Breadth-First Search (BFS) is a graph traversal algorithm that explores all the vertices of a graph level by level.
It starts from a chosen source vertex and visits all its neighbors before moving to their neighbors, and so on. BFS is commonly implemented using a queue data structure.
BFS Algorithm
- Create a queue and enqueue the source vertex.
- Create a boolean array or hash set to keep track of visited vertices and mark the source vertex as visited.
- While the queue is not empty, do the following: a. Dequeue a vertex from the queue and process it (print or store). b. Enqueue all unvisited neighbors of the dequeued vertex and mark them as visited.
BFS Traversal
- First we start with an empty queue and mark all nodes as unvisited.
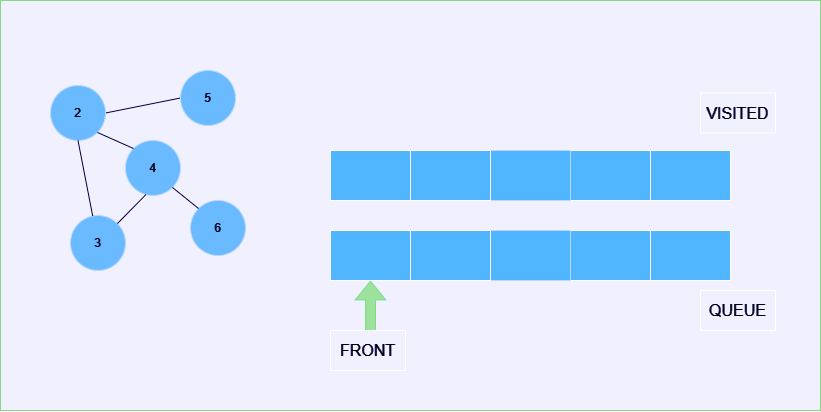
2. Choose a starting node, say node 2, and enqueue it. Mark node 2 as visited. We put 3,4,5 int the QUEUE as these are the adjacent nodes of 2. We mark 2 as visited and put it in the VISITED queue.
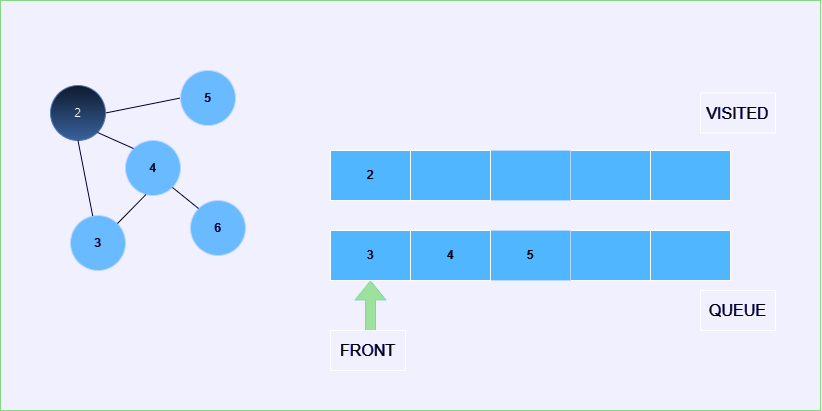
3. We repeat the process with the Front item of the QUEUE and start scanning. We put 4 and 5 in the QUEUE as these are the adjacent nodes of 3. We mark 3 as VISITED. We pop 3 from the QUEUE.
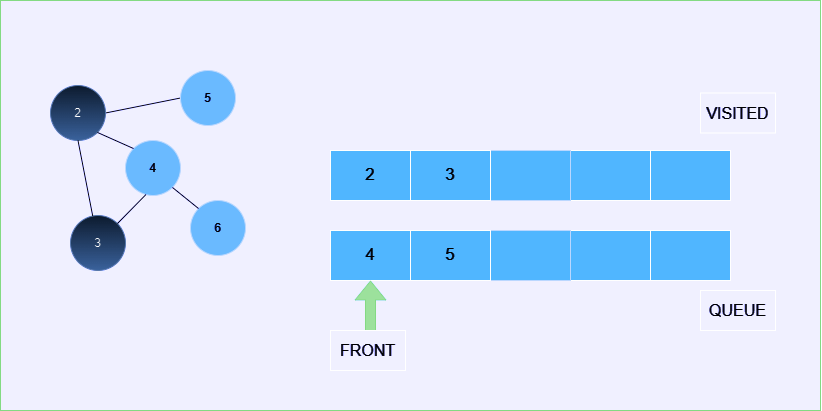
4. Next up, we take the front of the QUEUE again and repeat the above process. So there is 5 and 6 as the adjacent nodes of 4, we put them in the QUEUE, mark 4 as VISITED and pop 4.
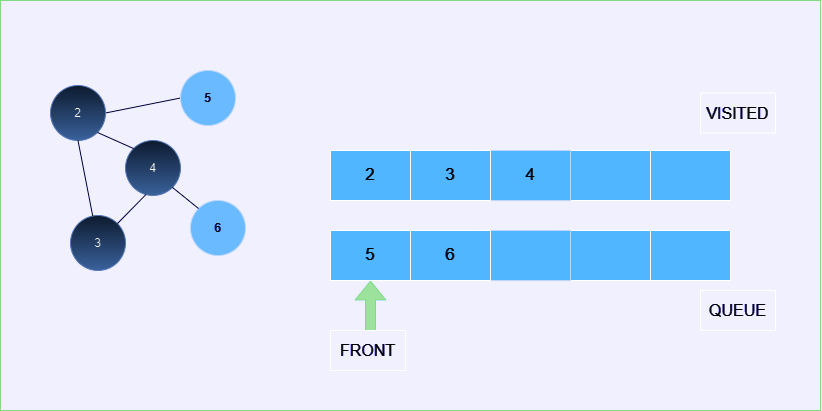
5. We repeat the above process for the rest of the items in the QUEUE which are 5 and 6.
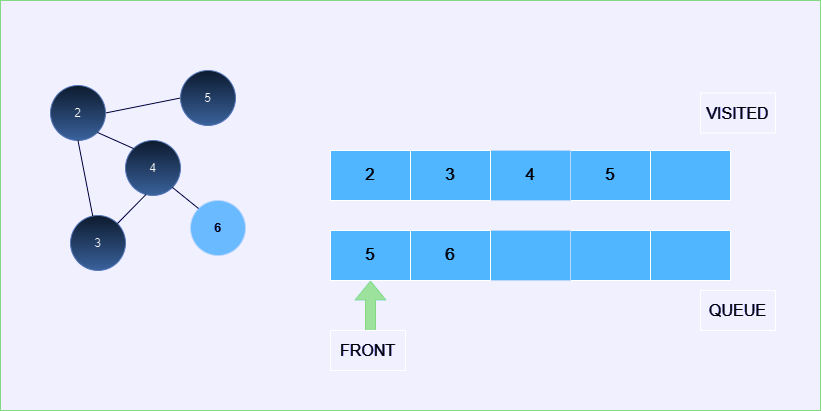
All elements have been visited and the final output is 2,3,4,5,6.
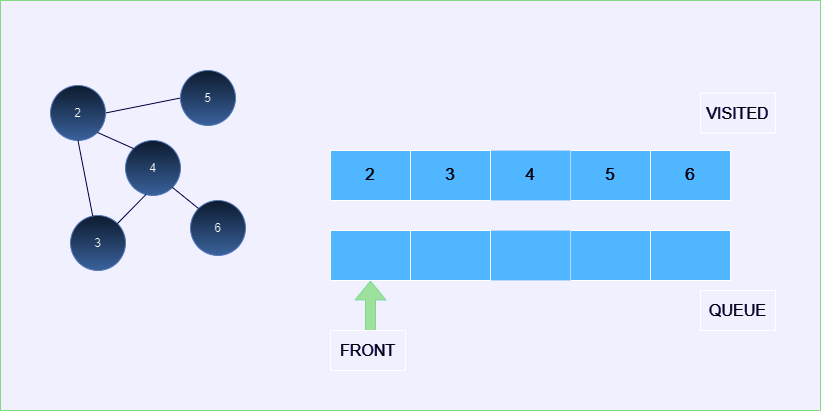
C++ implementation of BFS
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
// Graph class with BFS traversal method
class Graph {
int V; // Number of vertices
vector<vector<int>> adj; // Adjacency list
public:
Graph(int v) {
V = v;
adj.resize(V);
}
void addEdge(int u, int v) {
adj[u].push_back(v);
adj[v].push_back(u); // For undirected graph
}
void BFS(int source) {
vector<bool> visited(V, false);
queue<int> q;
q.push(source);
visited[source] = true;
while (!q.empty()) {
int vertex = q.front();
q.pop();
cout << vertex << " "; // Process the vertex (print in this case)
for (int neighbor : adj[vertex]) {
if (!visited[neighbor]) {
q.push(neighbor);
visited[neighbor] = true;
}
}
}
}
};
int main() {
Graph g(7);
g.addEdge(2, 3);
g.addEdge(2, 4);
g.addEdge(2, 5);
g.addEdge(3, 4);
g.addEdge(4, 6);
g.addEdge(6, 4);
cout << "BFS traversal starting from vertex 2: ";
g.BFS(2);
return 0;
}
Output:
BFS traversal starting from vertex 2: 2 3 4 5 6