Arrays are a fundamental concept in programming, providing an efficient way to store and organize data. In this article, we will explore the definition, syntax, and declaration of arrays in C++. This article provides a comprehensive guide on accessing, manipulating, sorting, and searching array elements, along with exploring the practical applications of multi-dimensional arrays in solving complex problems and many more.
What is Array?
An array is a data structure that stores a collection of elements of the same data types stored in contiguous memory locations. Elements within an array can be accessed using an index, which represents the position of each element within the array. Some array operations involve accessing elements, inserting and deleting elements, sorting, searching etc.
Topics covered in this article:
- C++ array initialization
- Accessing array elements
- Traversing array elements
- Updating array elements
- Deleting array elements
- Types of arrays
- Advantages and disadvantages of arrays in C++
C++ array initialization
Initializing an array refers to the process of assigning values to the elements of an array at the time of declaration or later in the program. Array initialization ensures that the array has predefined values. When the array is declared it contains garbage values initially. The initialization can be done using individual values, an initializer list, default values, string literals, or dynamic input from the user.
The syntax for initialization an array is:
datatype arrayName[size] = {value1, value2, value3, ...};
Arrays can be initialized in different ways.
1.Initializing array with Individual Values:
Individual values can be assigned to array elements one by one using the assignment operator (=). The size of the array is defined beforehand. For example:
int numbers[3];
numbers[0] = 1;
numbers[1] = 2;
numbers[2] = 3;
However, this is not an effective way of initializing an array.
2.Initializing array with an Initializer List:
In this case, the array "numbers" is declared without specifying a size. The compiler automatically determines the size based on the number of values in the initializer list, which is three in this example. The values 1, 2, and 3 are assigned to the elements of the array in the order they appear in the initializer list. Notice the values are enclosed in braces { } and separated by commas. For example:
int numbers[] = {1, 2, 3};
3. Initializing array with a Partial List:
Initializing an array with a partial list provides values for only a subset of elements during initialization, while the remaining elements are initialized with default values. For example:
int numbers[5] = {1, 2, 3};
In this case, the array "numbers" has a size of 5, but only the first three elements receive explicit values of 1, 2, and 3, respectively. The remaining elements, index 3 and 4, will be initialized with default values, which for integers is 0.
4.Initializing array with a String Literal:
To initialize a character array with a string literal, you simply assign the string literal directly to the array variable. The compiler automatically allocates memory for the array based on the length of the string and assigns each character of the string to consecutive elements of the array. For example:
char greeting[] = "Hello";
In this case, the character array "greeting" is declared and initialized with the string literal "Hello". The compiler determines the size of the array based on the length of the string. An additional space for a null character ('\0') is automatically added at the end of the array to mark the end of the string.

Accessing array elements:
Array elements are accessed by using their respective index positions, which typically start from 0 and increment by 1 for each subsequent element. To access a specific element of an array, it is needed to provide the array name followed by the index of the element within square brackets [].
arrayName[index];
The initial element of an array is always at index 0, and the last member is always at index N - 1, where N is the total number of elements in the array.
Here's an example in C++ demonstrating how to access array elements:
#include<bits/stdc++.h>
using namespace std;
int main() {
// Initialize an array of integers
int numbers[] = {10, 20, 30, 40, 50};
// Access and print individual elements of the array
std::cout << "Element at index 0: " << numbers[0] << endl;
std::cout << "Element at index 1: " << numbers[1] << endl;
std::cout << "Element at index 2: " << numbers[2] << endl;
std::cout << "Element at index 3: " << numbers[3] << endl;
std::cout << "Element at index 4: " << numbers[4] << endl;
return 0;
}
Output:
Traversing array elements:
Array traversal is a fundamental operation in computer programming that involves systematically accessing and processing each element within an array. To visit each element of the array loops, such as for or while are applied.
for (int i = 0; i < N; i++) {
arrayName[i];
}
Updating array elements:
Updating array elements refers to modifying the values of specific elements within an array. Once an array is declared and initialized, individual elements can be updated with new values to reflect changes in the program or to store different data.
To update an array element, it is needed to specify the index of the element to be modified and assign a new value to it using the assignment operator (=).
arrayName[i] = new_value;
Here's an example in C++ demonstrating how to update array elements:
#include<bits/stdc++.h>
using namespace std;
int main() {
// Declare and initialize an array of integers
int numbers[] = {10, 20, 30, 40, 50};
// Print the original array
cout << "Original array: ";
for (int i = 0; i < 5; ++i) {
cout << numbers[i] << " ";
}
cout << endl;
// Update the element at index 2 with a new value
numbers[2] = 35;
// Print the updated array
cout << "Updated array: ";
for (int i = 0; i < 5; ++i) {
cout << numbers[i] << " ";
}
cout << std::endl;
return 0;
}
Output:
Original array: 10 20 30 40 50
Updated array: 10 20 35 40 50
Deleting array elements:
Elements in an array can either be deleted by index or by value.
Here's an example in C++ demonstrating how to delete array elements by index and by value:
#include <iostream>
using namespace std;
int main() {
// Sample array
int myArray[] = {10, 20, 30, 40, 50};
int size = sizeof(myArray) / sizeof(myArray[0]);
cout<<"original array: ";
// Printing the updated array
for (int i = 0; i < size; i++) {
cout << myArray[i] << " ";
}
cout <<endl;
// Deleting an element at a specific index
int indexToDelete = 2;
for (int i = indexToDelete; i < size - 1; i++) {
myArray[i] = myArray[i + 1];
}
size--;
cout<<"array after deleting by index: ";
// Printing the updated array
for (int i = 0; i < size; i++) {
cout << myArray[i] << " ";
}
cout <<endl;
// Deleting a specific element by value
int valueToDelete = 40;
int foundIndex = -1;
for (int i = 0; i < size; i++) {
if (myArray[i] == valueToDelete) {
foundIndex = i;
break;
}
}
if (foundIndex != -1) {
for (int i = foundIndex; i < size - 1; i++) {
myArray[i] = myArray[i + 1];
}
size--;
}
cout<<"array after deleting by value: ";
// Printing the updated array
for (int i = 0; i < size; i++) {
cout << myArray[i] << " ";
}
cout << endl;
return 0;
}
Types of arrays:
Based on dimension there are two types of arrays.
1.One Dimensional Array:
The simplest and most typical sort of arrays are one-dimensional arrays, usually referred to as flat arrays. Each element is accessible using an index, and they are stored in a linear order. Data of a single kind, such as integers, characters, or floating-point values, can be stored in one-dimensional arrays.
arrayName [size];
Here's an example in C++ demonstrating a One Dimensional Array :
#include <iostream>
using namespace std;
int main() {
// Input array size
int size;
cout << "Enter the size of the array: ";
cin >> size;
// Create the array
int myArray[size];
// Input array elements
cout << "Enter the elements of the array:\n";
for (int i = 0; i < size; i++) {
cout << "Element " << i << ": ";
cin >> myArray[i];
}
// Traverse and print array elements
cout << "Array elements:\n";
for (int i = 0; i < size; i++) {
cout << myArray[i] << " ";
}
cout << endl;
return 0;
}
Output:
Enter the size of the array: 3
Enter the elements of the array:
Element 0: 3
Element 1: 2
Element 2: 4
Array elements:
3 2 4
2.Multi Dimensional Array
Multi-dimensional arrays offer a tabular or grid-like layout, allowing for more complicated data organization, as opposed to expressing data in a linear succession.
The two-dimensional array, which represents data in a grid-like manner with rows and columns, is the most prevalent sort of multi-dimensional array. However, multi-dimensional arrays, such three-dimensional or higher-dimensional arrays, can have more than two dimensions.
When displaying graphics, gaming boards, spreadsheet-like data, or scientific data sets, multi-dimensional arrays are very helpful since they can handle situations where structured data or matrices are present.
Two Dimensional Array:
syntax:
arrayName[size1] [size2];
Here, size1 refers to number of rows
size2 refers to number of columns
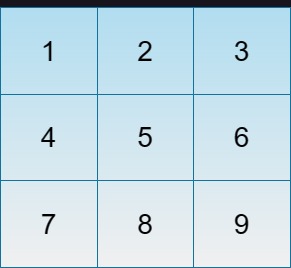
Here's an example in C++ demonstrating a Two Dimensional Array:
#include <iostream>
using namespace std;
int main() {
// Create a 2D array (3x3 matrix)
int matrix[3][3] = {{1, 2, 3},
{4, 5, 6},
{7, 8, 9}};
// Traverse and print the matrix elements
cout << "Matrix elements:\n";
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cout << matrix[i][j] << " ";
}
cout << endl;
}
return 0;
}
Output:
Matrix elements:
1 2 3
4 5 6
7 8 9
Three Dimensional Array:
syntax:
arrayName [size1] [size2] [size3];
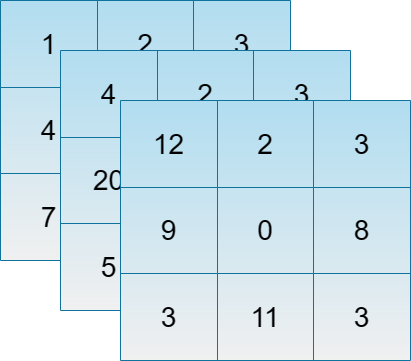
Here's an example in C++ demonstrating a Three Dimensional Array:
// C Program to illustrate the 3d array
#include <iostream>
using namespace std;
int main() {
// 3D array initialization
int arr[3][3][3] = { {{10, 20,40}, {30, 40,0}}, {{50, 60,10,9}, {0, 0}} };
// Traverse and print the matrix elements
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 2; j++) {
for (int k = 0; k < 2; k++) {
cout << arr[i][j][k] << " ";
}
cout << endl;
}
cout << endl << endl;
}
return 0;
}
Advantages and disadvantages of arrays in C++:
- Arrays that provide effective sequential element access. As a result, index-based access to elements is quicker than using other data structures like linked lists.
- Fast retrieval or alteration of elements at certain points within the array are made possible by direct access to any element in arrays using its index.
- Arrays require a fixed amount of memory because their size is predetermined, which results in efficient memory usage.
- Arrays provide a straightforward and succinct syntax for declaring, initializing, and accessing their elements.
Disadvantages and disadvantages of arrays in C++:
- In C++, an array's size is predetermined at declaration time. It is difficult to dynamically resize. It is necessary to establish a new array with a larger size in order to fit additional elements.
- Arrays use memory regardless of how many elements are contained in them. Memory could be wasted if the array size is significantly larger than the number of elements it can hold.
- C++ arrays do not automatically check their boundaries. Accessing or changing items outside of their defined boundaries may result in unpredictable behavior or memory corruption.
- Flexibility is limited because it's difficult to add or remove elements from an array. It is not possible to add or remove elements from an array in the middle.