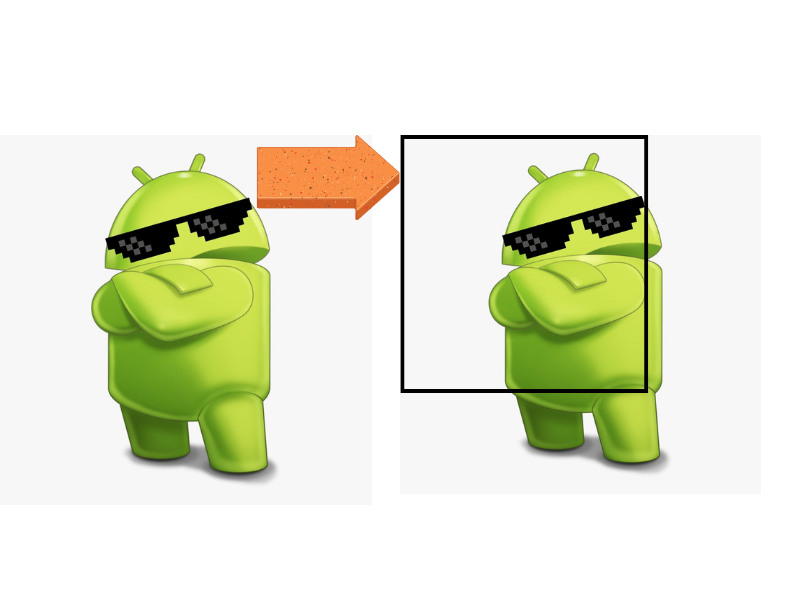
Using python opencv, easily possible to crop image. Four parameters are necessary for cropping image.
- start index or pixel along x axis (start_x_axis)
- width or cropping value along x axis (cropping width)
- start index or pixel along y axis (start_y_axis)
- height or cropping value along y axis (cropping height)
Code:
First install python opencv using pip install opencv-python
# importing opencv
import cv2
# cropping width
c_width = 500
#cropping height
c_height = 600
# read image
# input = cv2.imread(path)
input = cv2.imread("image.jpeg")
# real width of image
w = input.shape[1]
# real height of image
h = input.shape[0]
# your required value, input here what you want
start_x = 0
# your required value, input here what you want
start_y = 0
# crop image
crop = input[start_y : start_y + c_height, start_x : start_x + c_width]
# save image
# cv2.imwrite(output_path, crop)
cv2.imwrite("output.png", crop)
Input:
image.jpeg ( in same folder in python file)
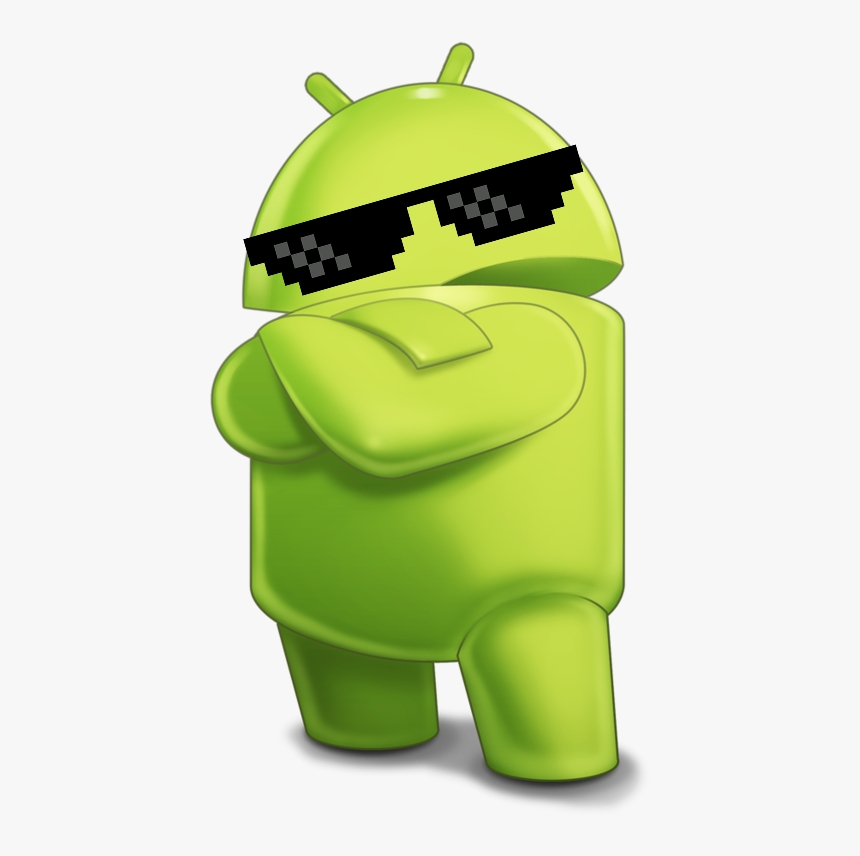
Output:
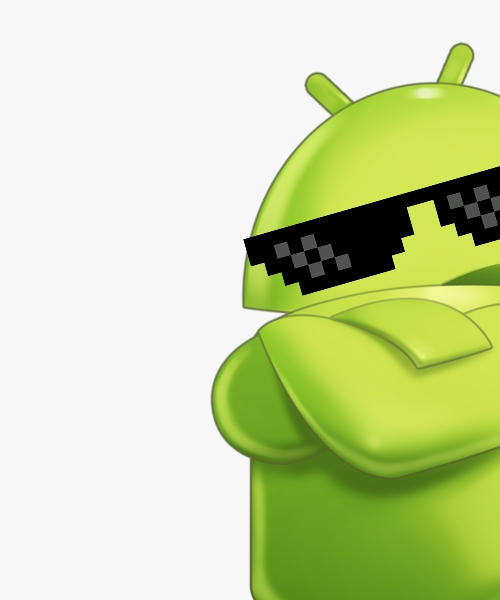