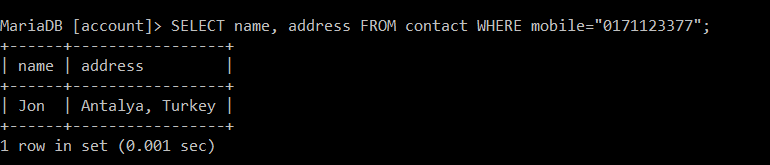
Using SQL find the names and mobile numbers of people who are over 18 years old:
SELECT name, mobile FROM contact WHERE age > 18;
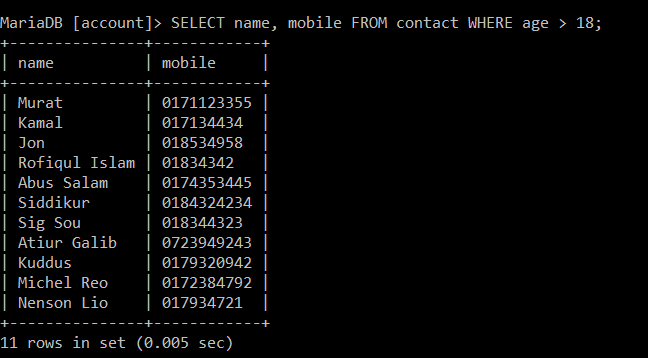
Using SQL find data who are live in BD:
SELECT * FROM contact WHERE country = "BD";
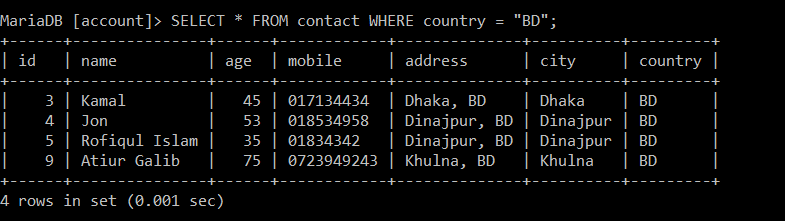
Using SQL find data who are foreigner(Do not live in BD):
SELECT * FROM contact WHERE country != "BD";
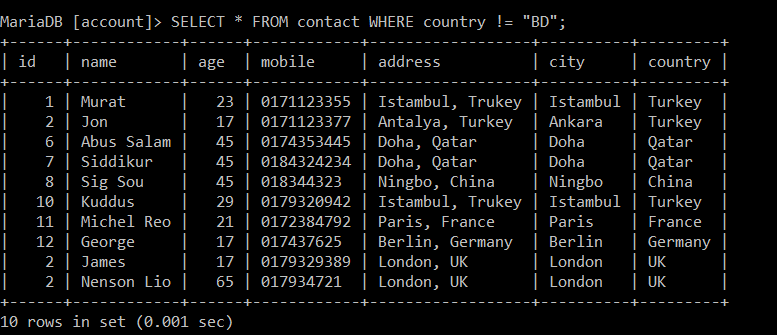
Multiple condition using WHERE clause:
- Find data of people who are over 18 years old and do not live in Bangladesh.
- Find data of people who are over 18 years old or live in Turkey.
Syntax:
SELECT * FROM table_name WHERE 1st_condition AND 2nd_condition AND 3rd condition ...... ;
OR
SELECT * FROM table_name WHERE 1st_condition OR 2nd_condition OR 3rd condition ...... ;
OR
SELECT * FROM table_name WHERE 1st_condition OR 2nd_condition AND 3rd condition ...... ;
OR
SELECT * FROM table_name WHERE 1st_condition AND 2nd_condition OR 3rd condition ...... ;
Using SQL find data of people who are over 18 years old and do not live in Bangladesh:
SELECT * FROM contact WHERE age > 18 AND country != "BD";
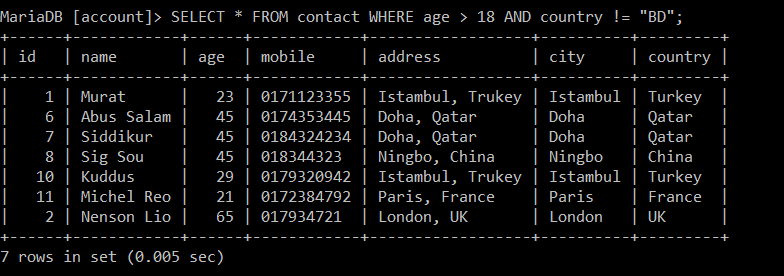
Using SQL find data of people who are over 18 years old or live in Turkey:
SELECT * FROM contact WHERE age > 18 OR country = "Turkey";
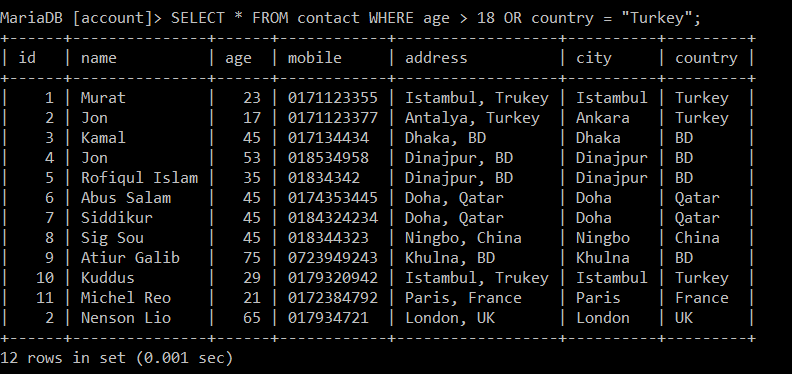