Inorder traversal is a tree traversal algorithm that visits the nodes of a binary tree in an in-order fashion. This means that
- the left subtree of a node is visited
- then the root is visited
- fianally the right subtree of a node is visited
Inorder traversal can be used to perform a variety of operations on binary trees, such as:
- Finding the smallest or largest element in the tree
- Sorting the elements in the tree
- Deleting a node from the tree
Let's understand inorder traversal using an example-
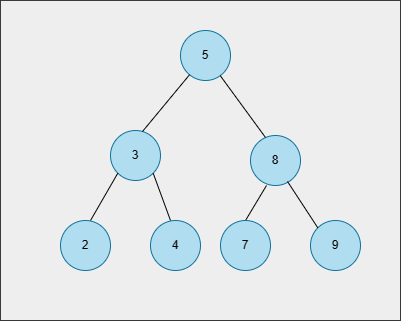
after inorder traversal the output is: 2, 3, 4, 5, 7, 8, 9
Step-by-step process for inorder traversal:
1. Start at the root node (5).
2. Traverse the left subtree by recursively applying the inorder traversal to the left child (3).
- Move to the left child node (3).
3. Repeat step 2 until reaching a node with no left child (2).
- Move to the left child node (2).
- As there is no left child, output the value of the current node (2).
- Move to the right child node (3).
4. Output the value of the current node (3).
5. Traverse the right subtree by recursively applying the inorder traversal to the right child (4).
- Move to the left child node (4).
- As there is no left child, output the value of the current node (4).
- Move to the right child node (3).
6. Output the value of the current node (5).
7. Traverse the right subtree by recursively applying the inorder traversal to the right child (8).
- Move to the left child node (7).
8. Repeat steps 3 to 6 for the remaining nodes in the tree (7, 8, 9).
- Move to the left child node (7).
- As there is no left child, output the value of the current node (7).
- Move to the right child node (8).
9. Output the value of the current node (8).
10. Traverse the left subtree by recursively applying the inorder traversal to the left child (9).
- Move to the left child node (9).
- As there is no left child, output the value of the current node (9).
- Move to the right child node (8).
11. Output the value of the current node (9).
The inorder traversal of the given tree would produce the following sequence: 2, 3, 4, 5, 7, 8, 9.
Complexity Analysis:
Time Complexity: The time complexity of inorder traversal for a binary tree with n nodes is O(n). This is because, in the worst case, the algorithm needs to visit and process each node exactly once. Since each node is visited once, the time complexity grows linearly with the number of nodes.
Space Complexity: The space complexity of the inorder traversal algorithm depends on the implementation approach like recursive approach and iterative approach.
- Recursive Approach: In the recursive approach, the space complexity is determined by the maximum depth of the tree, which is the height of the tree. In the worst case scenario, where the binary tree is skewed and resembles a linked list, the height of the tree is O(n), resulting in a space complexity of O(n). This is because the recursion call stack will grow to accommodate the recursive calls for each node.
- Iterative Approach: In the iterative approach, the space complexity is determined by the maximum number of nodes that can be present on the stack at any given time. In the case of inorder traversal, the maximum number of nodes on the stack is equal to the maximum number of left children encountered during traversal. In the worst case, where the binary tree is skewed, the number of left children can be n-1, resulting in a space complexity of O(n). However, in a balanced binary tree, the number of left children is limited, resulting in a space complexity of O(log n).
Approach | Time Complexity | Space Complexity |
---|---|---|
Recursive | O(n) | O(n) |
Iterative | O(n) | O(log n) or O(n) |