In tree data structures, there are three commonly used types of traversals: pre-order, in-order, and post-order. These traversal techniques define the order in which the nodes of a tree are visited. A tree can be traversed in the following ways:
- Pre-order Traversal
- In-order Traversal
- Post-order Traversal
Pre-order Traversal in Trees:
Pre-order traversal is a way to visit all the nodes of a tree in a specific order. Traversing a binary tree in pre-order means visiting the nodes in the following order:
- Visit the root node.
- Traverse the left subtree.
- Traverse the right subtree.
Pre-order traversal visits the current node before its children. It is useful for creating a copy of the tree, evaluating an expression, or implementing prefix notation.
Here's an example of pre-order traversal of a tree:
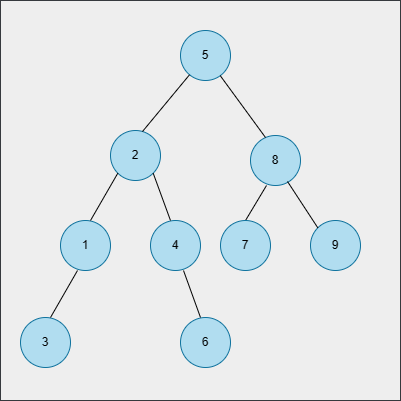
Pre-order traversal of this tree would result in the following sequence of numbers: 5, 2, 1, 3, 4, 8, 9, 7.
Here, we start by visiting the root node, which is 5. Then we traverse the left subtree (2, 1, 3) and the right subtree (4). The traversal continues recursively until all the nodes are visited.
C++ implementation for pre-order traversal:
#include <iostream>
using namespace std;
struct TreeNode {
int data;
TreeNode* left;
TreeNode* right;
};
TreeNode* createNode(int data) {
TreeNode* newNode = new TreeNode;
newNode->data = data;
newNode->left = nullptr;
newNode->right = nullptr;
return newNode;
}
void preOrderTraversal(TreeNode* root) {
if (root != nullptr) {
cout << root->data << " ";
preOrderTraversal(root->left);
preOrderTraversal(root->right);
}
}
int main() {
// Create the tree
TreeNode* root = createNode(5);
root->left = createNode(2);
root->right = createNode(8);
root->left->left = createNode(1);
root->left->right = createNode(4);
root->right->right = createNode(9);
root->left->left->left = createNode(3);
root->right->right->left = createNode(7);
// Perform pre-order traversal
cout << "Pre-order traversal: ";
preOrderTraversal(root);
cout << endl;
return 0;
}
Output:
Pre-order traversal: 5 2 1 3 4 8 9 7
In the above code, the 'createNode' function is defined to create a new node with the given data. It allocates memory for the new node, assigns the data, and initializes the left and right pointers to `nullptr`. The function then returns the pointer to the newly created node.
The 'preOrderTraversal' function is defined to perform the pre-order traversal of the binary tree. It takes a pointer to the root of the tree as a parameter. If the current node is not 'nullptr', the function first prints the data of the current node, and then recursively calls itself on the left subtree and the right subtree.
In the 'main' function, the binary tree is created by using the 'createNode' function. Nodes are created one by
one and connected to their parent nodes using the left and right pointers. The tree structure in the example is recreated.
After creating the tree, the 'preOrderTraversal' function is called with the root of the tree as the argument. This will traverse the tree in pre-order fashion and print the node values.